mirror of
https://github.com/ehw-fit/ariths-gen.git
synced 2025-04-19 13:30:56 +01:00
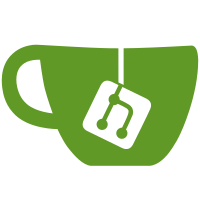
* #10 CGP Circuits as inputs (#11) * CGP Circuits as inputs * #10 support of signed output in general circuit * input as output works * output connected to input (c) * automated verilog testing * output rename * Implemented CSA and Wallace tree multiplier composing of CSAs. Also did some code cleanup. * Typos fix and code cleanup. * Added new (approximate) multiplier architectures and did some minor changes regarding sign extension for c output formats. * Updated automated testing scripts. * Small bugfix in python code generation (I initially thought this line is useless). * Updated generated circuits folder. Co-authored-by: Vojta Mrazek <mrazek@fit.vutbr.cz>
1586 lines
132 KiB
C
1586 lines
132 KiB
C
#include <stdio.h>
|
|
#include <stdint.h>
|
|
|
|
uint8_t xor_gate(uint8_t a, uint8_t b){
|
|
return ((a >> 0) & 0x01) ^ ((b >> 0) & 0x01);
|
|
}
|
|
|
|
uint8_t not_gate(uint8_t a){
|
|
return ~(((a >> 0) & 0x01)) & 0x01;
|
|
}
|
|
|
|
uint8_t and_gate(uint8_t a, uint8_t b){
|
|
return ((a >> 0) & 0x01) & ((b >> 0) & 0x01);
|
|
}
|
|
|
|
uint8_t or_gate(uint8_t a, uint8_t b){
|
|
return ((a >> 0) & 0x01) | ((b >> 0) & 0x01);
|
|
}
|
|
|
|
uint8_t fs(uint8_t a, uint8_t b, uint8_t bin){
|
|
uint8_t fs_out = 0;
|
|
uint8_t fs_xor0 = 0;
|
|
uint8_t fs_not0 = 0;
|
|
uint8_t fs_and0 = 0;
|
|
uint8_t fs_xor1 = 0;
|
|
uint8_t fs_not1 = 0;
|
|
uint8_t fs_and1 = 0;
|
|
uint8_t fs_or0 = 0;
|
|
|
|
fs_xor0 = xor_gate(((a >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
fs_not0 = not_gate(((a >> 0) & 0x01));
|
|
fs_and0 = and_gate(((fs_not0 >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
fs_xor1 = xor_gate(((bin >> 0) & 0x01), ((fs_xor0 >> 0) & 0x01));
|
|
fs_not1 = not_gate(((fs_xor0 >> 0) & 0x01));
|
|
fs_and1 = and_gate(((fs_not1 >> 0) & 0x01), ((bin >> 0) & 0x01));
|
|
fs_or0 = or_gate(((fs_and1 >> 0) & 0x01), ((fs_and0 >> 0) & 0x01));
|
|
|
|
fs_out |= ((fs_xor1 >> 0) & 0x01ull) << 0;
|
|
fs_out |= ((fs_or0 >> 0) & 0x01ull) << 1;
|
|
return fs_out;
|
|
}
|
|
|
|
uint8_t mux2to1(uint8_t d0, uint8_t d1, uint8_t sel){
|
|
uint8_t mux2to1_out = 0;
|
|
uint8_t mux2to1_and0 = 0;
|
|
uint8_t mux2to1_not0 = 0;
|
|
uint8_t mux2to1_and1 = 0;
|
|
uint8_t mux2to1_xor0 = 0;
|
|
|
|
mux2to1_and0 = and_gate(((d1 >> 0) & 0x01), ((sel >> 0) & 0x01));
|
|
mux2to1_not0 = not_gate(((sel >> 0) & 0x01));
|
|
mux2to1_and1 = and_gate(((d0 >> 0) & 0x01), ((mux2to1_not0 >> 0) & 0x01));
|
|
mux2to1_xor0 = xor_gate(((mux2to1_and0 >> 0) & 0x01), ((mux2to1_and1 >> 0) & 0x01));
|
|
|
|
mux2to1_out |= ((mux2to1_xor0 >> 0) & 0x01ull) << 0;
|
|
return mux2to1_out;
|
|
}
|
|
|
|
uint64_t arrdiv16(uint64_t a, uint64_t b){
|
|
uint64_t arrdiv16_out = 0;
|
|
uint8_t arrdiv16_fs0_xor0 = 0;
|
|
uint8_t arrdiv16_fs0_and0 = 0;
|
|
uint8_t arrdiv16_fs1_xor1 = 0;
|
|
uint8_t arrdiv16_fs1_or0 = 0;
|
|
uint8_t arrdiv16_fs2_xor1 = 0;
|
|
uint8_t arrdiv16_fs2_or0 = 0;
|
|
uint8_t arrdiv16_fs3_xor1 = 0;
|
|
uint8_t arrdiv16_fs3_or0 = 0;
|
|
uint8_t arrdiv16_fs4_xor1 = 0;
|
|
uint8_t arrdiv16_fs4_or0 = 0;
|
|
uint8_t arrdiv16_fs5_xor1 = 0;
|
|
uint8_t arrdiv16_fs5_or0 = 0;
|
|
uint8_t arrdiv16_fs6_xor1 = 0;
|
|
uint8_t arrdiv16_fs6_or0 = 0;
|
|
uint8_t arrdiv16_fs7_xor1 = 0;
|
|
uint8_t arrdiv16_fs7_or0 = 0;
|
|
uint8_t arrdiv16_fs8_xor1 = 0;
|
|
uint8_t arrdiv16_fs8_or0 = 0;
|
|
uint8_t arrdiv16_fs9_xor1 = 0;
|
|
uint8_t arrdiv16_fs9_or0 = 0;
|
|
uint8_t arrdiv16_fs10_xor1 = 0;
|
|
uint8_t arrdiv16_fs10_or0 = 0;
|
|
uint8_t arrdiv16_fs11_xor1 = 0;
|
|
uint8_t arrdiv16_fs11_or0 = 0;
|
|
uint8_t arrdiv16_fs12_xor1 = 0;
|
|
uint8_t arrdiv16_fs12_or0 = 0;
|
|
uint8_t arrdiv16_fs13_xor1 = 0;
|
|
uint8_t arrdiv16_fs13_or0 = 0;
|
|
uint8_t arrdiv16_fs14_xor1 = 0;
|
|
uint8_t arrdiv16_fs14_or0 = 0;
|
|
uint8_t arrdiv16_fs15_xor1 = 0;
|
|
uint8_t arrdiv16_fs15_or0 = 0;
|
|
uint8_t arrdiv16_mux2to10_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to11_and1 = 0;
|
|
uint8_t arrdiv16_mux2to12_and1 = 0;
|
|
uint8_t arrdiv16_mux2to13_and1 = 0;
|
|
uint8_t arrdiv16_mux2to14_and1 = 0;
|
|
uint8_t arrdiv16_mux2to15_and1 = 0;
|
|
uint8_t arrdiv16_mux2to16_and1 = 0;
|
|
uint8_t arrdiv16_mux2to17_and1 = 0;
|
|
uint8_t arrdiv16_mux2to18_and1 = 0;
|
|
uint8_t arrdiv16_mux2to19_and1 = 0;
|
|
uint8_t arrdiv16_mux2to110_and1 = 0;
|
|
uint8_t arrdiv16_mux2to111_and1 = 0;
|
|
uint8_t arrdiv16_mux2to112_and1 = 0;
|
|
uint8_t arrdiv16_mux2to113_and1 = 0;
|
|
uint8_t arrdiv16_mux2to114_and1 = 0;
|
|
uint8_t arrdiv16_not0 = 0;
|
|
uint8_t arrdiv16_fs16_xor0 = 0;
|
|
uint8_t arrdiv16_fs16_and0 = 0;
|
|
uint8_t arrdiv16_fs17_xor1 = 0;
|
|
uint8_t arrdiv16_fs17_or0 = 0;
|
|
uint8_t arrdiv16_fs18_xor1 = 0;
|
|
uint8_t arrdiv16_fs18_or0 = 0;
|
|
uint8_t arrdiv16_fs19_xor1 = 0;
|
|
uint8_t arrdiv16_fs19_or0 = 0;
|
|
uint8_t arrdiv16_fs20_xor1 = 0;
|
|
uint8_t arrdiv16_fs20_or0 = 0;
|
|
uint8_t arrdiv16_fs21_xor1 = 0;
|
|
uint8_t arrdiv16_fs21_or0 = 0;
|
|
uint8_t arrdiv16_fs22_xor1 = 0;
|
|
uint8_t arrdiv16_fs22_or0 = 0;
|
|
uint8_t arrdiv16_fs23_xor1 = 0;
|
|
uint8_t arrdiv16_fs23_or0 = 0;
|
|
uint8_t arrdiv16_fs24_xor1 = 0;
|
|
uint8_t arrdiv16_fs24_or0 = 0;
|
|
uint8_t arrdiv16_fs25_xor1 = 0;
|
|
uint8_t arrdiv16_fs25_or0 = 0;
|
|
uint8_t arrdiv16_fs26_xor1 = 0;
|
|
uint8_t arrdiv16_fs26_or0 = 0;
|
|
uint8_t arrdiv16_fs27_xor1 = 0;
|
|
uint8_t arrdiv16_fs27_or0 = 0;
|
|
uint8_t arrdiv16_fs28_xor1 = 0;
|
|
uint8_t arrdiv16_fs28_or0 = 0;
|
|
uint8_t arrdiv16_fs29_xor1 = 0;
|
|
uint8_t arrdiv16_fs29_or0 = 0;
|
|
uint8_t arrdiv16_fs30_xor1 = 0;
|
|
uint8_t arrdiv16_fs30_or0 = 0;
|
|
uint8_t arrdiv16_fs31_xor1 = 0;
|
|
uint8_t arrdiv16_fs31_or0 = 0;
|
|
uint8_t arrdiv16_mux2to115_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to116_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to117_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to118_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to119_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to120_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to121_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to122_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to123_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to124_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to125_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to126_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to127_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to128_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to129_xor0 = 0;
|
|
uint8_t arrdiv16_not1 = 0;
|
|
uint8_t arrdiv16_fs32_xor0 = 0;
|
|
uint8_t arrdiv16_fs32_and0 = 0;
|
|
uint8_t arrdiv16_fs33_xor1 = 0;
|
|
uint8_t arrdiv16_fs33_or0 = 0;
|
|
uint8_t arrdiv16_fs34_xor1 = 0;
|
|
uint8_t arrdiv16_fs34_or0 = 0;
|
|
uint8_t arrdiv16_fs35_xor1 = 0;
|
|
uint8_t arrdiv16_fs35_or0 = 0;
|
|
uint8_t arrdiv16_fs36_xor1 = 0;
|
|
uint8_t arrdiv16_fs36_or0 = 0;
|
|
uint8_t arrdiv16_fs37_xor1 = 0;
|
|
uint8_t arrdiv16_fs37_or0 = 0;
|
|
uint8_t arrdiv16_fs38_xor1 = 0;
|
|
uint8_t arrdiv16_fs38_or0 = 0;
|
|
uint8_t arrdiv16_fs39_xor1 = 0;
|
|
uint8_t arrdiv16_fs39_or0 = 0;
|
|
uint8_t arrdiv16_fs40_xor1 = 0;
|
|
uint8_t arrdiv16_fs40_or0 = 0;
|
|
uint8_t arrdiv16_fs41_xor1 = 0;
|
|
uint8_t arrdiv16_fs41_or0 = 0;
|
|
uint8_t arrdiv16_fs42_xor1 = 0;
|
|
uint8_t arrdiv16_fs42_or0 = 0;
|
|
uint8_t arrdiv16_fs43_xor1 = 0;
|
|
uint8_t arrdiv16_fs43_or0 = 0;
|
|
uint8_t arrdiv16_fs44_xor1 = 0;
|
|
uint8_t arrdiv16_fs44_or0 = 0;
|
|
uint8_t arrdiv16_fs45_xor1 = 0;
|
|
uint8_t arrdiv16_fs45_or0 = 0;
|
|
uint8_t arrdiv16_fs46_xor1 = 0;
|
|
uint8_t arrdiv16_fs46_or0 = 0;
|
|
uint8_t arrdiv16_fs47_xor1 = 0;
|
|
uint8_t arrdiv16_fs47_or0 = 0;
|
|
uint8_t arrdiv16_mux2to130_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to131_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to132_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to133_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to134_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to135_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to136_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to137_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to138_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to139_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to140_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to141_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to142_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to143_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to144_xor0 = 0;
|
|
uint8_t arrdiv16_not2 = 0;
|
|
uint8_t arrdiv16_fs48_xor0 = 0;
|
|
uint8_t arrdiv16_fs48_and0 = 0;
|
|
uint8_t arrdiv16_fs49_xor1 = 0;
|
|
uint8_t arrdiv16_fs49_or0 = 0;
|
|
uint8_t arrdiv16_fs50_xor1 = 0;
|
|
uint8_t arrdiv16_fs50_or0 = 0;
|
|
uint8_t arrdiv16_fs51_xor1 = 0;
|
|
uint8_t arrdiv16_fs51_or0 = 0;
|
|
uint8_t arrdiv16_fs52_xor1 = 0;
|
|
uint8_t arrdiv16_fs52_or0 = 0;
|
|
uint8_t arrdiv16_fs53_xor1 = 0;
|
|
uint8_t arrdiv16_fs53_or0 = 0;
|
|
uint8_t arrdiv16_fs54_xor1 = 0;
|
|
uint8_t arrdiv16_fs54_or0 = 0;
|
|
uint8_t arrdiv16_fs55_xor1 = 0;
|
|
uint8_t arrdiv16_fs55_or0 = 0;
|
|
uint8_t arrdiv16_fs56_xor1 = 0;
|
|
uint8_t arrdiv16_fs56_or0 = 0;
|
|
uint8_t arrdiv16_fs57_xor1 = 0;
|
|
uint8_t arrdiv16_fs57_or0 = 0;
|
|
uint8_t arrdiv16_fs58_xor1 = 0;
|
|
uint8_t arrdiv16_fs58_or0 = 0;
|
|
uint8_t arrdiv16_fs59_xor1 = 0;
|
|
uint8_t arrdiv16_fs59_or0 = 0;
|
|
uint8_t arrdiv16_fs60_xor1 = 0;
|
|
uint8_t arrdiv16_fs60_or0 = 0;
|
|
uint8_t arrdiv16_fs61_xor1 = 0;
|
|
uint8_t arrdiv16_fs61_or0 = 0;
|
|
uint8_t arrdiv16_fs62_xor1 = 0;
|
|
uint8_t arrdiv16_fs62_or0 = 0;
|
|
uint8_t arrdiv16_fs63_xor1 = 0;
|
|
uint8_t arrdiv16_fs63_or0 = 0;
|
|
uint8_t arrdiv16_mux2to145_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to146_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to147_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to148_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to149_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to150_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to151_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to152_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to153_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to154_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to155_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to156_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to157_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to158_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to159_xor0 = 0;
|
|
uint8_t arrdiv16_not3 = 0;
|
|
uint8_t arrdiv16_fs64_xor0 = 0;
|
|
uint8_t arrdiv16_fs64_and0 = 0;
|
|
uint8_t arrdiv16_fs65_xor1 = 0;
|
|
uint8_t arrdiv16_fs65_or0 = 0;
|
|
uint8_t arrdiv16_fs66_xor1 = 0;
|
|
uint8_t arrdiv16_fs66_or0 = 0;
|
|
uint8_t arrdiv16_fs67_xor1 = 0;
|
|
uint8_t arrdiv16_fs67_or0 = 0;
|
|
uint8_t arrdiv16_fs68_xor1 = 0;
|
|
uint8_t arrdiv16_fs68_or0 = 0;
|
|
uint8_t arrdiv16_fs69_xor1 = 0;
|
|
uint8_t arrdiv16_fs69_or0 = 0;
|
|
uint8_t arrdiv16_fs70_xor1 = 0;
|
|
uint8_t arrdiv16_fs70_or0 = 0;
|
|
uint8_t arrdiv16_fs71_xor1 = 0;
|
|
uint8_t arrdiv16_fs71_or0 = 0;
|
|
uint8_t arrdiv16_fs72_xor1 = 0;
|
|
uint8_t arrdiv16_fs72_or0 = 0;
|
|
uint8_t arrdiv16_fs73_xor1 = 0;
|
|
uint8_t arrdiv16_fs73_or0 = 0;
|
|
uint8_t arrdiv16_fs74_xor1 = 0;
|
|
uint8_t arrdiv16_fs74_or0 = 0;
|
|
uint8_t arrdiv16_fs75_xor1 = 0;
|
|
uint8_t arrdiv16_fs75_or0 = 0;
|
|
uint8_t arrdiv16_fs76_xor1 = 0;
|
|
uint8_t arrdiv16_fs76_or0 = 0;
|
|
uint8_t arrdiv16_fs77_xor1 = 0;
|
|
uint8_t arrdiv16_fs77_or0 = 0;
|
|
uint8_t arrdiv16_fs78_xor1 = 0;
|
|
uint8_t arrdiv16_fs78_or0 = 0;
|
|
uint8_t arrdiv16_fs79_xor1 = 0;
|
|
uint8_t arrdiv16_fs79_or0 = 0;
|
|
uint8_t arrdiv16_mux2to160_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to161_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to162_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to163_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to164_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to165_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to166_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to167_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to168_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to169_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to170_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to171_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to172_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to173_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to174_xor0 = 0;
|
|
uint8_t arrdiv16_not4 = 0;
|
|
uint8_t arrdiv16_fs80_xor0 = 0;
|
|
uint8_t arrdiv16_fs80_and0 = 0;
|
|
uint8_t arrdiv16_fs81_xor1 = 0;
|
|
uint8_t arrdiv16_fs81_or0 = 0;
|
|
uint8_t arrdiv16_fs82_xor1 = 0;
|
|
uint8_t arrdiv16_fs82_or0 = 0;
|
|
uint8_t arrdiv16_fs83_xor1 = 0;
|
|
uint8_t arrdiv16_fs83_or0 = 0;
|
|
uint8_t arrdiv16_fs84_xor1 = 0;
|
|
uint8_t arrdiv16_fs84_or0 = 0;
|
|
uint8_t arrdiv16_fs85_xor1 = 0;
|
|
uint8_t arrdiv16_fs85_or0 = 0;
|
|
uint8_t arrdiv16_fs86_xor1 = 0;
|
|
uint8_t arrdiv16_fs86_or0 = 0;
|
|
uint8_t arrdiv16_fs87_xor1 = 0;
|
|
uint8_t arrdiv16_fs87_or0 = 0;
|
|
uint8_t arrdiv16_fs88_xor1 = 0;
|
|
uint8_t arrdiv16_fs88_or0 = 0;
|
|
uint8_t arrdiv16_fs89_xor1 = 0;
|
|
uint8_t arrdiv16_fs89_or0 = 0;
|
|
uint8_t arrdiv16_fs90_xor1 = 0;
|
|
uint8_t arrdiv16_fs90_or0 = 0;
|
|
uint8_t arrdiv16_fs91_xor1 = 0;
|
|
uint8_t arrdiv16_fs91_or0 = 0;
|
|
uint8_t arrdiv16_fs92_xor1 = 0;
|
|
uint8_t arrdiv16_fs92_or0 = 0;
|
|
uint8_t arrdiv16_fs93_xor1 = 0;
|
|
uint8_t arrdiv16_fs93_or0 = 0;
|
|
uint8_t arrdiv16_fs94_xor1 = 0;
|
|
uint8_t arrdiv16_fs94_or0 = 0;
|
|
uint8_t arrdiv16_fs95_xor1 = 0;
|
|
uint8_t arrdiv16_fs95_or0 = 0;
|
|
uint8_t arrdiv16_mux2to175_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to176_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to177_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to178_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to179_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to180_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to181_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to182_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to183_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to184_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to185_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to186_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to187_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to188_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to189_xor0 = 0;
|
|
uint8_t arrdiv16_not5 = 0;
|
|
uint8_t arrdiv16_fs96_xor0 = 0;
|
|
uint8_t arrdiv16_fs96_and0 = 0;
|
|
uint8_t arrdiv16_fs97_xor1 = 0;
|
|
uint8_t arrdiv16_fs97_or0 = 0;
|
|
uint8_t arrdiv16_fs98_xor1 = 0;
|
|
uint8_t arrdiv16_fs98_or0 = 0;
|
|
uint8_t arrdiv16_fs99_xor1 = 0;
|
|
uint8_t arrdiv16_fs99_or0 = 0;
|
|
uint8_t arrdiv16_fs100_xor1 = 0;
|
|
uint8_t arrdiv16_fs100_or0 = 0;
|
|
uint8_t arrdiv16_fs101_xor1 = 0;
|
|
uint8_t arrdiv16_fs101_or0 = 0;
|
|
uint8_t arrdiv16_fs102_xor1 = 0;
|
|
uint8_t arrdiv16_fs102_or0 = 0;
|
|
uint8_t arrdiv16_fs103_xor1 = 0;
|
|
uint8_t arrdiv16_fs103_or0 = 0;
|
|
uint8_t arrdiv16_fs104_xor1 = 0;
|
|
uint8_t arrdiv16_fs104_or0 = 0;
|
|
uint8_t arrdiv16_fs105_xor1 = 0;
|
|
uint8_t arrdiv16_fs105_or0 = 0;
|
|
uint8_t arrdiv16_fs106_xor1 = 0;
|
|
uint8_t arrdiv16_fs106_or0 = 0;
|
|
uint8_t arrdiv16_fs107_xor1 = 0;
|
|
uint8_t arrdiv16_fs107_or0 = 0;
|
|
uint8_t arrdiv16_fs108_xor1 = 0;
|
|
uint8_t arrdiv16_fs108_or0 = 0;
|
|
uint8_t arrdiv16_fs109_xor1 = 0;
|
|
uint8_t arrdiv16_fs109_or0 = 0;
|
|
uint8_t arrdiv16_fs110_xor1 = 0;
|
|
uint8_t arrdiv16_fs110_or0 = 0;
|
|
uint8_t arrdiv16_fs111_xor1 = 0;
|
|
uint8_t arrdiv16_fs111_or0 = 0;
|
|
uint8_t arrdiv16_mux2to190_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to191_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to192_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to193_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to194_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to195_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to196_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to197_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to198_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to199_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1100_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1101_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1102_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1103_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1104_xor0 = 0;
|
|
uint8_t arrdiv16_not6 = 0;
|
|
uint8_t arrdiv16_fs112_xor0 = 0;
|
|
uint8_t arrdiv16_fs112_and0 = 0;
|
|
uint8_t arrdiv16_fs113_xor1 = 0;
|
|
uint8_t arrdiv16_fs113_or0 = 0;
|
|
uint8_t arrdiv16_fs114_xor1 = 0;
|
|
uint8_t arrdiv16_fs114_or0 = 0;
|
|
uint8_t arrdiv16_fs115_xor1 = 0;
|
|
uint8_t arrdiv16_fs115_or0 = 0;
|
|
uint8_t arrdiv16_fs116_xor1 = 0;
|
|
uint8_t arrdiv16_fs116_or0 = 0;
|
|
uint8_t arrdiv16_fs117_xor1 = 0;
|
|
uint8_t arrdiv16_fs117_or0 = 0;
|
|
uint8_t arrdiv16_fs118_xor1 = 0;
|
|
uint8_t arrdiv16_fs118_or0 = 0;
|
|
uint8_t arrdiv16_fs119_xor1 = 0;
|
|
uint8_t arrdiv16_fs119_or0 = 0;
|
|
uint8_t arrdiv16_fs120_xor1 = 0;
|
|
uint8_t arrdiv16_fs120_or0 = 0;
|
|
uint8_t arrdiv16_fs121_xor1 = 0;
|
|
uint8_t arrdiv16_fs121_or0 = 0;
|
|
uint8_t arrdiv16_fs122_xor1 = 0;
|
|
uint8_t arrdiv16_fs122_or0 = 0;
|
|
uint8_t arrdiv16_fs123_xor1 = 0;
|
|
uint8_t arrdiv16_fs123_or0 = 0;
|
|
uint8_t arrdiv16_fs124_xor1 = 0;
|
|
uint8_t arrdiv16_fs124_or0 = 0;
|
|
uint8_t arrdiv16_fs125_xor1 = 0;
|
|
uint8_t arrdiv16_fs125_or0 = 0;
|
|
uint8_t arrdiv16_fs126_xor1 = 0;
|
|
uint8_t arrdiv16_fs126_or0 = 0;
|
|
uint8_t arrdiv16_fs127_xor1 = 0;
|
|
uint8_t arrdiv16_fs127_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1105_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1106_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1107_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1108_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1109_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1110_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1111_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1112_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1113_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1114_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1115_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1116_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1117_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1118_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1119_xor0 = 0;
|
|
uint8_t arrdiv16_not7 = 0;
|
|
uint8_t arrdiv16_fs128_xor0 = 0;
|
|
uint8_t arrdiv16_fs128_and0 = 0;
|
|
uint8_t arrdiv16_fs129_xor1 = 0;
|
|
uint8_t arrdiv16_fs129_or0 = 0;
|
|
uint8_t arrdiv16_fs130_xor1 = 0;
|
|
uint8_t arrdiv16_fs130_or0 = 0;
|
|
uint8_t arrdiv16_fs131_xor1 = 0;
|
|
uint8_t arrdiv16_fs131_or0 = 0;
|
|
uint8_t arrdiv16_fs132_xor1 = 0;
|
|
uint8_t arrdiv16_fs132_or0 = 0;
|
|
uint8_t arrdiv16_fs133_xor1 = 0;
|
|
uint8_t arrdiv16_fs133_or0 = 0;
|
|
uint8_t arrdiv16_fs134_xor1 = 0;
|
|
uint8_t arrdiv16_fs134_or0 = 0;
|
|
uint8_t arrdiv16_fs135_xor1 = 0;
|
|
uint8_t arrdiv16_fs135_or0 = 0;
|
|
uint8_t arrdiv16_fs136_xor1 = 0;
|
|
uint8_t arrdiv16_fs136_or0 = 0;
|
|
uint8_t arrdiv16_fs137_xor1 = 0;
|
|
uint8_t arrdiv16_fs137_or0 = 0;
|
|
uint8_t arrdiv16_fs138_xor1 = 0;
|
|
uint8_t arrdiv16_fs138_or0 = 0;
|
|
uint8_t arrdiv16_fs139_xor1 = 0;
|
|
uint8_t arrdiv16_fs139_or0 = 0;
|
|
uint8_t arrdiv16_fs140_xor1 = 0;
|
|
uint8_t arrdiv16_fs140_or0 = 0;
|
|
uint8_t arrdiv16_fs141_xor1 = 0;
|
|
uint8_t arrdiv16_fs141_or0 = 0;
|
|
uint8_t arrdiv16_fs142_xor1 = 0;
|
|
uint8_t arrdiv16_fs142_or0 = 0;
|
|
uint8_t arrdiv16_fs143_xor1 = 0;
|
|
uint8_t arrdiv16_fs143_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1120_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1121_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1122_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1123_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1124_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1125_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1126_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1127_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1128_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1129_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1130_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1131_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1132_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1133_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1134_xor0 = 0;
|
|
uint8_t arrdiv16_not8 = 0;
|
|
uint8_t arrdiv16_fs144_xor0 = 0;
|
|
uint8_t arrdiv16_fs144_and0 = 0;
|
|
uint8_t arrdiv16_fs145_xor1 = 0;
|
|
uint8_t arrdiv16_fs145_or0 = 0;
|
|
uint8_t arrdiv16_fs146_xor1 = 0;
|
|
uint8_t arrdiv16_fs146_or0 = 0;
|
|
uint8_t arrdiv16_fs147_xor1 = 0;
|
|
uint8_t arrdiv16_fs147_or0 = 0;
|
|
uint8_t arrdiv16_fs148_xor1 = 0;
|
|
uint8_t arrdiv16_fs148_or0 = 0;
|
|
uint8_t arrdiv16_fs149_xor1 = 0;
|
|
uint8_t arrdiv16_fs149_or0 = 0;
|
|
uint8_t arrdiv16_fs150_xor1 = 0;
|
|
uint8_t arrdiv16_fs150_or0 = 0;
|
|
uint8_t arrdiv16_fs151_xor1 = 0;
|
|
uint8_t arrdiv16_fs151_or0 = 0;
|
|
uint8_t arrdiv16_fs152_xor1 = 0;
|
|
uint8_t arrdiv16_fs152_or0 = 0;
|
|
uint8_t arrdiv16_fs153_xor1 = 0;
|
|
uint8_t arrdiv16_fs153_or0 = 0;
|
|
uint8_t arrdiv16_fs154_xor1 = 0;
|
|
uint8_t arrdiv16_fs154_or0 = 0;
|
|
uint8_t arrdiv16_fs155_xor1 = 0;
|
|
uint8_t arrdiv16_fs155_or0 = 0;
|
|
uint8_t arrdiv16_fs156_xor1 = 0;
|
|
uint8_t arrdiv16_fs156_or0 = 0;
|
|
uint8_t arrdiv16_fs157_xor1 = 0;
|
|
uint8_t arrdiv16_fs157_or0 = 0;
|
|
uint8_t arrdiv16_fs158_xor1 = 0;
|
|
uint8_t arrdiv16_fs158_or0 = 0;
|
|
uint8_t arrdiv16_fs159_xor1 = 0;
|
|
uint8_t arrdiv16_fs159_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1135_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1136_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1137_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1138_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1139_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1140_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1141_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1142_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1143_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1144_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1145_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1146_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1147_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1148_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1149_xor0 = 0;
|
|
uint8_t arrdiv16_not9 = 0;
|
|
uint8_t arrdiv16_fs160_xor0 = 0;
|
|
uint8_t arrdiv16_fs160_and0 = 0;
|
|
uint8_t arrdiv16_fs161_xor1 = 0;
|
|
uint8_t arrdiv16_fs161_or0 = 0;
|
|
uint8_t arrdiv16_fs162_xor1 = 0;
|
|
uint8_t arrdiv16_fs162_or0 = 0;
|
|
uint8_t arrdiv16_fs163_xor1 = 0;
|
|
uint8_t arrdiv16_fs163_or0 = 0;
|
|
uint8_t arrdiv16_fs164_xor1 = 0;
|
|
uint8_t arrdiv16_fs164_or0 = 0;
|
|
uint8_t arrdiv16_fs165_xor1 = 0;
|
|
uint8_t arrdiv16_fs165_or0 = 0;
|
|
uint8_t arrdiv16_fs166_xor1 = 0;
|
|
uint8_t arrdiv16_fs166_or0 = 0;
|
|
uint8_t arrdiv16_fs167_xor1 = 0;
|
|
uint8_t arrdiv16_fs167_or0 = 0;
|
|
uint8_t arrdiv16_fs168_xor1 = 0;
|
|
uint8_t arrdiv16_fs168_or0 = 0;
|
|
uint8_t arrdiv16_fs169_xor1 = 0;
|
|
uint8_t arrdiv16_fs169_or0 = 0;
|
|
uint8_t arrdiv16_fs170_xor1 = 0;
|
|
uint8_t arrdiv16_fs170_or0 = 0;
|
|
uint8_t arrdiv16_fs171_xor1 = 0;
|
|
uint8_t arrdiv16_fs171_or0 = 0;
|
|
uint8_t arrdiv16_fs172_xor1 = 0;
|
|
uint8_t arrdiv16_fs172_or0 = 0;
|
|
uint8_t arrdiv16_fs173_xor1 = 0;
|
|
uint8_t arrdiv16_fs173_or0 = 0;
|
|
uint8_t arrdiv16_fs174_xor1 = 0;
|
|
uint8_t arrdiv16_fs174_or0 = 0;
|
|
uint8_t arrdiv16_fs175_xor1 = 0;
|
|
uint8_t arrdiv16_fs175_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1150_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1151_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1152_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1153_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1154_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1155_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1156_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1157_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1158_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1159_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1160_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1161_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1162_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1163_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1164_xor0 = 0;
|
|
uint8_t arrdiv16_not10 = 0;
|
|
uint8_t arrdiv16_fs176_xor0 = 0;
|
|
uint8_t arrdiv16_fs176_and0 = 0;
|
|
uint8_t arrdiv16_fs177_xor1 = 0;
|
|
uint8_t arrdiv16_fs177_or0 = 0;
|
|
uint8_t arrdiv16_fs178_xor1 = 0;
|
|
uint8_t arrdiv16_fs178_or0 = 0;
|
|
uint8_t arrdiv16_fs179_xor1 = 0;
|
|
uint8_t arrdiv16_fs179_or0 = 0;
|
|
uint8_t arrdiv16_fs180_xor1 = 0;
|
|
uint8_t arrdiv16_fs180_or0 = 0;
|
|
uint8_t arrdiv16_fs181_xor1 = 0;
|
|
uint8_t arrdiv16_fs181_or0 = 0;
|
|
uint8_t arrdiv16_fs182_xor1 = 0;
|
|
uint8_t arrdiv16_fs182_or0 = 0;
|
|
uint8_t arrdiv16_fs183_xor1 = 0;
|
|
uint8_t arrdiv16_fs183_or0 = 0;
|
|
uint8_t arrdiv16_fs184_xor1 = 0;
|
|
uint8_t arrdiv16_fs184_or0 = 0;
|
|
uint8_t arrdiv16_fs185_xor1 = 0;
|
|
uint8_t arrdiv16_fs185_or0 = 0;
|
|
uint8_t arrdiv16_fs186_xor1 = 0;
|
|
uint8_t arrdiv16_fs186_or0 = 0;
|
|
uint8_t arrdiv16_fs187_xor1 = 0;
|
|
uint8_t arrdiv16_fs187_or0 = 0;
|
|
uint8_t arrdiv16_fs188_xor1 = 0;
|
|
uint8_t arrdiv16_fs188_or0 = 0;
|
|
uint8_t arrdiv16_fs189_xor1 = 0;
|
|
uint8_t arrdiv16_fs189_or0 = 0;
|
|
uint8_t arrdiv16_fs190_xor1 = 0;
|
|
uint8_t arrdiv16_fs190_or0 = 0;
|
|
uint8_t arrdiv16_fs191_xor1 = 0;
|
|
uint8_t arrdiv16_fs191_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1165_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1166_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1167_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1168_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1169_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1170_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1171_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1172_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1173_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1174_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1175_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1176_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1177_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1178_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1179_xor0 = 0;
|
|
uint8_t arrdiv16_not11 = 0;
|
|
uint8_t arrdiv16_fs192_xor0 = 0;
|
|
uint8_t arrdiv16_fs192_and0 = 0;
|
|
uint8_t arrdiv16_fs193_xor1 = 0;
|
|
uint8_t arrdiv16_fs193_or0 = 0;
|
|
uint8_t arrdiv16_fs194_xor1 = 0;
|
|
uint8_t arrdiv16_fs194_or0 = 0;
|
|
uint8_t arrdiv16_fs195_xor1 = 0;
|
|
uint8_t arrdiv16_fs195_or0 = 0;
|
|
uint8_t arrdiv16_fs196_xor1 = 0;
|
|
uint8_t arrdiv16_fs196_or0 = 0;
|
|
uint8_t arrdiv16_fs197_xor1 = 0;
|
|
uint8_t arrdiv16_fs197_or0 = 0;
|
|
uint8_t arrdiv16_fs198_xor1 = 0;
|
|
uint8_t arrdiv16_fs198_or0 = 0;
|
|
uint8_t arrdiv16_fs199_xor1 = 0;
|
|
uint8_t arrdiv16_fs199_or0 = 0;
|
|
uint8_t arrdiv16_fs200_xor1 = 0;
|
|
uint8_t arrdiv16_fs200_or0 = 0;
|
|
uint8_t arrdiv16_fs201_xor1 = 0;
|
|
uint8_t arrdiv16_fs201_or0 = 0;
|
|
uint8_t arrdiv16_fs202_xor1 = 0;
|
|
uint8_t arrdiv16_fs202_or0 = 0;
|
|
uint8_t arrdiv16_fs203_xor1 = 0;
|
|
uint8_t arrdiv16_fs203_or0 = 0;
|
|
uint8_t arrdiv16_fs204_xor1 = 0;
|
|
uint8_t arrdiv16_fs204_or0 = 0;
|
|
uint8_t arrdiv16_fs205_xor1 = 0;
|
|
uint8_t arrdiv16_fs205_or0 = 0;
|
|
uint8_t arrdiv16_fs206_xor1 = 0;
|
|
uint8_t arrdiv16_fs206_or0 = 0;
|
|
uint8_t arrdiv16_fs207_xor1 = 0;
|
|
uint8_t arrdiv16_fs207_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1180_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1181_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1182_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1183_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1184_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1185_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1186_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1187_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1188_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1189_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1190_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1191_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1192_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1193_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1194_xor0 = 0;
|
|
uint8_t arrdiv16_not12 = 0;
|
|
uint8_t arrdiv16_fs208_xor0 = 0;
|
|
uint8_t arrdiv16_fs208_and0 = 0;
|
|
uint8_t arrdiv16_fs209_xor1 = 0;
|
|
uint8_t arrdiv16_fs209_or0 = 0;
|
|
uint8_t arrdiv16_fs210_xor1 = 0;
|
|
uint8_t arrdiv16_fs210_or0 = 0;
|
|
uint8_t arrdiv16_fs211_xor1 = 0;
|
|
uint8_t arrdiv16_fs211_or0 = 0;
|
|
uint8_t arrdiv16_fs212_xor1 = 0;
|
|
uint8_t arrdiv16_fs212_or0 = 0;
|
|
uint8_t arrdiv16_fs213_xor1 = 0;
|
|
uint8_t arrdiv16_fs213_or0 = 0;
|
|
uint8_t arrdiv16_fs214_xor1 = 0;
|
|
uint8_t arrdiv16_fs214_or0 = 0;
|
|
uint8_t arrdiv16_fs215_xor1 = 0;
|
|
uint8_t arrdiv16_fs215_or0 = 0;
|
|
uint8_t arrdiv16_fs216_xor1 = 0;
|
|
uint8_t arrdiv16_fs216_or0 = 0;
|
|
uint8_t arrdiv16_fs217_xor1 = 0;
|
|
uint8_t arrdiv16_fs217_or0 = 0;
|
|
uint8_t arrdiv16_fs218_xor1 = 0;
|
|
uint8_t arrdiv16_fs218_or0 = 0;
|
|
uint8_t arrdiv16_fs219_xor1 = 0;
|
|
uint8_t arrdiv16_fs219_or0 = 0;
|
|
uint8_t arrdiv16_fs220_xor1 = 0;
|
|
uint8_t arrdiv16_fs220_or0 = 0;
|
|
uint8_t arrdiv16_fs221_xor1 = 0;
|
|
uint8_t arrdiv16_fs221_or0 = 0;
|
|
uint8_t arrdiv16_fs222_xor1 = 0;
|
|
uint8_t arrdiv16_fs222_or0 = 0;
|
|
uint8_t arrdiv16_fs223_xor1 = 0;
|
|
uint8_t arrdiv16_fs223_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1195_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1196_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1197_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1198_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1199_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1200_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1201_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1202_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1203_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1204_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1205_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1206_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1207_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1208_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1209_xor0 = 0;
|
|
uint8_t arrdiv16_not13 = 0;
|
|
uint8_t arrdiv16_fs224_xor0 = 0;
|
|
uint8_t arrdiv16_fs224_and0 = 0;
|
|
uint8_t arrdiv16_fs225_xor1 = 0;
|
|
uint8_t arrdiv16_fs225_or0 = 0;
|
|
uint8_t arrdiv16_fs226_xor1 = 0;
|
|
uint8_t arrdiv16_fs226_or0 = 0;
|
|
uint8_t arrdiv16_fs227_xor1 = 0;
|
|
uint8_t arrdiv16_fs227_or0 = 0;
|
|
uint8_t arrdiv16_fs228_xor1 = 0;
|
|
uint8_t arrdiv16_fs228_or0 = 0;
|
|
uint8_t arrdiv16_fs229_xor1 = 0;
|
|
uint8_t arrdiv16_fs229_or0 = 0;
|
|
uint8_t arrdiv16_fs230_xor1 = 0;
|
|
uint8_t arrdiv16_fs230_or0 = 0;
|
|
uint8_t arrdiv16_fs231_xor1 = 0;
|
|
uint8_t arrdiv16_fs231_or0 = 0;
|
|
uint8_t arrdiv16_fs232_xor1 = 0;
|
|
uint8_t arrdiv16_fs232_or0 = 0;
|
|
uint8_t arrdiv16_fs233_xor1 = 0;
|
|
uint8_t arrdiv16_fs233_or0 = 0;
|
|
uint8_t arrdiv16_fs234_xor1 = 0;
|
|
uint8_t arrdiv16_fs234_or0 = 0;
|
|
uint8_t arrdiv16_fs235_xor1 = 0;
|
|
uint8_t arrdiv16_fs235_or0 = 0;
|
|
uint8_t arrdiv16_fs236_xor1 = 0;
|
|
uint8_t arrdiv16_fs236_or0 = 0;
|
|
uint8_t arrdiv16_fs237_xor1 = 0;
|
|
uint8_t arrdiv16_fs237_or0 = 0;
|
|
uint8_t arrdiv16_fs238_xor1 = 0;
|
|
uint8_t arrdiv16_fs238_or0 = 0;
|
|
uint8_t arrdiv16_fs239_xor1 = 0;
|
|
uint8_t arrdiv16_fs239_or0 = 0;
|
|
uint8_t arrdiv16_mux2to1210_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1211_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1212_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1213_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1214_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1215_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1216_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1217_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1218_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1219_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1220_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1221_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1222_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1223_xor0 = 0;
|
|
uint8_t arrdiv16_mux2to1224_xor0 = 0;
|
|
uint8_t arrdiv16_not14 = 0;
|
|
uint8_t arrdiv16_fs240_xor0 = 0;
|
|
uint8_t arrdiv16_fs240_and0 = 0;
|
|
uint8_t arrdiv16_fs241_xor1 = 0;
|
|
uint8_t arrdiv16_fs241_or0 = 0;
|
|
uint8_t arrdiv16_fs242_xor1 = 0;
|
|
uint8_t arrdiv16_fs242_or0 = 0;
|
|
uint8_t arrdiv16_fs243_xor1 = 0;
|
|
uint8_t arrdiv16_fs243_or0 = 0;
|
|
uint8_t arrdiv16_fs244_xor1 = 0;
|
|
uint8_t arrdiv16_fs244_or0 = 0;
|
|
uint8_t arrdiv16_fs245_xor1 = 0;
|
|
uint8_t arrdiv16_fs245_or0 = 0;
|
|
uint8_t arrdiv16_fs246_xor1 = 0;
|
|
uint8_t arrdiv16_fs246_or0 = 0;
|
|
uint8_t arrdiv16_fs247_xor1 = 0;
|
|
uint8_t arrdiv16_fs247_or0 = 0;
|
|
uint8_t arrdiv16_fs248_xor1 = 0;
|
|
uint8_t arrdiv16_fs248_or0 = 0;
|
|
uint8_t arrdiv16_fs249_xor1 = 0;
|
|
uint8_t arrdiv16_fs249_or0 = 0;
|
|
uint8_t arrdiv16_fs250_xor1 = 0;
|
|
uint8_t arrdiv16_fs250_or0 = 0;
|
|
uint8_t arrdiv16_fs251_xor1 = 0;
|
|
uint8_t arrdiv16_fs251_or0 = 0;
|
|
uint8_t arrdiv16_fs252_xor1 = 0;
|
|
uint8_t arrdiv16_fs252_or0 = 0;
|
|
uint8_t arrdiv16_fs253_xor1 = 0;
|
|
uint8_t arrdiv16_fs253_or0 = 0;
|
|
uint8_t arrdiv16_fs254_xor1 = 0;
|
|
uint8_t arrdiv16_fs254_or0 = 0;
|
|
uint8_t arrdiv16_fs255_xor1 = 0;
|
|
uint8_t arrdiv16_fs255_or0 = 0;
|
|
uint8_t arrdiv16_not15 = 0;
|
|
|
|
arrdiv16_fs0_xor0 = (fs(((a >> 15) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs0_and0 = (fs(((a >> 15) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs1_xor1 = (fs((0x00), ((b >> 1) & 0x01), ((arrdiv16_fs0_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs1_or0 = (fs((0x00), ((b >> 1) & 0x01), ((arrdiv16_fs0_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs2_xor1 = (fs((0x00), ((b >> 2) & 0x01), ((arrdiv16_fs1_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs2_or0 = (fs((0x00), ((b >> 2) & 0x01), ((arrdiv16_fs1_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs3_xor1 = (fs((0x00), ((b >> 3) & 0x01), ((arrdiv16_fs2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs3_or0 = (fs((0x00), ((b >> 3) & 0x01), ((arrdiv16_fs2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs4_xor1 = (fs((0x00), ((b >> 4) & 0x01), ((arrdiv16_fs3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs4_or0 = (fs((0x00), ((b >> 4) & 0x01), ((arrdiv16_fs3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs5_xor1 = (fs((0x00), ((b >> 5) & 0x01), ((arrdiv16_fs4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs5_or0 = (fs((0x00), ((b >> 5) & 0x01), ((arrdiv16_fs4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs6_xor1 = (fs((0x00), ((b >> 6) & 0x01), ((arrdiv16_fs5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs6_or0 = (fs((0x00), ((b >> 6) & 0x01), ((arrdiv16_fs5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs7_xor1 = (fs((0x00), ((b >> 7) & 0x01), ((arrdiv16_fs6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs7_or0 = (fs((0x00), ((b >> 7) & 0x01), ((arrdiv16_fs6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs8_xor1 = (fs((0x00), ((b >> 8) & 0x01), ((arrdiv16_fs7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs8_or0 = (fs((0x00), ((b >> 8) & 0x01), ((arrdiv16_fs7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs9_xor1 = (fs((0x00), ((b >> 9) & 0x01), ((arrdiv16_fs8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs9_or0 = (fs((0x00), ((b >> 9) & 0x01), ((arrdiv16_fs8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs10_xor1 = (fs((0x00), ((b >> 10) & 0x01), ((arrdiv16_fs9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs10_or0 = (fs((0x00), ((b >> 10) & 0x01), ((arrdiv16_fs9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs11_xor1 = (fs((0x00), ((b >> 11) & 0x01), ((arrdiv16_fs10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs11_or0 = (fs((0x00), ((b >> 11) & 0x01), ((arrdiv16_fs10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs12_xor1 = (fs((0x00), ((b >> 12) & 0x01), ((arrdiv16_fs11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs12_or0 = (fs((0x00), ((b >> 12) & 0x01), ((arrdiv16_fs11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs13_xor1 = (fs((0x00), ((b >> 13) & 0x01), ((arrdiv16_fs12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs13_or0 = (fs((0x00), ((b >> 13) & 0x01), ((arrdiv16_fs12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs14_xor1 = (fs((0x00), ((b >> 14) & 0x01), ((arrdiv16_fs13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs14_or0 = (fs((0x00), ((b >> 14) & 0x01), ((arrdiv16_fs13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs15_xor1 = (fs((0x00), ((b >> 15) & 0x01), ((arrdiv16_fs14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs15_or0 = (fs((0x00), ((b >> 15) & 0x01), ((arrdiv16_fs14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to10_xor0 = (mux2to1(((arrdiv16_fs0_xor0 >> 0) & 0x01), ((a >> 15) & 0x01), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to11_and1 = (mux2to1(((arrdiv16_fs1_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to12_and1 = (mux2to1(((arrdiv16_fs2_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to13_and1 = (mux2to1(((arrdiv16_fs3_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to14_and1 = (mux2to1(((arrdiv16_fs4_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to15_and1 = (mux2to1(((arrdiv16_fs5_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to16_and1 = (mux2to1(((arrdiv16_fs6_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to17_and1 = (mux2to1(((arrdiv16_fs7_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to18_and1 = (mux2to1(((arrdiv16_fs8_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to19_and1 = (mux2to1(((arrdiv16_fs9_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to110_and1 = (mux2to1(((arrdiv16_fs10_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to111_and1 = (mux2to1(((arrdiv16_fs11_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to112_and1 = (mux2to1(((arrdiv16_fs12_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to113_and1 = (mux2to1(((arrdiv16_fs13_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to114_and1 = (mux2to1(((arrdiv16_fs14_xor1 >> 0) & 0x01), (0x00), ((arrdiv16_fs15_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not0 = not_gate(((arrdiv16_fs15_or0 >> 0) & 0x01));
|
|
arrdiv16_fs16_xor0 = (fs(((a >> 14) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs16_and0 = (fs(((a >> 14) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs17_xor1 = (fs(((arrdiv16_mux2to10_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs16_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs17_or0 = (fs(((arrdiv16_mux2to10_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs16_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs18_xor1 = (fs(((arrdiv16_mux2to11_and1 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs17_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs18_or0 = (fs(((arrdiv16_mux2to11_and1 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs17_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs19_xor1 = (fs(((arrdiv16_mux2to12_and1 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs18_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs19_or0 = (fs(((arrdiv16_mux2to12_and1 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs18_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs20_xor1 = (fs(((arrdiv16_mux2to13_and1 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs19_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs20_or0 = (fs(((arrdiv16_mux2to13_and1 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs19_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs21_xor1 = (fs(((arrdiv16_mux2to14_and1 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs20_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs21_or0 = (fs(((arrdiv16_mux2to14_and1 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs20_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs22_xor1 = (fs(((arrdiv16_mux2to15_and1 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs21_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs22_or0 = (fs(((arrdiv16_mux2to15_and1 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs21_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs23_xor1 = (fs(((arrdiv16_mux2to16_and1 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs22_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs23_or0 = (fs(((arrdiv16_mux2to16_and1 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs22_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs24_xor1 = (fs(((arrdiv16_mux2to17_and1 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs23_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs24_or0 = (fs(((arrdiv16_mux2to17_and1 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs23_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs25_xor1 = (fs(((arrdiv16_mux2to18_and1 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs24_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs25_or0 = (fs(((arrdiv16_mux2to18_and1 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs24_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs26_xor1 = (fs(((arrdiv16_mux2to19_and1 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs25_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs26_or0 = (fs(((arrdiv16_mux2to19_and1 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs25_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs27_xor1 = (fs(((arrdiv16_mux2to110_and1 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs26_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs27_or0 = (fs(((arrdiv16_mux2to110_and1 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs26_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs28_xor1 = (fs(((arrdiv16_mux2to111_and1 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs27_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs28_or0 = (fs(((arrdiv16_mux2to111_and1 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs27_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs29_xor1 = (fs(((arrdiv16_mux2to112_and1 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs28_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs29_or0 = (fs(((arrdiv16_mux2to112_and1 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs28_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs30_xor1 = (fs(((arrdiv16_mux2to113_and1 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs29_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs30_or0 = (fs(((arrdiv16_mux2to113_and1 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs29_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs31_xor1 = (fs(((arrdiv16_mux2to114_and1 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs30_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs31_or0 = (fs(((arrdiv16_mux2to114_and1 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs30_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to115_xor0 = (mux2to1(((arrdiv16_fs16_xor0 >> 0) & 0x01), ((a >> 14) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to116_xor0 = (mux2to1(((arrdiv16_fs17_xor1 >> 0) & 0x01), ((arrdiv16_mux2to10_xor0 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to117_xor0 = (mux2to1(((arrdiv16_fs18_xor1 >> 0) & 0x01), ((arrdiv16_mux2to11_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to118_xor0 = (mux2to1(((arrdiv16_fs19_xor1 >> 0) & 0x01), ((arrdiv16_mux2to12_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to119_xor0 = (mux2to1(((arrdiv16_fs20_xor1 >> 0) & 0x01), ((arrdiv16_mux2to13_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to120_xor0 = (mux2to1(((arrdiv16_fs21_xor1 >> 0) & 0x01), ((arrdiv16_mux2to14_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to121_xor0 = (mux2to1(((arrdiv16_fs22_xor1 >> 0) & 0x01), ((arrdiv16_mux2to15_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to122_xor0 = (mux2to1(((arrdiv16_fs23_xor1 >> 0) & 0x01), ((arrdiv16_mux2to16_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to123_xor0 = (mux2to1(((arrdiv16_fs24_xor1 >> 0) & 0x01), ((arrdiv16_mux2to17_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to124_xor0 = (mux2to1(((arrdiv16_fs25_xor1 >> 0) & 0x01), ((arrdiv16_mux2to18_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to125_xor0 = (mux2to1(((arrdiv16_fs26_xor1 >> 0) & 0x01), ((arrdiv16_mux2to19_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to126_xor0 = (mux2to1(((arrdiv16_fs27_xor1 >> 0) & 0x01), ((arrdiv16_mux2to110_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to127_xor0 = (mux2to1(((arrdiv16_fs28_xor1 >> 0) & 0x01), ((arrdiv16_mux2to111_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to128_xor0 = (mux2to1(((arrdiv16_fs29_xor1 >> 0) & 0x01), ((arrdiv16_mux2to112_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to129_xor0 = (mux2to1(((arrdiv16_fs30_xor1 >> 0) & 0x01), ((arrdiv16_mux2to113_and1 >> 0) & 0x01), ((arrdiv16_fs31_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not1 = not_gate(((arrdiv16_fs31_or0 >> 0) & 0x01));
|
|
arrdiv16_fs32_xor0 = (fs(((a >> 13) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs32_and0 = (fs(((a >> 13) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs33_xor1 = (fs(((arrdiv16_mux2to115_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs32_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs33_or0 = (fs(((arrdiv16_mux2to115_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs32_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs34_xor1 = (fs(((arrdiv16_mux2to116_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs33_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs34_or0 = (fs(((arrdiv16_mux2to116_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs33_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs35_xor1 = (fs(((arrdiv16_mux2to117_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs34_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs35_or0 = (fs(((arrdiv16_mux2to117_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs34_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs36_xor1 = (fs(((arrdiv16_mux2to118_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs35_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs36_or0 = (fs(((arrdiv16_mux2to118_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs35_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs37_xor1 = (fs(((arrdiv16_mux2to119_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs36_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs37_or0 = (fs(((arrdiv16_mux2to119_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs36_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs38_xor1 = (fs(((arrdiv16_mux2to120_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs37_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs38_or0 = (fs(((arrdiv16_mux2to120_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs37_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs39_xor1 = (fs(((arrdiv16_mux2to121_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs38_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs39_or0 = (fs(((arrdiv16_mux2to121_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs38_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs40_xor1 = (fs(((arrdiv16_mux2to122_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs39_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs40_or0 = (fs(((arrdiv16_mux2to122_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs39_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs41_xor1 = (fs(((arrdiv16_mux2to123_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs40_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs41_or0 = (fs(((arrdiv16_mux2to123_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs40_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs42_xor1 = (fs(((arrdiv16_mux2to124_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs41_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs42_or0 = (fs(((arrdiv16_mux2to124_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs41_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs43_xor1 = (fs(((arrdiv16_mux2to125_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs42_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs43_or0 = (fs(((arrdiv16_mux2to125_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs42_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs44_xor1 = (fs(((arrdiv16_mux2to126_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs43_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs44_or0 = (fs(((arrdiv16_mux2to126_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs43_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs45_xor1 = (fs(((arrdiv16_mux2to127_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs44_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs45_or0 = (fs(((arrdiv16_mux2to127_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs44_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs46_xor1 = (fs(((arrdiv16_mux2to128_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs45_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs46_or0 = (fs(((arrdiv16_mux2to128_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs45_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs47_xor1 = (fs(((arrdiv16_mux2to129_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs46_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs47_or0 = (fs(((arrdiv16_mux2to129_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs46_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to130_xor0 = (mux2to1(((arrdiv16_fs32_xor0 >> 0) & 0x01), ((a >> 13) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to131_xor0 = (mux2to1(((arrdiv16_fs33_xor1 >> 0) & 0x01), ((arrdiv16_mux2to115_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to132_xor0 = (mux2to1(((arrdiv16_fs34_xor1 >> 0) & 0x01), ((arrdiv16_mux2to116_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to133_xor0 = (mux2to1(((arrdiv16_fs35_xor1 >> 0) & 0x01), ((arrdiv16_mux2to117_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to134_xor0 = (mux2to1(((arrdiv16_fs36_xor1 >> 0) & 0x01), ((arrdiv16_mux2to118_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to135_xor0 = (mux2to1(((arrdiv16_fs37_xor1 >> 0) & 0x01), ((arrdiv16_mux2to119_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to136_xor0 = (mux2to1(((arrdiv16_fs38_xor1 >> 0) & 0x01), ((arrdiv16_mux2to120_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to137_xor0 = (mux2to1(((arrdiv16_fs39_xor1 >> 0) & 0x01), ((arrdiv16_mux2to121_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to138_xor0 = (mux2to1(((arrdiv16_fs40_xor1 >> 0) & 0x01), ((arrdiv16_mux2to122_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to139_xor0 = (mux2to1(((arrdiv16_fs41_xor1 >> 0) & 0x01), ((arrdiv16_mux2to123_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to140_xor0 = (mux2to1(((arrdiv16_fs42_xor1 >> 0) & 0x01), ((arrdiv16_mux2to124_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to141_xor0 = (mux2to1(((arrdiv16_fs43_xor1 >> 0) & 0x01), ((arrdiv16_mux2to125_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to142_xor0 = (mux2to1(((arrdiv16_fs44_xor1 >> 0) & 0x01), ((arrdiv16_mux2to126_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to143_xor0 = (mux2to1(((arrdiv16_fs45_xor1 >> 0) & 0x01), ((arrdiv16_mux2to127_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to144_xor0 = (mux2to1(((arrdiv16_fs46_xor1 >> 0) & 0x01), ((arrdiv16_mux2to128_xor0 >> 0) & 0x01), ((arrdiv16_fs47_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not2 = not_gate(((arrdiv16_fs47_or0 >> 0) & 0x01));
|
|
arrdiv16_fs48_xor0 = (fs(((a >> 12) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs48_and0 = (fs(((a >> 12) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs49_xor1 = (fs(((arrdiv16_mux2to130_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs48_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs49_or0 = (fs(((arrdiv16_mux2to130_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs48_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs50_xor1 = (fs(((arrdiv16_mux2to131_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs49_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs50_or0 = (fs(((arrdiv16_mux2to131_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs49_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs51_xor1 = (fs(((arrdiv16_mux2to132_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs50_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs51_or0 = (fs(((arrdiv16_mux2to132_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs50_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs52_xor1 = (fs(((arrdiv16_mux2to133_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs51_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs52_or0 = (fs(((arrdiv16_mux2to133_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs51_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs53_xor1 = (fs(((arrdiv16_mux2to134_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs52_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs53_or0 = (fs(((arrdiv16_mux2to134_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs52_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs54_xor1 = (fs(((arrdiv16_mux2to135_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs53_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs54_or0 = (fs(((arrdiv16_mux2to135_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs53_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs55_xor1 = (fs(((arrdiv16_mux2to136_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs54_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs55_or0 = (fs(((arrdiv16_mux2to136_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs54_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs56_xor1 = (fs(((arrdiv16_mux2to137_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs55_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs56_or0 = (fs(((arrdiv16_mux2to137_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs55_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs57_xor1 = (fs(((arrdiv16_mux2to138_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs56_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs57_or0 = (fs(((arrdiv16_mux2to138_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs56_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs58_xor1 = (fs(((arrdiv16_mux2to139_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs57_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs58_or0 = (fs(((arrdiv16_mux2to139_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs57_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs59_xor1 = (fs(((arrdiv16_mux2to140_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs58_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs59_or0 = (fs(((arrdiv16_mux2to140_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs58_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs60_xor1 = (fs(((arrdiv16_mux2to141_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs59_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs60_or0 = (fs(((arrdiv16_mux2to141_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs59_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs61_xor1 = (fs(((arrdiv16_mux2to142_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs60_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs61_or0 = (fs(((arrdiv16_mux2to142_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs60_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs62_xor1 = (fs(((arrdiv16_mux2to143_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs61_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs62_or0 = (fs(((arrdiv16_mux2to143_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs61_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs63_xor1 = (fs(((arrdiv16_mux2to144_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs62_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs63_or0 = (fs(((arrdiv16_mux2to144_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs62_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to145_xor0 = (mux2to1(((arrdiv16_fs48_xor0 >> 0) & 0x01), ((a >> 12) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to146_xor0 = (mux2to1(((arrdiv16_fs49_xor1 >> 0) & 0x01), ((arrdiv16_mux2to130_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to147_xor0 = (mux2to1(((arrdiv16_fs50_xor1 >> 0) & 0x01), ((arrdiv16_mux2to131_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to148_xor0 = (mux2to1(((arrdiv16_fs51_xor1 >> 0) & 0x01), ((arrdiv16_mux2to132_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to149_xor0 = (mux2to1(((arrdiv16_fs52_xor1 >> 0) & 0x01), ((arrdiv16_mux2to133_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to150_xor0 = (mux2to1(((arrdiv16_fs53_xor1 >> 0) & 0x01), ((arrdiv16_mux2to134_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to151_xor0 = (mux2to1(((arrdiv16_fs54_xor1 >> 0) & 0x01), ((arrdiv16_mux2to135_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to152_xor0 = (mux2to1(((arrdiv16_fs55_xor1 >> 0) & 0x01), ((arrdiv16_mux2to136_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to153_xor0 = (mux2to1(((arrdiv16_fs56_xor1 >> 0) & 0x01), ((arrdiv16_mux2to137_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to154_xor0 = (mux2to1(((arrdiv16_fs57_xor1 >> 0) & 0x01), ((arrdiv16_mux2to138_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to155_xor0 = (mux2to1(((arrdiv16_fs58_xor1 >> 0) & 0x01), ((arrdiv16_mux2to139_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to156_xor0 = (mux2to1(((arrdiv16_fs59_xor1 >> 0) & 0x01), ((arrdiv16_mux2to140_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to157_xor0 = (mux2to1(((arrdiv16_fs60_xor1 >> 0) & 0x01), ((arrdiv16_mux2to141_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to158_xor0 = (mux2to1(((arrdiv16_fs61_xor1 >> 0) & 0x01), ((arrdiv16_mux2to142_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to159_xor0 = (mux2to1(((arrdiv16_fs62_xor1 >> 0) & 0x01), ((arrdiv16_mux2to143_xor0 >> 0) & 0x01), ((arrdiv16_fs63_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not3 = not_gate(((arrdiv16_fs63_or0 >> 0) & 0x01));
|
|
arrdiv16_fs64_xor0 = (fs(((a >> 11) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs64_and0 = (fs(((a >> 11) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs65_xor1 = (fs(((arrdiv16_mux2to145_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs64_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs65_or0 = (fs(((arrdiv16_mux2to145_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs64_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs66_xor1 = (fs(((arrdiv16_mux2to146_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs65_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs66_or0 = (fs(((arrdiv16_mux2to146_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs65_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs67_xor1 = (fs(((arrdiv16_mux2to147_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs66_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs67_or0 = (fs(((arrdiv16_mux2to147_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs66_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs68_xor1 = (fs(((arrdiv16_mux2to148_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs67_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs68_or0 = (fs(((arrdiv16_mux2to148_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs67_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs69_xor1 = (fs(((arrdiv16_mux2to149_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs68_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs69_or0 = (fs(((arrdiv16_mux2to149_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs68_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs70_xor1 = (fs(((arrdiv16_mux2to150_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs69_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs70_or0 = (fs(((arrdiv16_mux2to150_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs69_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs71_xor1 = (fs(((arrdiv16_mux2to151_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs70_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs71_or0 = (fs(((arrdiv16_mux2to151_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs70_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs72_xor1 = (fs(((arrdiv16_mux2to152_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs71_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs72_or0 = (fs(((arrdiv16_mux2to152_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs71_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs73_xor1 = (fs(((arrdiv16_mux2to153_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs72_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs73_or0 = (fs(((arrdiv16_mux2to153_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs72_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs74_xor1 = (fs(((arrdiv16_mux2to154_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs73_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs74_or0 = (fs(((arrdiv16_mux2to154_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs73_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs75_xor1 = (fs(((arrdiv16_mux2to155_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs74_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs75_or0 = (fs(((arrdiv16_mux2to155_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs74_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs76_xor1 = (fs(((arrdiv16_mux2to156_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs75_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs76_or0 = (fs(((arrdiv16_mux2to156_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs75_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs77_xor1 = (fs(((arrdiv16_mux2to157_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs76_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs77_or0 = (fs(((arrdiv16_mux2to157_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs76_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs78_xor1 = (fs(((arrdiv16_mux2to158_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs77_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs78_or0 = (fs(((arrdiv16_mux2to158_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs77_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs79_xor1 = (fs(((arrdiv16_mux2to159_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs78_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs79_or0 = (fs(((arrdiv16_mux2to159_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs78_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to160_xor0 = (mux2to1(((arrdiv16_fs64_xor0 >> 0) & 0x01), ((a >> 11) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to161_xor0 = (mux2to1(((arrdiv16_fs65_xor1 >> 0) & 0x01), ((arrdiv16_mux2to145_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to162_xor0 = (mux2to1(((arrdiv16_fs66_xor1 >> 0) & 0x01), ((arrdiv16_mux2to146_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to163_xor0 = (mux2to1(((arrdiv16_fs67_xor1 >> 0) & 0x01), ((arrdiv16_mux2to147_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to164_xor0 = (mux2to1(((arrdiv16_fs68_xor1 >> 0) & 0x01), ((arrdiv16_mux2to148_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to165_xor0 = (mux2to1(((arrdiv16_fs69_xor1 >> 0) & 0x01), ((arrdiv16_mux2to149_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to166_xor0 = (mux2to1(((arrdiv16_fs70_xor1 >> 0) & 0x01), ((arrdiv16_mux2to150_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to167_xor0 = (mux2to1(((arrdiv16_fs71_xor1 >> 0) & 0x01), ((arrdiv16_mux2to151_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to168_xor0 = (mux2to1(((arrdiv16_fs72_xor1 >> 0) & 0x01), ((arrdiv16_mux2to152_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to169_xor0 = (mux2to1(((arrdiv16_fs73_xor1 >> 0) & 0x01), ((arrdiv16_mux2to153_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to170_xor0 = (mux2to1(((arrdiv16_fs74_xor1 >> 0) & 0x01), ((arrdiv16_mux2to154_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to171_xor0 = (mux2to1(((arrdiv16_fs75_xor1 >> 0) & 0x01), ((arrdiv16_mux2to155_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to172_xor0 = (mux2to1(((arrdiv16_fs76_xor1 >> 0) & 0x01), ((arrdiv16_mux2to156_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to173_xor0 = (mux2to1(((arrdiv16_fs77_xor1 >> 0) & 0x01), ((arrdiv16_mux2to157_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to174_xor0 = (mux2to1(((arrdiv16_fs78_xor1 >> 0) & 0x01), ((arrdiv16_mux2to158_xor0 >> 0) & 0x01), ((arrdiv16_fs79_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not4 = not_gate(((arrdiv16_fs79_or0 >> 0) & 0x01));
|
|
arrdiv16_fs80_xor0 = (fs(((a >> 10) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs80_and0 = (fs(((a >> 10) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs81_xor1 = (fs(((arrdiv16_mux2to160_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs80_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs81_or0 = (fs(((arrdiv16_mux2to160_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs80_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs82_xor1 = (fs(((arrdiv16_mux2to161_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs81_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs82_or0 = (fs(((arrdiv16_mux2to161_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs81_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs83_xor1 = (fs(((arrdiv16_mux2to162_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs82_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs83_or0 = (fs(((arrdiv16_mux2to162_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs82_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs84_xor1 = (fs(((arrdiv16_mux2to163_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs83_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs84_or0 = (fs(((arrdiv16_mux2to163_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs83_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs85_xor1 = (fs(((arrdiv16_mux2to164_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs84_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs85_or0 = (fs(((arrdiv16_mux2to164_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs84_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs86_xor1 = (fs(((arrdiv16_mux2to165_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs85_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs86_or0 = (fs(((arrdiv16_mux2to165_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs85_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs87_xor1 = (fs(((arrdiv16_mux2to166_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs86_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs87_or0 = (fs(((arrdiv16_mux2to166_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs86_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs88_xor1 = (fs(((arrdiv16_mux2to167_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs87_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs88_or0 = (fs(((arrdiv16_mux2to167_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs87_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs89_xor1 = (fs(((arrdiv16_mux2to168_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs88_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs89_or0 = (fs(((arrdiv16_mux2to168_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs88_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs90_xor1 = (fs(((arrdiv16_mux2to169_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs89_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs90_or0 = (fs(((arrdiv16_mux2to169_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs89_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs91_xor1 = (fs(((arrdiv16_mux2to170_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs90_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs91_or0 = (fs(((arrdiv16_mux2to170_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs90_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs92_xor1 = (fs(((arrdiv16_mux2to171_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs91_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs92_or0 = (fs(((arrdiv16_mux2to171_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs91_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs93_xor1 = (fs(((arrdiv16_mux2to172_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs92_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs93_or0 = (fs(((arrdiv16_mux2to172_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs92_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs94_xor1 = (fs(((arrdiv16_mux2to173_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs93_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs94_or0 = (fs(((arrdiv16_mux2to173_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs93_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs95_xor1 = (fs(((arrdiv16_mux2to174_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs94_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs95_or0 = (fs(((arrdiv16_mux2to174_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs94_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to175_xor0 = (mux2to1(((arrdiv16_fs80_xor0 >> 0) & 0x01), ((a >> 10) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to176_xor0 = (mux2to1(((arrdiv16_fs81_xor1 >> 0) & 0x01), ((arrdiv16_mux2to160_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to177_xor0 = (mux2to1(((arrdiv16_fs82_xor1 >> 0) & 0x01), ((arrdiv16_mux2to161_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to178_xor0 = (mux2to1(((arrdiv16_fs83_xor1 >> 0) & 0x01), ((arrdiv16_mux2to162_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to179_xor0 = (mux2to1(((arrdiv16_fs84_xor1 >> 0) & 0x01), ((arrdiv16_mux2to163_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to180_xor0 = (mux2to1(((arrdiv16_fs85_xor1 >> 0) & 0x01), ((arrdiv16_mux2to164_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to181_xor0 = (mux2to1(((arrdiv16_fs86_xor1 >> 0) & 0x01), ((arrdiv16_mux2to165_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to182_xor0 = (mux2to1(((arrdiv16_fs87_xor1 >> 0) & 0x01), ((arrdiv16_mux2to166_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to183_xor0 = (mux2to1(((arrdiv16_fs88_xor1 >> 0) & 0x01), ((arrdiv16_mux2to167_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to184_xor0 = (mux2to1(((arrdiv16_fs89_xor1 >> 0) & 0x01), ((arrdiv16_mux2to168_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to185_xor0 = (mux2to1(((arrdiv16_fs90_xor1 >> 0) & 0x01), ((arrdiv16_mux2to169_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to186_xor0 = (mux2to1(((arrdiv16_fs91_xor1 >> 0) & 0x01), ((arrdiv16_mux2to170_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to187_xor0 = (mux2to1(((arrdiv16_fs92_xor1 >> 0) & 0x01), ((arrdiv16_mux2to171_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to188_xor0 = (mux2to1(((arrdiv16_fs93_xor1 >> 0) & 0x01), ((arrdiv16_mux2to172_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to189_xor0 = (mux2to1(((arrdiv16_fs94_xor1 >> 0) & 0x01), ((arrdiv16_mux2to173_xor0 >> 0) & 0x01), ((arrdiv16_fs95_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not5 = not_gate(((arrdiv16_fs95_or0 >> 0) & 0x01));
|
|
arrdiv16_fs96_xor0 = (fs(((a >> 9) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs96_and0 = (fs(((a >> 9) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs97_xor1 = (fs(((arrdiv16_mux2to175_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs96_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs97_or0 = (fs(((arrdiv16_mux2to175_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs96_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs98_xor1 = (fs(((arrdiv16_mux2to176_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs97_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs98_or0 = (fs(((arrdiv16_mux2to176_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs97_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs99_xor1 = (fs(((arrdiv16_mux2to177_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs98_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs99_or0 = (fs(((arrdiv16_mux2to177_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs98_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs100_xor1 = (fs(((arrdiv16_mux2to178_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs99_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs100_or0 = (fs(((arrdiv16_mux2to178_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs99_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs101_xor1 = (fs(((arrdiv16_mux2to179_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs100_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs101_or0 = (fs(((arrdiv16_mux2to179_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs100_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs102_xor1 = (fs(((arrdiv16_mux2to180_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs101_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs102_or0 = (fs(((arrdiv16_mux2to180_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs101_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs103_xor1 = (fs(((arrdiv16_mux2to181_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs102_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs103_or0 = (fs(((arrdiv16_mux2to181_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs102_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs104_xor1 = (fs(((arrdiv16_mux2to182_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs103_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs104_or0 = (fs(((arrdiv16_mux2to182_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs103_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs105_xor1 = (fs(((arrdiv16_mux2to183_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs104_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs105_or0 = (fs(((arrdiv16_mux2to183_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs104_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs106_xor1 = (fs(((arrdiv16_mux2to184_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs105_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs106_or0 = (fs(((arrdiv16_mux2to184_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs105_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs107_xor1 = (fs(((arrdiv16_mux2to185_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs106_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs107_or0 = (fs(((arrdiv16_mux2to185_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs106_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs108_xor1 = (fs(((arrdiv16_mux2to186_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs107_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs108_or0 = (fs(((arrdiv16_mux2to186_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs107_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs109_xor1 = (fs(((arrdiv16_mux2to187_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs108_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs109_or0 = (fs(((arrdiv16_mux2to187_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs108_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs110_xor1 = (fs(((arrdiv16_mux2to188_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs109_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs110_or0 = (fs(((arrdiv16_mux2to188_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs109_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs111_xor1 = (fs(((arrdiv16_mux2to189_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs110_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs111_or0 = (fs(((arrdiv16_mux2to189_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs110_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to190_xor0 = (mux2to1(((arrdiv16_fs96_xor0 >> 0) & 0x01), ((a >> 9) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to191_xor0 = (mux2to1(((arrdiv16_fs97_xor1 >> 0) & 0x01), ((arrdiv16_mux2to175_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to192_xor0 = (mux2to1(((arrdiv16_fs98_xor1 >> 0) & 0x01), ((arrdiv16_mux2to176_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to193_xor0 = (mux2to1(((arrdiv16_fs99_xor1 >> 0) & 0x01), ((arrdiv16_mux2to177_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to194_xor0 = (mux2to1(((arrdiv16_fs100_xor1 >> 0) & 0x01), ((arrdiv16_mux2to178_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to195_xor0 = (mux2to1(((arrdiv16_fs101_xor1 >> 0) & 0x01), ((arrdiv16_mux2to179_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to196_xor0 = (mux2to1(((arrdiv16_fs102_xor1 >> 0) & 0x01), ((arrdiv16_mux2to180_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to197_xor0 = (mux2to1(((arrdiv16_fs103_xor1 >> 0) & 0x01), ((arrdiv16_mux2to181_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to198_xor0 = (mux2to1(((arrdiv16_fs104_xor1 >> 0) & 0x01), ((arrdiv16_mux2to182_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to199_xor0 = (mux2to1(((arrdiv16_fs105_xor1 >> 0) & 0x01), ((arrdiv16_mux2to183_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1100_xor0 = (mux2to1(((arrdiv16_fs106_xor1 >> 0) & 0x01), ((arrdiv16_mux2to184_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1101_xor0 = (mux2to1(((arrdiv16_fs107_xor1 >> 0) & 0x01), ((arrdiv16_mux2to185_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1102_xor0 = (mux2to1(((arrdiv16_fs108_xor1 >> 0) & 0x01), ((arrdiv16_mux2to186_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1103_xor0 = (mux2to1(((arrdiv16_fs109_xor1 >> 0) & 0x01), ((arrdiv16_mux2to187_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1104_xor0 = (mux2to1(((arrdiv16_fs110_xor1 >> 0) & 0x01), ((arrdiv16_mux2to188_xor0 >> 0) & 0x01), ((arrdiv16_fs111_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not6 = not_gate(((arrdiv16_fs111_or0 >> 0) & 0x01));
|
|
arrdiv16_fs112_xor0 = (fs(((a >> 8) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs112_and0 = (fs(((a >> 8) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs113_xor1 = (fs(((arrdiv16_mux2to190_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs112_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs113_or0 = (fs(((arrdiv16_mux2to190_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs112_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs114_xor1 = (fs(((arrdiv16_mux2to191_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs113_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs114_or0 = (fs(((arrdiv16_mux2to191_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs113_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs115_xor1 = (fs(((arrdiv16_mux2to192_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs114_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs115_or0 = (fs(((arrdiv16_mux2to192_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs114_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs116_xor1 = (fs(((arrdiv16_mux2to193_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs115_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs116_or0 = (fs(((arrdiv16_mux2to193_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs115_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs117_xor1 = (fs(((arrdiv16_mux2to194_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs116_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs117_or0 = (fs(((arrdiv16_mux2to194_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs116_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs118_xor1 = (fs(((arrdiv16_mux2to195_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs117_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs118_or0 = (fs(((arrdiv16_mux2to195_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs117_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs119_xor1 = (fs(((arrdiv16_mux2to196_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs118_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs119_or0 = (fs(((arrdiv16_mux2to196_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs118_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs120_xor1 = (fs(((arrdiv16_mux2to197_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs119_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs120_or0 = (fs(((arrdiv16_mux2to197_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs119_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs121_xor1 = (fs(((arrdiv16_mux2to198_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs120_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs121_or0 = (fs(((arrdiv16_mux2to198_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs120_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs122_xor1 = (fs(((arrdiv16_mux2to199_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs121_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs122_or0 = (fs(((arrdiv16_mux2to199_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs121_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs123_xor1 = (fs(((arrdiv16_mux2to1100_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs122_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs123_or0 = (fs(((arrdiv16_mux2to1100_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs122_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs124_xor1 = (fs(((arrdiv16_mux2to1101_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs123_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs124_or0 = (fs(((arrdiv16_mux2to1101_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs123_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs125_xor1 = (fs(((arrdiv16_mux2to1102_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs124_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs125_or0 = (fs(((arrdiv16_mux2to1102_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs124_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs126_xor1 = (fs(((arrdiv16_mux2to1103_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs125_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs126_or0 = (fs(((arrdiv16_mux2to1103_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs125_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs127_xor1 = (fs(((arrdiv16_mux2to1104_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs126_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs127_or0 = (fs(((arrdiv16_mux2to1104_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs126_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1105_xor0 = (mux2to1(((arrdiv16_fs112_xor0 >> 0) & 0x01), ((a >> 8) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1106_xor0 = (mux2to1(((arrdiv16_fs113_xor1 >> 0) & 0x01), ((arrdiv16_mux2to190_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1107_xor0 = (mux2to1(((arrdiv16_fs114_xor1 >> 0) & 0x01), ((arrdiv16_mux2to191_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1108_xor0 = (mux2to1(((arrdiv16_fs115_xor1 >> 0) & 0x01), ((arrdiv16_mux2to192_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1109_xor0 = (mux2to1(((arrdiv16_fs116_xor1 >> 0) & 0x01), ((arrdiv16_mux2to193_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1110_xor0 = (mux2to1(((arrdiv16_fs117_xor1 >> 0) & 0x01), ((arrdiv16_mux2to194_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1111_xor0 = (mux2to1(((arrdiv16_fs118_xor1 >> 0) & 0x01), ((arrdiv16_mux2to195_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1112_xor0 = (mux2to1(((arrdiv16_fs119_xor1 >> 0) & 0x01), ((arrdiv16_mux2to196_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1113_xor0 = (mux2to1(((arrdiv16_fs120_xor1 >> 0) & 0x01), ((arrdiv16_mux2to197_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1114_xor0 = (mux2to1(((arrdiv16_fs121_xor1 >> 0) & 0x01), ((arrdiv16_mux2to198_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1115_xor0 = (mux2to1(((arrdiv16_fs122_xor1 >> 0) & 0x01), ((arrdiv16_mux2to199_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1116_xor0 = (mux2to1(((arrdiv16_fs123_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1100_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1117_xor0 = (mux2to1(((arrdiv16_fs124_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1101_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1118_xor0 = (mux2to1(((arrdiv16_fs125_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1102_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1119_xor0 = (mux2to1(((arrdiv16_fs126_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1103_xor0 >> 0) & 0x01), ((arrdiv16_fs127_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not7 = not_gate(((arrdiv16_fs127_or0 >> 0) & 0x01));
|
|
arrdiv16_fs128_xor0 = (fs(((a >> 7) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs128_and0 = (fs(((a >> 7) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs129_xor1 = (fs(((arrdiv16_mux2to1105_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs128_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs129_or0 = (fs(((arrdiv16_mux2to1105_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs128_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs130_xor1 = (fs(((arrdiv16_mux2to1106_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs129_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs130_or0 = (fs(((arrdiv16_mux2to1106_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs129_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs131_xor1 = (fs(((arrdiv16_mux2to1107_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs130_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs131_or0 = (fs(((arrdiv16_mux2to1107_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs130_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs132_xor1 = (fs(((arrdiv16_mux2to1108_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs131_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs132_or0 = (fs(((arrdiv16_mux2to1108_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs131_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs133_xor1 = (fs(((arrdiv16_mux2to1109_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs132_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs133_or0 = (fs(((arrdiv16_mux2to1109_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs132_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs134_xor1 = (fs(((arrdiv16_mux2to1110_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs133_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs134_or0 = (fs(((arrdiv16_mux2to1110_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs133_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs135_xor1 = (fs(((arrdiv16_mux2to1111_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs134_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs135_or0 = (fs(((arrdiv16_mux2to1111_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs134_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs136_xor1 = (fs(((arrdiv16_mux2to1112_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs135_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs136_or0 = (fs(((arrdiv16_mux2to1112_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs135_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs137_xor1 = (fs(((arrdiv16_mux2to1113_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs136_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs137_or0 = (fs(((arrdiv16_mux2to1113_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs136_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs138_xor1 = (fs(((arrdiv16_mux2to1114_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs137_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs138_or0 = (fs(((arrdiv16_mux2to1114_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs137_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs139_xor1 = (fs(((arrdiv16_mux2to1115_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs138_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs139_or0 = (fs(((arrdiv16_mux2to1115_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs138_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs140_xor1 = (fs(((arrdiv16_mux2to1116_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs139_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs140_or0 = (fs(((arrdiv16_mux2to1116_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs139_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs141_xor1 = (fs(((arrdiv16_mux2to1117_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs140_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs141_or0 = (fs(((arrdiv16_mux2to1117_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs140_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs142_xor1 = (fs(((arrdiv16_mux2to1118_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs141_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs142_or0 = (fs(((arrdiv16_mux2to1118_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs141_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs143_xor1 = (fs(((arrdiv16_mux2to1119_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs142_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs143_or0 = (fs(((arrdiv16_mux2to1119_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs142_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1120_xor0 = (mux2to1(((arrdiv16_fs128_xor0 >> 0) & 0x01), ((a >> 7) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1121_xor0 = (mux2to1(((arrdiv16_fs129_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1105_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1122_xor0 = (mux2to1(((arrdiv16_fs130_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1106_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1123_xor0 = (mux2to1(((arrdiv16_fs131_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1107_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1124_xor0 = (mux2to1(((arrdiv16_fs132_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1108_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1125_xor0 = (mux2to1(((arrdiv16_fs133_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1109_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1126_xor0 = (mux2to1(((arrdiv16_fs134_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1110_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1127_xor0 = (mux2to1(((arrdiv16_fs135_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1111_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1128_xor0 = (mux2to1(((arrdiv16_fs136_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1112_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1129_xor0 = (mux2to1(((arrdiv16_fs137_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1113_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1130_xor0 = (mux2to1(((arrdiv16_fs138_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1114_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1131_xor0 = (mux2to1(((arrdiv16_fs139_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1115_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1132_xor0 = (mux2to1(((arrdiv16_fs140_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1116_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1133_xor0 = (mux2to1(((arrdiv16_fs141_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1117_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1134_xor0 = (mux2to1(((arrdiv16_fs142_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1118_xor0 >> 0) & 0x01), ((arrdiv16_fs143_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not8 = not_gate(((arrdiv16_fs143_or0 >> 0) & 0x01));
|
|
arrdiv16_fs144_xor0 = (fs(((a >> 6) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs144_and0 = (fs(((a >> 6) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs145_xor1 = (fs(((arrdiv16_mux2to1120_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs144_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs145_or0 = (fs(((arrdiv16_mux2to1120_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs144_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs146_xor1 = (fs(((arrdiv16_mux2to1121_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs145_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs146_or0 = (fs(((arrdiv16_mux2to1121_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs145_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs147_xor1 = (fs(((arrdiv16_mux2to1122_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs146_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs147_or0 = (fs(((arrdiv16_mux2to1122_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs146_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs148_xor1 = (fs(((arrdiv16_mux2to1123_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs147_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs148_or0 = (fs(((arrdiv16_mux2to1123_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs147_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs149_xor1 = (fs(((arrdiv16_mux2to1124_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs148_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs149_or0 = (fs(((arrdiv16_mux2to1124_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs148_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs150_xor1 = (fs(((arrdiv16_mux2to1125_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs149_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs150_or0 = (fs(((arrdiv16_mux2to1125_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs149_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs151_xor1 = (fs(((arrdiv16_mux2to1126_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs150_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs151_or0 = (fs(((arrdiv16_mux2to1126_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs150_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs152_xor1 = (fs(((arrdiv16_mux2to1127_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs151_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs152_or0 = (fs(((arrdiv16_mux2to1127_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs151_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs153_xor1 = (fs(((arrdiv16_mux2to1128_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs152_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs153_or0 = (fs(((arrdiv16_mux2to1128_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs152_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs154_xor1 = (fs(((arrdiv16_mux2to1129_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs153_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs154_or0 = (fs(((arrdiv16_mux2to1129_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs153_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs155_xor1 = (fs(((arrdiv16_mux2to1130_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs154_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs155_or0 = (fs(((arrdiv16_mux2to1130_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs154_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs156_xor1 = (fs(((arrdiv16_mux2to1131_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs155_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs156_or0 = (fs(((arrdiv16_mux2to1131_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs155_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs157_xor1 = (fs(((arrdiv16_mux2to1132_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs156_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs157_or0 = (fs(((arrdiv16_mux2to1132_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs156_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs158_xor1 = (fs(((arrdiv16_mux2to1133_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs157_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs158_or0 = (fs(((arrdiv16_mux2to1133_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs157_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs159_xor1 = (fs(((arrdiv16_mux2to1134_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs158_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs159_or0 = (fs(((arrdiv16_mux2to1134_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs158_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1135_xor0 = (mux2to1(((arrdiv16_fs144_xor0 >> 0) & 0x01), ((a >> 6) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1136_xor0 = (mux2to1(((arrdiv16_fs145_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1120_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1137_xor0 = (mux2to1(((arrdiv16_fs146_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1121_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1138_xor0 = (mux2to1(((arrdiv16_fs147_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1122_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1139_xor0 = (mux2to1(((arrdiv16_fs148_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1123_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1140_xor0 = (mux2to1(((arrdiv16_fs149_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1124_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1141_xor0 = (mux2to1(((arrdiv16_fs150_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1125_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1142_xor0 = (mux2to1(((arrdiv16_fs151_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1126_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1143_xor0 = (mux2to1(((arrdiv16_fs152_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1127_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1144_xor0 = (mux2to1(((arrdiv16_fs153_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1128_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1145_xor0 = (mux2to1(((arrdiv16_fs154_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1129_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1146_xor0 = (mux2to1(((arrdiv16_fs155_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1130_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1147_xor0 = (mux2to1(((arrdiv16_fs156_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1131_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1148_xor0 = (mux2to1(((arrdiv16_fs157_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1132_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1149_xor0 = (mux2to1(((arrdiv16_fs158_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1133_xor0 >> 0) & 0x01), ((arrdiv16_fs159_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not9 = not_gate(((arrdiv16_fs159_or0 >> 0) & 0x01));
|
|
arrdiv16_fs160_xor0 = (fs(((a >> 5) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs160_and0 = (fs(((a >> 5) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs161_xor1 = (fs(((arrdiv16_mux2to1135_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs160_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs161_or0 = (fs(((arrdiv16_mux2to1135_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs160_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs162_xor1 = (fs(((arrdiv16_mux2to1136_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs161_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs162_or0 = (fs(((arrdiv16_mux2to1136_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs161_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs163_xor1 = (fs(((arrdiv16_mux2to1137_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs162_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs163_or0 = (fs(((arrdiv16_mux2to1137_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs162_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs164_xor1 = (fs(((arrdiv16_mux2to1138_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs163_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs164_or0 = (fs(((arrdiv16_mux2to1138_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs163_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs165_xor1 = (fs(((arrdiv16_mux2to1139_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs164_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs165_or0 = (fs(((arrdiv16_mux2to1139_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs164_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs166_xor1 = (fs(((arrdiv16_mux2to1140_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs165_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs166_or0 = (fs(((arrdiv16_mux2to1140_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs165_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs167_xor1 = (fs(((arrdiv16_mux2to1141_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs166_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs167_or0 = (fs(((arrdiv16_mux2to1141_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs166_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs168_xor1 = (fs(((arrdiv16_mux2to1142_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs167_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs168_or0 = (fs(((arrdiv16_mux2to1142_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs167_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs169_xor1 = (fs(((arrdiv16_mux2to1143_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs168_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs169_or0 = (fs(((arrdiv16_mux2to1143_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs168_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs170_xor1 = (fs(((arrdiv16_mux2to1144_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs169_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs170_or0 = (fs(((arrdiv16_mux2to1144_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs169_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs171_xor1 = (fs(((arrdiv16_mux2to1145_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs170_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs171_or0 = (fs(((arrdiv16_mux2to1145_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs170_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs172_xor1 = (fs(((arrdiv16_mux2to1146_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs171_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs172_or0 = (fs(((arrdiv16_mux2to1146_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs171_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs173_xor1 = (fs(((arrdiv16_mux2to1147_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs172_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs173_or0 = (fs(((arrdiv16_mux2to1147_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs172_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs174_xor1 = (fs(((arrdiv16_mux2to1148_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs173_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs174_or0 = (fs(((arrdiv16_mux2to1148_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs173_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs175_xor1 = (fs(((arrdiv16_mux2to1149_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs174_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs175_or0 = (fs(((arrdiv16_mux2to1149_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs174_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1150_xor0 = (mux2to1(((arrdiv16_fs160_xor0 >> 0) & 0x01), ((a >> 5) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1151_xor0 = (mux2to1(((arrdiv16_fs161_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1135_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1152_xor0 = (mux2to1(((arrdiv16_fs162_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1136_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1153_xor0 = (mux2to1(((arrdiv16_fs163_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1137_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1154_xor0 = (mux2to1(((arrdiv16_fs164_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1138_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1155_xor0 = (mux2to1(((arrdiv16_fs165_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1139_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1156_xor0 = (mux2to1(((arrdiv16_fs166_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1140_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1157_xor0 = (mux2to1(((arrdiv16_fs167_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1141_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1158_xor0 = (mux2to1(((arrdiv16_fs168_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1142_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1159_xor0 = (mux2to1(((arrdiv16_fs169_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1143_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1160_xor0 = (mux2to1(((arrdiv16_fs170_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1144_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1161_xor0 = (mux2to1(((arrdiv16_fs171_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1145_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1162_xor0 = (mux2to1(((arrdiv16_fs172_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1146_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1163_xor0 = (mux2to1(((arrdiv16_fs173_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1147_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1164_xor0 = (mux2to1(((arrdiv16_fs174_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1148_xor0 >> 0) & 0x01), ((arrdiv16_fs175_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not10 = not_gate(((arrdiv16_fs175_or0 >> 0) & 0x01));
|
|
arrdiv16_fs176_xor0 = (fs(((a >> 4) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs176_and0 = (fs(((a >> 4) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs177_xor1 = (fs(((arrdiv16_mux2to1150_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs176_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs177_or0 = (fs(((arrdiv16_mux2to1150_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs176_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs178_xor1 = (fs(((arrdiv16_mux2to1151_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs177_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs178_or0 = (fs(((arrdiv16_mux2to1151_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs177_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs179_xor1 = (fs(((arrdiv16_mux2to1152_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs178_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs179_or0 = (fs(((arrdiv16_mux2to1152_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs178_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs180_xor1 = (fs(((arrdiv16_mux2to1153_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs179_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs180_or0 = (fs(((arrdiv16_mux2to1153_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs179_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs181_xor1 = (fs(((arrdiv16_mux2to1154_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs180_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs181_or0 = (fs(((arrdiv16_mux2to1154_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs180_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs182_xor1 = (fs(((arrdiv16_mux2to1155_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs181_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs182_or0 = (fs(((arrdiv16_mux2to1155_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs181_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs183_xor1 = (fs(((arrdiv16_mux2to1156_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs182_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs183_or0 = (fs(((arrdiv16_mux2to1156_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs182_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs184_xor1 = (fs(((arrdiv16_mux2to1157_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs183_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs184_or0 = (fs(((arrdiv16_mux2to1157_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs183_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs185_xor1 = (fs(((arrdiv16_mux2to1158_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs184_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs185_or0 = (fs(((arrdiv16_mux2to1158_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs184_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs186_xor1 = (fs(((arrdiv16_mux2to1159_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs185_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs186_or0 = (fs(((arrdiv16_mux2to1159_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs185_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs187_xor1 = (fs(((arrdiv16_mux2to1160_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs186_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs187_or0 = (fs(((arrdiv16_mux2to1160_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs186_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs188_xor1 = (fs(((arrdiv16_mux2to1161_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs187_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs188_or0 = (fs(((arrdiv16_mux2to1161_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs187_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs189_xor1 = (fs(((arrdiv16_mux2to1162_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs188_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs189_or0 = (fs(((arrdiv16_mux2to1162_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs188_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs190_xor1 = (fs(((arrdiv16_mux2to1163_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs189_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs190_or0 = (fs(((arrdiv16_mux2to1163_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs189_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs191_xor1 = (fs(((arrdiv16_mux2to1164_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs190_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs191_or0 = (fs(((arrdiv16_mux2to1164_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs190_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1165_xor0 = (mux2to1(((arrdiv16_fs176_xor0 >> 0) & 0x01), ((a >> 4) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1166_xor0 = (mux2to1(((arrdiv16_fs177_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1150_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1167_xor0 = (mux2to1(((arrdiv16_fs178_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1151_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1168_xor0 = (mux2to1(((arrdiv16_fs179_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1152_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1169_xor0 = (mux2to1(((arrdiv16_fs180_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1153_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1170_xor0 = (mux2to1(((arrdiv16_fs181_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1154_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1171_xor0 = (mux2to1(((arrdiv16_fs182_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1155_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1172_xor0 = (mux2to1(((arrdiv16_fs183_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1156_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1173_xor0 = (mux2to1(((arrdiv16_fs184_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1157_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1174_xor0 = (mux2to1(((arrdiv16_fs185_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1158_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1175_xor0 = (mux2to1(((arrdiv16_fs186_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1159_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1176_xor0 = (mux2to1(((arrdiv16_fs187_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1160_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1177_xor0 = (mux2to1(((arrdiv16_fs188_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1161_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1178_xor0 = (mux2to1(((arrdiv16_fs189_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1162_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1179_xor0 = (mux2to1(((arrdiv16_fs190_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1163_xor0 >> 0) & 0x01), ((arrdiv16_fs191_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not11 = not_gate(((arrdiv16_fs191_or0 >> 0) & 0x01));
|
|
arrdiv16_fs192_xor0 = (fs(((a >> 3) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs192_and0 = (fs(((a >> 3) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs193_xor1 = (fs(((arrdiv16_mux2to1165_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs192_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs193_or0 = (fs(((arrdiv16_mux2to1165_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs192_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs194_xor1 = (fs(((arrdiv16_mux2to1166_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs193_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs194_or0 = (fs(((arrdiv16_mux2to1166_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs193_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs195_xor1 = (fs(((arrdiv16_mux2to1167_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs194_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs195_or0 = (fs(((arrdiv16_mux2to1167_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs194_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs196_xor1 = (fs(((arrdiv16_mux2to1168_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs195_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs196_or0 = (fs(((arrdiv16_mux2to1168_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs195_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs197_xor1 = (fs(((arrdiv16_mux2to1169_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs196_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs197_or0 = (fs(((arrdiv16_mux2to1169_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs196_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs198_xor1 = (fs(((arrdiv16_mux2to1170_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs197_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs198_or0 = (fs(((arrdiv16_mux2to1170_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs197_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs199_xor1 = (fs(((arrdiv16_mux2to1171_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs198_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs199_or0 = (fs(((arrdiv16_mux2to1171_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs198_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs200_xor1 = (fs(((arrdiv16_mux2to1172_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs199_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs200_or0 = (fs(((arrdiv16_mux2to1172_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs199_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs201_xor1 = (fs(((arrdiv16_mux2to1173_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs200_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs201_or0 = (fs(((arrdiv16_mux2to1173_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs200_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs202_xor1 = (fs(((arrdiv16_mux2to1174_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs201_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs202_or0 = (fs(((arrdiv16_mux2to1174_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs201_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs203_xor1 = (fs(((arrdiv16_mux2to1175_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs202_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs203_or0 = (fs(((arrdiv16_mux2to1175_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs202_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs204_xor1 = (fs(((arrdiv16_mux2to1176_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs203_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs204_or0 = (fs(((arrdiv16_mux2to1176_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs203_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs205_xor1 = (fs(((arrdiv16_mux2to1177_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs204_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs205_or0 = (fs(((arrdiv16_mux2to1177_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs204_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs206_xor1 = (fs(((arrdiv16_mux2to1178_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs205_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs206_or0 = (fs(((arrdiv16_mux2to1178_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs205_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs207_xor1 = (fs(((arrdiv16_mux2to1179_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs206_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs207_or0 = (fs(((arrdiv16_mux2to1179_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs206_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1180_xor0 = (mux2to1(((arrdiv16_fs192_xor0 >> 0) & 0x01), ((a >> 3) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1181_xor0 = (mux2to1(((arrdiv16_fs193_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1165_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1182_xor0 = (mux2to1(((arrdiv16_fs194_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1166_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1183_xor0 = (mux2to1(((arrdiv16_fs195_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1167_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1184_xor0 = (mux2to1(((arrdiv16_fs196_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1168_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1185_xor0 = (mux2to1(((arrdiv16_fs197_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1169_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1186_xor0 = (mux2to1(((arrdiv16_fs198_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1170_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1187_xor0 = (mux2to1(((arrdiv16_fs199_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1171_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1188_xor0 = (mux2to1(((arrdiv16_fs200_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1172_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1189_xor0 = (mux2to1(((arrdiv16_fs201_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1173_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1190_xor0 = (mux2to1(((arrdiv16_fs202_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1174_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1191_xor0 = (mux2to1(((arrdiv16_fs203_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1175_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1192_xor0 = (mux2to1(((arrdiv16_fs204_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1176_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1193_xor0 = (mux2to1(((arrdiv16_fs205_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1177_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1194_xor0 = (mux2to1(((arrdiv16_fs206_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1178_xor0 >> 0) & 0x01), ((arrdiv16_fs207_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not12 = not_gate(((arrdiv16_fs207_or0 >> 0) & 0x01));
|
|
arrdiv16_fs208_xor0 = (fs(((a >> 2) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs208_and0 = (fs(((a >> 2) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs209_xor1 = (fs(((arrdiv16_mux2to1180_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs208_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs209_or0 = (fs(((arrdiv16_mux2to1180_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs208_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs210_xor1 = (fs(((arrdiv16_mux2to1181_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs209_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs210_or0 = (fs(((arrdiv16_mux2to1181_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs209_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs211_xor1 = (fs(((arrdiv16_mux2to1182_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs210_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs211_or0 = (fs(((arrdiv16_mux2to1182_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs210_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs212_xor1 = (fs(((arrdiv16_mux2to1183_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs211_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs212_or0 = (fs(((arrdiv16_mux2to1183_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs211_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs213_xor1 = (fs(((arrdiv16_mux2to1184_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs212_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs213_or0 = (fs(((arrdiv16_mux2to1184_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs212_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs214_xor1 = (fs(((arrdiv16_mux2to1185_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs213_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs214_or0 = (fs(((arrdiv16_mux2to1185_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs213_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs215_xor1 = (fs(((arrdiv16_mux2to1186_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs214_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs215_or0 = (fs(((arrdiv16_mux2to1186_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs214_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs216_xor1 = (fs(((arrdiv16_mux2to1187_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs215_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs216_or0 = (fs(((arrdiv16_mux2to1187_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs215_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs217_xor1 = (fs(((arrdiv16_mux2to1188_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs216_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs217_or0 = (fs(((arrdiv16_mux2to1188_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs216_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs218_xor1 = (fs(((arrdiv16_mux2to1189_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs217_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs218_or0 = (fs(((arrdiv16_mux2to1189_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs217_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs219_xor1 = (fs(((arrdiv16_mux2to1190_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs218_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs219_or0 = (fs(((arrdiv16_mux2to1190_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs218_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs220_xor1 = (fs(((arrdiv16_mux2to1191_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs219_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs220_or0 = (fs(((arrdiv16_mux2to1191_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs219_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs221_xor1 = (fs(((arrdiv16_mux2to1192_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs220_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs221_or0 = (fs(((arrdiv16_mux2to1192_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs220_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs222_xor1 = (fs(((arrdiv16_mux2to1193_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs221_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs222_or0 = (fs(((arrdiv16_mux2to1193_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs221_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs223_xor1 = (fs(((arrdiv16_mux2to1194_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs222_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs223_or0 = (fs(((arrdiv16_mux2to1194_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs222_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1195_xor0 = (mux2to1(((arrdiv16_fs208_xor0 >> 0) & 0x01), ((a >> 2) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1196_xor0 = (mux2to1(((arrdiv16_fs209_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1180_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1197_xor0 = (mux2to1(((arrdiv16_fs210_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1181_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1198_xor0 = (mux2to1(((arrdiv16_fs211_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1182_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1199_xor0 = (mux2to1(((arrdiv16_fs212_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1183_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1200_xor0 = (mux2to1(((arrdiv16_fs213_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1184_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1201_xor0 = (mux2to1(((arrdiv16_fs214_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1185_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1202_xor0 = (mux2to1(((arrdiv16_fs215_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1186_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1203_xor0 = (mux2to1(((arrdiv16_fs216_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1187_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1204_xor0 = (mux2to1(((arrdiv16_fs217_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1188_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1205_xor0 = (mux2to1(((arrdiv16_fs218_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1189_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1206_xor0 = (mux2to1(((arrdiv16_fs219_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1190_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1207_xor0 = (mux2to1(((arrdiv16_fs220_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1191_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1208_xor0 = (mux2to1(((arrdiv16_fs221_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1192_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1209_xor0 = (mux2to1(((arrdiv16_fs222_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1193_xor0 >> 0) & 0x01), ((arrdiv16_fs223_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not13 = not_gate(((arrdiv16_fs223_or0 >> 0) & 0x01));
|
|
arrdiv16_fs224_xor0 = (fs(((a >> 1) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs224_and0 = (fs(((a >> 1) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs225_xor1 = (fs(((arrdiv16_mux2to1195_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs224_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs225_or0 = (fs(((arrdiv16_mux2to1195_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs224_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs226_xor1 = (fs(((arrdiv16_mux2to1196_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs225_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs226_or0 = (fs(((arrdiv16_mux2to1196_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs225_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs227_xor1 = (fs(((arrdiv16_mux2to1197_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs226_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs227_or0 = (fs(((arrdiv16_mux2to1197_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs226_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs228_xor1 = (fs(((arrdiv16_mux2to1198_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs227_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs228_or0 = (fs(((arrdiv16_mux2to1198_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs227_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs229_xor1 = (fs(((arrdiv16_mux2to1199_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs228_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs229_or0 = (fs(((arrdiv16_mux2to1199_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs228_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs230_xor1 = (fs(((arrdiv16_mux2to1200_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs229_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs230_or0 = (fs(((arrdiv16_mux2to1200_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs229_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs231_xor1 = (fs(((arrdiv16_mux2to1201_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs230_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs231_or0 = (fs(((arrdiv16_mux2to1201_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs230_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs232_xor1 = (fs(((arrdiv16_mux2to1202_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs231_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs232_or0 = (fs(((arrdiv16_mux2to1202_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs231_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs233_xor1 = (fs(((arrdiv16_mux2to1203_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs232_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs233_or0 = (fs(((arrdiv16_mux2to1203_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs232_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs234_xor1 = (fs(((arrdiv16_mux2to1204_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs233_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs234_or0 = (fs(((arrdiv16_mux2to1204_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs233_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs235_xor1 = (fs(((arrdiv16_mux2to1205_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs234_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs235_or0 = (fs(((arrdiv16_mux2to1205_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs234_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs236_xor1 = (fs(((arrdiv16_mux2to1206_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs235_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs236_or0 = (fs(((arrdiv16_mux2to1206_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs235_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs237_xor1 = (fs(((arrdiv16_mux2to1207_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs236_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs237_or0 = (fs(((arrdiv16_mux2to1207_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs236_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs238_xor1 = (fs(((arrdiv16_mux2to1208_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs237_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs238_or0 = (fs(((arrdiv16_mux2to1208_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs237_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs239_xor1 = (fs(((arrdiv16_mux2to1209_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs238_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs239_or0 = (fs(((arrdiv16_mux2to1209_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs238_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_mux2to1210_xor0 = (mux2to1(((arrdiv16_fs224_xor0 >> 0) & 0x01), ((a >> 1) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1211_xor0 = (mux2to1(((arrdiv16_fs225_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1195_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1212_xor0 = (mux2to1(((arrdiv16_fs226_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1196_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1213_xor0 = (mux2to1(((arrdiv16_fs227_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1197_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1214_xor0 = (mux2to1(((arrdiv16_fs228_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1198_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1215_xor0 = (mux2to1(((arrdiv16_fs229_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1199_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1216_xor0 = (mux2to1(((arrdiv16_fs230_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1200_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1217_xor0 = (mux2to1(((arrdiv16_fs231_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1201_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1218_xor0 = (mux2to1(((arrdiv16_fs232_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1202_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1219_xor0 = (mux2to1(((arrdiv16_fs233_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1203_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1220_xor0 = (mux2to1(((arrdiv16_fs234_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1204_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1221_xor0 = (mux2to1(((arrdiv16_fs235_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1205_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1222_xor0 = (mux2to1(((arrdiv16_fs236_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1206_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1223_xor0 = (mux2to1(((arrdiv16_fs237_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1207_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_mux2to1224_xor0 = (mux2to1(((arrdiv16_fs238_xor1 >> 0) & 0x01), ((arrdiv16_mux2to1208_xor0 >> 0) & 0x01), ((arrdiv16_fs239_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_not14 = not_gate(((arrdiv16_fs239_or0 >> 0) & 0x01));
|
|
arrdiv16_fs240_xor0 = (fs(((a >> 0) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 0) & 0x01;
|
|
arrdiv16_fs240_and0 = (fs(((a >> 0) & 0x01), ((b >> 0) & 0x01), (0x00)) >> 1) & 0x01;
|
|
arrdiv16_fs241_xor1 = (fs(((arrdiv16_mux2to1210_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs240_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs241_or0 = (fs(((arrdiv16_mux2to1210_xor0 >> 0) & 0x01), ((b >> 1) & 0x01), ((arrdiv16_fs240_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs242_xor1 = (fs(((arrdiv16_mux2to1211_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs241_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs242_or0 = (fs(((arrdiv16_mux2to1211_xor0 >> 0) & 0x01), ((b >> 2) & 0x01), ((arrdiv16_fs241_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs243_xor1 = (fs(((arrdiv16_mux2to1212_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs242_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs243_or0 = (fs(((arrdiv16_mux2to1212_xor0 >> 0) & 0x01), ((b >> 3) & 0x01), ((arrdiv16_fs242_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs244_xor1 = (fs(((arrdiv16_mux2to1213_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs243_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs244_or0 = (fs(((arrdiv16_mux2to1213_xor0 >> 0) & 0x01), ((b >> 4) & 0x01), ((arrdiv16_fs243_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs245_xor1 = (fs(((arrdiv16_mux2to1214_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs244_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs245_or0 = (fs(((arrdiv16_mux2to1214_xor0 >> 0) & 0x01), ((b >> 5) & 0x01), ((arrdiv16_fs244_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs246_xor1 = (fs(((arrdiv16_mux2to1215_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs245_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs246_or0 = (fs(((arrdiv16_mux2to1215_xor0 >> 0) & 0x01), ((b >> 6) & 0x01), ((arrdiv16_fs245_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs247_xor1 = (fs(((arrdiv16_mux2to1216_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs246_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs247_or0 = (fs(((arrdiv16_mux2to1216_xor0 >> 0) & 0x01), ((b >> 7) & 0x01), ((arrdiv16_fs246_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs248_xor1 = (fs(((arrdiv16_mux2to1217_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs247_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs248_or0 = (fs(((arrdiv16_mux2to1217_xor0 >> 0) & 0x01), ((b >> 8) & 0x01), ((arrdiv16_fs247_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs249_xor1 = (fs(((arrdiv16_mux2to1218_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs248_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs249_or0 = (fs(((arrdiv16_mux2to1218_xor0 >> 0) & 0x01), ((b >> 9) & 0x01), ((arrdiv16_fs248_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs250_xor1 = (fs(((arrdiv16_mux2to1219_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs249_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs250_or0 = (fs(((arrdiv16_mux2to1219_xor0 >> 0) & 0x01), ((b >> 10) & 0x01), ((arrdiv16_fs249_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs251_xor1 = (fs(((arrdiv16_mux2to1220_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs250_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs251_or0 = (fs(((arrdiv16_mux2to1220_xor0 >> 0) & 0x01), ((b >> 11) & 0x01), ((arrdiv16_fs250_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs252_xor1 = (fs(((arrdiv16_mux2to1221_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs251_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs252_or0 = (fs(((arrdiv16_mux2to1221_xor0 >> 0) & 0x01), ((b >> 12) & 0x01), ((arrdiv16_fs251_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs253_xor1 = (fs(((arrdiv16_mux2to1222_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs252_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs253_or0 = (fs(((arrdiv16_mux2to1222_xor0 >> 0) & 0x01), ((b >> 13) & 0x01), ((arrdiv16_fs252_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs254_xor1 = (fs(((arrdiv16_mux2to1223_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs253_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs254_or0 = (fs(((arrdiv16_mux2to1223_xor0 >> 0) & 0x01), ((b >> 14) & 0x01), ((arrdiv16_fs253_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_fs255_xor1 = (fs(((arrdiv16_mux2to1224_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs254_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
arrdiv16_fs255_or0 = (fs(((arrdiv16_mux2to1224_xor0 >> 0) & 0x01), ((b >> 15) & 0x01), ((arrdiv16_fs254_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
arrdiv16_not15 = not_gate(((arrdiv16_fs255_or0 >> 0) & 0x01));
|
|
|
|
arrdiv16_out |= ((arrdiv16_not15 >> 0) & 0x01ull) << 0;
|
|
arrdiv16_out |= ((arrdiv16_not14 >> 0) & 0x01ull) << 1;
|
|
arrdiv16_out |= ((arrdiv16_not13 >> 0) & 0x01ull) << 2;
|
|
arrdiv16_out |= ((arrdiv16_not12 >> 0) & 0x01ull) << 3;
|
|
arrdiv16_out |= ((arrdiv16_not11 >> 0) & 0x01ull) << 4;
|
|
arrdiv16_out |= ((arrdiv16_not10 >> 0) & 0x01ull) << 5;
|
|
arrdiv16_out |= ((arrdiv16_not9 >> 0) & 0x01ull) << 6;
|
|
arrdiv16_out |= ((arrdiv16_not8 >> 0) & 0x01ull) << 7;
|
|
arrdiv16_out |= ((arrdiv16_not7 >> 0) & 0x01ull) << 8;
|
|
arrdiv16_out |= ((arrdiv16_not6 >> 0) & 0x01ull) << 9;
|
|
arrdiv16_out |= ((arrdiv16_not5 >> 0) & 0x01ull) << 10;
|
|
arrdiv16_out |= ((arrdiv16_not4 >> 0) & 0x01ull) << 11;
|
|
arrdiv16_out |= ((arrdiv16_not3 >> 0) & 0x01ull) << 12;
|
|
arrdiv16_out |= ((arrdiv16_not2 >> 0) & 0x01ull) << 13;
|
|
arrdiv16_out |= ((arrdiv16_not1 >> 0) & 0x01ull) << 14;
|
|
arrdiv16_out |= ((arrdiv16_not0 >> 0) & 0x01ull) << 15;
|
|
return arrdiv16_out;
|
|
} |