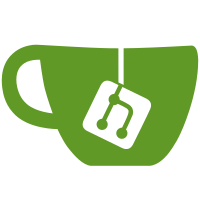
* #10 CGP Circuits as inputs (#11) * CGP Circuits as inputs * #10 support of signed output in general circuit * input as output works * output connected to input (c) * automated verilog testing * output rename * Implemented CSA and Wallace tree multiplier composing of CSAs. Also did some code cleanup. * Typos fix and code cleanup. * Added new (approximate) multiplier architectures and did some minor changes regarding sign extension for c output formats. * Updated automated testing scripts. * Small bugfix in python code generation (I initially thought this line is useless). * Updated generated circuits folder. Co-authored-by: Vojta Mrazek <mrazek@fit.vutbr.cz>
1621 lines
134 KiB
C
1621 lines
134 KiB
C
#include <stdio.h>
|
|
#include <stdint.h>
|
|
|
|
uint8_t and_gate(uint8_t a, uint8_t b){
|
|
return ((a >> 0) & 0x01) & ((b >> 0) & 0x01);
|
|
}
|
|
|
|
uint8_t xor_gate(uint8_t a, uint8_t b){
|
|
return ((a >> 0) & 0x01) ^ ((b >> 0) & 0x01);
|
|
}
|
|
|
|
uint8_t or_gate(uint8_t a, uint8_t b){
|
|
return ((a >> 0) & 0x01) | ((b >> 0) & 0x01);
|
|
}
|
|
|
|
uint8_t ha(uint8_t a, uint8_t b){
|
|
uint8_t ha_out = 0;
|
|
uint8_t ha_xor0 = 0;
|
|
uint8_t ha_and0 = 0;
|
|
|
|
ha_xor0 = xor_gate(((a >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
ha_and0 = and_gate(((a >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
|
|
ha_out |= ((ha_xor0 >> 0) & 0x01ull) << 0;
|
|
ha_out |= ((ha_and0 >> 0) & 0x01ull) << 1;
|
|
return ha_out;
|
|
}
|
|
|
|
uint8_t fa(uint8_t a, uint8_t b, uint8_t cin){
|
|
uint8_t fa_out = 0;
|
|
uint8_t fa_xor0 = 0;
|
|
uint8_t fa_and0 = 0;
|
|
uint8_t fa_xor1 = 0;
|
|
uint8_t fa_and1 = 0;
|
|
uint8_t fa_or0 = 0;
|
|
|
|
fa_xor0 = xor_gate(((a >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
fa_and0 = and_gate(((a >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
fa_xor1 = xor_gate(((fa_xor0 >> 0) & 0x01), ((cin >> 0) & 0x01));
|
|
fa_and1 = and_gate(((fa_xor0 >> 0) & 0x01), ((cin >> 0) & 0x01));
|
|
fa_or0 = or_gate(((fa_and0 >> 0) & 0x01), ((fa_and1 >> 0) & 0x01));
|
|
|
|
fa_out |= ((fa_xor1 >> 0) & 0x01ull) << 0;
|
|
fa_out |= ((fa_or0 >> 0) & 0x01ull) << 1;
|
|
return fa_out;
|
|
}
|
|
|
|
uint64_t u_rca16(uint64_t a, uint64_t b){
|
|
uint64_t u_rca16_out = 0;
|
|
uint8_t u_rca16_ha_xor0 = 0;
|
|
uint8_t u_rca16_ha_and0 = 0;
|
|
uint8_t u_rca16_fa1_xor1 = 0;
|
|
uint8_t u_rca16_fa1_or0 = 0;
|
|
uint8_t u_rca16_fa2_xor1 = 0;
|
|
uint8_t u_rca16_fa2_or0 = 0;
|
|
uint8_t u_rca16_fa3_xor1 = 0;
|
|
uint8_t u_rca16_fa3_or0 = 0;
|
|
uint8_t u_rca16_fa4_xor1 = 0;
|
|
uint8_t u_rca16_fa4_or0 = 0;
|
|
uint8_t u_rca16_fa5_xor1 = 0;
|
|
uint8_t u_rca16_fa5_or0 = 0;
|
|
uint8_t u_rca16_fa6_xor1 = 0;
|
|
uint8_t u_rca16_fa6_or0 = 0;
|
|
uint8_t u_rca16_fa7_xor1 = 0;
|
|
uint8_t u_rca16_fa7_or0 = 0;
|
|
uint8_t u_rca16_fa8_xor1 = 0;
|
|
uint8_t u_rca16_fa8_or0 = 0;
|
|
uint8_t u_rca16_fa9_xor1 = 0;
|
|
uint8_t u_rca16_fa9_or0 = 0;
|
|
uint8_t u_rca16_fa10_xor1 = 0;
|
|
uint8_t u_rca16_fa10_or0 = 0;
|
|
uint8_t u_rca16_fa11_xor1 = 0;
|
|
uint8_t u_rca16_fa11_or0 = 0;
|
|
uint8_t u_rca16_fa12_xor1 = 0;
|
|
uint8_t u_rca16_fa12_or0 = 0;
|
|
uint8_t u_rca16_fa13_xor1 = 0;
|
|
uint8_t u_rca16_fa13_or0 = 0;
|
|
uint8_t u_rca16_fa14_xor1 = 0;
|
|
uint8_t u_rca16_fa14_or0 = 0;
|
|
uint8_t u_rca16_fa15_xor1 = 0;
|
|
uint8_t u_rca16_fa15_or0 = 0;
|
|
|
|
u_rca16_ha_xor0 = (ha(((a >> 0) & 0x01), ((b >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_ha_and0 = (ha(((a >> 0) & 0x01), ((b >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa1_xor1 = (fa(((a >> 1) & 0x01), ((b >> 1) & 0x01), ((u_rca16_ha_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa1_or0 = (fa(((a >> 1) & 0x01), ((b >> 1) & 0x01), ((u_rca16_ha_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa2_xor1 = (fa(((a >> 2) & 0x01), ((b >> 2) & 0x01), ((u_rca16_fa1_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa2_or0 = (fa(((a >> 2) & 0x01), ((b >> 2) & 0x01), ((u_rca16_fa1_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa3_xor1 = (fa(((a >> 3) & 0x01), ((b >> 3) & 0x01), ((u_rca16_fa2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa3_or0 = (fa(((a >> 3) & 0x01), ((b >> 3) & 0x01), ((u_rca16_fa2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa4_xor1 = (fa(((a >> 4) & 0x01), ((b >> 4) & 0x01), ((u_rca16_fa3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa4_or0 = (fa(((a >> 4) & 0x01), ((b >> 4) & 0x01), ((u_rca16_fa3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa5_xor1 = (fa(((a >> 5) & 0x01), ((b >> 5) & 0x01), ((u_rca16_fa4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa5_or0 = (fa(((a >> 5) & 0x01), ((b >> 5) & 0x01), ((u_rca16_fa4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa6_xor1 = (fa(((a >> 6) & 0x01), ((b >> 6) & 0x01), ((u_rca16_fa5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa6_or0 = (fa(((a >> 6) & 0x01), ((b >> 6) & 0x01), ((u_rca16_fa5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa7_xor1 = (fa(((a >> 7) & 0x01), ((b >> 7) & 0x01), ((u_rca16_fa6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa7_or0 = (fa(((a >> 7) & 0x01), ((b >> 7) & 0x01), ((u_rca16_fa6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa8_xor1 = (fa(((a >> 8) & 0x01), ((b >> 8) & 0x01), ((u_rca16_fa7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa8_or0 = (fa(((a >> 8) & 0x01), ((b >> 8) & 0x01), ((u_rca16_fa7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa9_xor1 = (fa(((a >> 9) & 0x01), ((b >> 9) & 0x01), ((u_rca16_fa8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa9_or0 = (fa(((a >> 9) & 0x01), ((b >> 9) & 0x01), ((u_rca16_fa8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa10_xor1 = (fa(((a >> 10) & 0x01), ((b >> 10) & 0x01), ((u_rca16_fa9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa10_or0 = (fa(((a >> 10) & 0x01), ((b >> 10) & 0x01), ((u_rca16_fa9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa11_xor1 = (fa(((a >> 11) & 0x01), ((b >> 11) & 0x01), ((u_rca16_fa10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa11_or0 = (fa(((a >> 11) & 0x01), ((b >> 11) & 0x01), ((u_rca16_fa10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa12_xor1 = (fa(((a >> 12) & 0x01), ((b >> 12) & 0x01), ((u_rca16_fa11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa12_or0 = (fa(((a >> 12) & 0x01), ((b >> 12) & 0x01), ((u_rca16_fa11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa13_xor1 = (fa(((a >> 13) & 0x01), ((b >> 13) & 0x01), ((u_rca16_fa12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa13_or0 = (fa(((a >> 13) & 0x01), ((b >> 13) & 0x01), ((u_rca16_fa12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa14_xor1 = (fa(((a >> 14) & 0x01), ((b >> 14) & 0x01), ((u_rca16_fa13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa14_or0 = (fa(((a >> 14) & 0x01), ((b >> 14) & 0x01), ((u_rca16_fa13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_rca16_fa15_xor1 = (fa(((a >> 15) & 0x01), ((b >> 15) & 0x01), ((u_rca16_fa14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_rca16_fa15_or0 = (fa(((a >> 15) & 0x01), ((b >> 15) & 0x01), ((u_rca16_fa14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
|
|
u_rca16_out |= ((u_rca16_ha_xor0 >> 0) & 0x01ull) << 0;
|
|
u_rca16_out |= ((u_rca16_fa1_xor1 >> 0) & 0x01ull) << 1;
|
|
u_rca16_out |= ((u_rca16_fa2_xor1 >> 0) & 0x01ull) << 2;
|
|
u_rca16_out |= ((u_rca16_fa3_xor1 >> 0) & 0x01ull) << 3;
|
|
u_rca16_out |= ((u_rca16_fa4_xor1 >> 0) & 0x01ull) << 4;
|
|
u_rca16_out |= ((u_rca16_fa5_xor1 >> 0) & 0x01ull) << 5;
|
|
u_rca16_out |= ((u_rca16_fa6_xor1 >> 0) & 0x01ull) << 6;
|
|
u_rca16_out |= ((u_rca16_fa7_xor1 >> 0) & 0x01ull) << 7;
|
|
u_rca16_out |= ((u_rca16_fa8_xor1 >> 0) & 0x01ull) << 8;
|
|
u_rca16_out |= ((u_rca16_fa9_xor1 >> 0) & 0x01ull) << 9;
|
|
u_rca16_out |= ((u_rca16_fa10_xor1 >> 0) & 0x01ull) << 10;
|
|
u_rca16_out |= ((u_rca16_fa11_xor1 >> 0) & 0x01ull) << 11;
|
|
u_rca16_out |= ((u_rca16_fa12_xor1 >> 0) & 0x01ull) << 12;
|
|
u_rca16_out |= ((u_rca16_fa13_xor1 >> 0) & 0x01ull) << 13;
|
|
u_rca16_out |= ((u_rca16_fa14_xor1 >> 0) & 0x01ull) << 14;
|
|
u_rca16_out |= ((u_rca16_fa15_xor1 >> 0) & 0x01ull) << 15;
|
|
u_rca16_out |= ((u_rca16_fa15_or0 >> 0) & 0x01ull) << 16;
|
|
return u_rca16_out;
|
|
}
|
|
|
|
uint64_t u_csamul_rca16(uint64_t a, uint64_t b){
|
|
uint64_t u_csamul_rca16_out = 0;
|
|
uint8_t u_csamul_rca16_and0_0 = 0;
|
|
uint8_t u_csamul_rca16_and1_0 = 0;
|
|
uint8_t u_csamul_rca16_and2_0 = 0;
|
|
uint8_t u_csamul_rca16_and3_0 = 0;
|
|
uint8_t u_csamul_rca16_and4_0 = 0;
|
|
uint8_t u_csamul_rca16_and5_0 = 0;
|
|
uint8_t u_csamul_rca16_and6_0 = 0;
|
|
uint8_t u_csamul_rca16_and7_0 = 0;
|
|
uint8_t u_csamul_rca16_and8_0 = 0;
|
|
uint8_t u_csamul_rca16_and9_0 = 0;
|
|
uint8_t u_csamul_rca16_and10_0 = 0;
|
|
uint8_t u_csamul_rca16_and11_0 = 0;
|
|
uint8_t u_csamul_rca16_and12_0 = 0;
|
|
uint8_t u_csamul_rca16_and13_0 = 0;
|
|
uint8_t u_csamul_rca16_and14_0 = 0;
|
|
uint8_t u_csamul_rca16_and15_0 = 0;
|
|
uint8_t u_csamul_rca16_and0_1 = 0;
|
|
uint8_t u_csamul_rca16_ha0_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha0_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and1_1 = 0;
|
|
uint8_t u_csamul_rca16_ha1_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha1_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and2_1 = 0;
|
|
uint8_t u_csamul_rca16_ha2_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha2_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and3_1 = 0;
|
|
uint8_t u_csamul_rca16_ha3_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha3_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and4_1 = 0;
|
|
uint8_t u_csamul_rca16_ha4_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha4_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and5_1 = 0;
|
|
uint8_t u_csamul_rca16_ha5_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha5_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and6_1 = 0;
|
|
uint8_t u_csamul_rca16_ha6_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha6_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and7_1 = 0;
|
|
uint8_t u_csamul_rca16_ha7_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha7_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and8_1 = 0;
|
|
uint8_t u_csamul_rca16_ha8_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha8_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and9_1 = 0;
|
|
uint8_t u_csamul_rca16_ha9_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha9_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and10_1 = 0;
|
|
uint8_t u_csamul_rca16_ha10_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha10_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and11_1 = 0;
|
|
uint8_t u_csamul_rca16_ha11_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha11_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and12_1 = 0;
|
|
uint8_t u_csamul_rca16_ha12_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha12_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and13_1 = 0;
|
|
uint8_t u_csamul_rca16_ha13_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha13_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and14_1 = 0;
|
|
uint8_t u_csamul_rca16_ha14_1_xor0 = 0;
|
|
uint8_t u_csamul_rca16_ha14_1_and0 = 0;
|
|
uint8_t u_csamul_rca16_and15_1 = 0;
|
|
uint8_t u_csamul_rca16_and0_2 = 0;
|
|
uint8_t u_csamul_rca16_fa0_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_2 = 0;
|
|
uint8_t u_csamul_rca16_fa1_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_2 = 0;
|
|
uint8_t u_csamul_rca16_fa2_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_2 = 0;
|
|
uint8_t u_csamul_rca16_fa3_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_2 = 0;
|
|
uint8_t u_csamul_rca16_fa4_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_2 = 0;
|
|
uint8_t u_csamul_rca16_fa5_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_2 = 0;
|
|
uint8_t u_csamul_rca16_fa6_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_2 = 0;
|
|
uint8_t u_csamul_rca16_fa7_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_2 = 0;
|
|
uint8_t u_csamul_rca16_fa8_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_2 = 0;
|
|
uint8_t u_csamul_rca16_fa9_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_2 = 0;
|
|
uint8_t u_csamul_rca16_fa10_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_2 = 0;
|
|
uint8_t u_csamul_rca16_fa11_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_2 = 0;
|
|
uint8_t u_csamul_rca16_fa12_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_2 = 0;
|
|
uint8_t u_csamul_rca16_fa13_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_2 = 0;
|
|
uint8_t u_csamul_rca16_fa14_2_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_2_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_2 = 0;
|
|
uint8_t u_csamul_rca16_and0_3 = 0;
|
|
uint8_t u_csamul_rca16_fa0_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_3 = 0;
|
|
uint8_t u_csamul_rca16_fa1_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_3 = 0;
|
|
uint8_t u_csamul_rca16_fa2_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_3 = 0;
|
|
uint8_t u_csamul_rca16_fa3_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_3 = 0;
|
|
uint8_t u_csamul_rca16_fa4_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_3 = 0;
|
|
uint8_t u_csamul_rca16_fa5_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_3 = 0;
|
|
uint8_t u_csamul_rca16_fa6_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_3 = 0;
|
|
uint8_t u_csamul_rca16_fa7_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_3 = 0;
|
|
uint8_t u_csamul_rca16_fa8_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_3 = 0;
|
|
uint8_t u_csamul_rca16_fa9_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_3 = 0;
|
|
uint8_t u_csamul_rca16_fa10_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_3 = 0;
|
|
uint8_t u_csamul_rca16_fa11_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_3 = 0;
|
|
uint8_t u_csamul_rca16_fa12_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_3 = 0;
|
|
uint8_t u_csamul_rca16_fa13_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_3 = 0;
|
|
uint8_t u_csamul_rca16_fa14_3_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_3_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_3 = 0;
|
|
uint8_t u_csamul_rca16_and0_4 = 0;
|
|
uint8_t u_csamul_rca16_fa0_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_4 = 0;
|
|
uint8_t u_csamul_rca16_fa1_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_4 = 0;
|
|
uint8_t u_csamul_rca16_fa2_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_4 = 0;
|
|
uint8_t u_csamul_rca16_fa3_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_4 = 0;
|
|
uint8_t u_csamul_rca16_fa4_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_4 = 0;
|
|
uint8_t u_csamul_rca16_fa5_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_4 = 0;
|
|
uint8_t u_csamul_rca16_fa6_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_4 = 0;
|
|
uint8_t u_csamul_rca16_fa7_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_4 = 0;
|
|
uint8_t u_csamul_rca16_fa8_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_4 = 0;
|
|
uint8_t u_csamul_rca16_fa9_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_4 = 0;
|
|
uint8_t u_csamul_rca16_fa10_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_4 = 0;
|
|
uint8_t u_csamul_rca16_fa11_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_4 = 0;
|
|
uint8_t u_csamul_rca16_fa12_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_4 = 0;
|
|
uint8_t u_csamul_rca16_fa13_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_4 = 0;
|
|
uint8_t u_csamul_rca16_fa14_4_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_4_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_4 = 0;
|
|
uint8_t u_csamul_rca16_and0_5 = 0;
|
|
uint8_t u_csamul_rca16_fa0_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_5 = 0;
|
|
uint8_t u_csamul_rca16_fa1_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_5 = 0;
|
|
uint8_t u_csamul_rca16_fa2_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_5 = 0;
|
|
uint8_t u_csamul_rca16_fa3_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_5 = 0;
|
|
uint8_t u_csamul_rca16_fa4_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_5 = 0;
|
|
uint8_t u_csamul_rca16_fa5_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_5 = 0;
|
|
uint8_t u_csamul_rca16_fa6_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_5 = 0;
|
|
uint8_t u_csamul_rca16_fa7_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_5 = 0;
|
|
uint8_t u_csamul_rca16_fa8_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_5 = 0;
|
|
uint8_t u_csamul_rca16_fa9_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_5 = 0;
|
|
uint8_t u_csamul_rca16_fa10_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_5 = 0;
|
|
uint8_t u_csamul_rca16_fa11_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_5 = 0;
|
|
uint8_t u_csamul_rca16_fa12_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_5 = 0;
|
|
uint8_t u_csamul_rca16_fa13_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_5 = 0;
|
|
uint8_t u_csamul_rca16_fa14_5_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_5_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_5 = 0;
|
|
uint8_t u_csamul_rca16_and0_6 = 0;
|
|
uint8_t u_csamul_rca16_fa0_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_6 = 0;
|
|
uint8_t u_csamul_rca16_fa1_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_6 = 0;
|
|
uint8_t u_csamul_rca16_fa2_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_6 = 0;
|
|
uint8_t u_csamul_rca16_fa3_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_6 = 0;
|
|
uint8_t u_csamul_rca16_fa4_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_6 = 0;
|
|
uint8_t u_csamul_rca16_fa5_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_6 = 0;
|
|
uint8_t u_csamul_rca16_fa6_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_6 = 0;
|
|
uint8_t u_csamul_rca16_fa7_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_6 = 0;
|
|
uint8_t u_csamul_rca16_fa8_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_6 = 0;
|
|
uint8_t u_csamul_rca16_fa9_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_6 = 0;
|
|
uint8_t u_csamul_rca16_fa10_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_6 = 0;
|
|
uint8_t u_csamul_rca16_fa11_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_6 = 0;
|
|
uint8_t u_csamul_rca16_fa12_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_6 = 0;
|
|
uint8_t u_csamul_rca16_fa13_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_6 = 0;
|
|
uint8_t u_csamul_rca16_fa14_6_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_6_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_6 = 0;
|
|
uint8_t u_csamul_rca16_and0_7 = 0;
|
|
uint8_t u_csamul_rca16_fa0_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_7 = 0;
|
|
uint8_t u_csamul_rca16_fa1_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_7 = 0;
|
|
uint8_t u_csamul_rca16_fa2_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_7 = 0;
|
|
uint8_t u_csamul_rca16_fa3_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_7 = 0;
|
|
uint8_t u_csamul_rca16_fa4_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_7 = 0;
|
|
uint8_t u_csamul_rca16_fa5_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_7 = 0;
|
|
uint8_t u_csamul_rca16_fa6_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_7 = 0;
|
|
uint8_t u_csamul_rca16_fa7_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_7 = 0;
|
|
uint8_t u_csamul_rca16_fa8_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_7 = 0;
|
|
uint8_t u_csamul_rca16_fa9_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_7 = 0;
|
|
uint8_t u_csamul_rca16_fa10_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_7 = 0;
|
|
uint8_t u_csamul_rca16_fa11_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_7 = 0;
|
|
uint8_t u_csamul_rca16_fa12_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_7 = 0;
|
|
uint8_t u_csamul_rca16_fa13_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_7 = 0;
|
|
uint8_t u_csamul_rca16_fa14_7_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_7_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_7 = 0;
|
|
uint8_t u_csamul_rca16_and0_8 = 0;
|
|
uint8_t u_csamul_rca16_fa0_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_8 = 0;
|
|
uint8_t u_csamul_rca16_fa1_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_8 = 0;
|
|
uint8_t u_csamul_rca16_fa2_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_8 = 0;
|
|
uint8_t u_csamul_rca16_fa3_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_8 = 0;
|
|
uint8_t u_csamul_rca16_fa4_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_8 = 0;
|
|
uint8_t u_csamul_rca16_fa5_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_8 = 0;
|
|
uint8_t u_csamul_rca16_fa6_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_8 = 0;
|
|
uint8_t u_csamul_rca16_fa7_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_8 = 0;
|
|
uint8_t u_csamul_rca16_fa8_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_8 = 0;
|
|
uint8_t u_csamul_rca16_fa9_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_8 = 0;
|
|
uint8_t u_csamul_rca16_fa10_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_8 = 0;
|
|
uint8_t u_csamul_rca16_fa11_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_8 = 0;
|
|
uint8_t u_csamul_rca16_fa12_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_8 = 0;
|
|
uint8_t u_csamul_rca16_fa13_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_8 = 0;
|
|
uint8_t u_csamul_rca16_fa14_8_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_8_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_8 = 0;
|
|
uint8_t u_csamul_rca16_and0_9 = 0;
|
|
uint8_t u_csamul_rca16_fa0_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_9 = 0;
|
|
uint8_t u_csamul_rca16_fa1_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_9 = 0;
|
|
uint8_t u_csamul_rca16_fa2_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_9 = 0;
|
|
uint8_t u_csamul_rca16_fa3_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_9 = 0;
|
|
uint8_t u_csamul_rca16_fa4_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_9 = 0;
|
|
uint8_t u_csamul_rca16_fa5_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_9 = 0;
|
|
uint8_t u_csamul_rca16_fa6_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_9 = 0;
|
|
uint8_t u_csamul_rca16_fa7_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_9 = 0;
|
|
uint8_t u_csamul_rca16_fa8_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_9 = 0;
|
|
uint8_t u_csamul_rca16_fa9_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_9 = 0;
|
|
uint8_t u_csamul_rca16_fa10_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_9 = 0;
|
|
uint8_t u_csamul_rca16_fa11_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_9 = 0;
|
|
uint8_t u_csamul_rca16_fa12_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_9 = 0;
|
|
uint8_t u_csamul_rca16_fa13_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_9 = 0;
|
|
uint8_t u_csamul_rca16_fa14_9_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_9_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_9 = 0;
|
|
uint8_t u_csamul_rca16_and0_10 = 0;
|
|
uint8_t u_csamul_rca16_fa0_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_10 = 0;
|
|
uint8_t u_csamul_rca16_fa1_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_10 = 0;
|
|
uint8_t u_csamul_rca16_fa2_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_10 = 0;
|
|
uint8_t u_csamul_rca16_fa3_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_10 = 0;
|
|
uint8_t u_csamul_rca16_fa4_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_10 = 0;
|
|
uint8_t u_csamul_rca16_fa5_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_10 = 0;
|
|
uint8_t u_csamul_rca16_fa6_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_10 = 0;
|
|
uint8_t u_csamul_rca16_fa7_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_10 = 0;
|
|
uint8_t u_csamul_rca16_fa8_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_10 = 0;
|
|
uint8_t u_csamul_rca16_fa9_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_10 = 0;
|
|
uint8_t u_csamul_rca16_fa10_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_10 = 0;
|
|
uint8_t u_csamul_rca16_fa11_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_10 = 0;
|
|
uint8_t u_csamul_rca16_fa12_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_10 = 0;
|
|
uint8_t u_csamul_rca16_fa13_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_10 = 0;
|
|
uint8_t u_csamul_rca16_fa14_10_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_10_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_10 = 0;
|
|
uint8_t u_csamul_rca16_and0_11 = 0;
|
|
uint8_t u_csamul_rca16_fa0_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_11 = 0;
|
|
uint8_t u_csamul_rca16_fa1_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_11 = 0;
|
|
uint8_t u_csamul_rca16_fa2_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_11 = 0;
|
|
uint8_t u_csamul_rca16_fa3_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_11 = 0;
|
|
uint8_t u_csamul_rca16_fa4_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_11 = 0;
|
|
uint8_t u_csamul_rca16_fa5_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_11 = 0;
|
|
uint8_t u_csamul_rca16_fa6_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_11 = 0;
|
|
uint8_t u_csamul_rca16_fa7_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_11 = 0;
|
|
uint8_t u_csamul_rca16_fa8_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_11 = 0;
|
|
uint8_t u_csamul_rca16_fa9_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_11 = 0;
|
|
uint8_t u_csamul_rca16_fa10_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_11 = 0;
|
|
uint8_t u_csamul_rca16_fa11_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_11 = 0;
|
|
uint8_t u_csamul_rca16_fa12_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_11 = 0;
|
|
uint8_t u_csamul_rca16_fa13_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_11 = 0;
|
|
uint8_t u_csamul_rca16_fa14_11_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_11_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_11 = 0;
|
|
uint8_t u_csamul_rca16_and0_12 = 0;
|
|
uint8_t u_csamul_rca16_fa0_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_12 = 0;
|
|
uint8_t u_csamul_rca16_fa1_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_12 = 0;
|
|
uint8_t u_csamul_rca16_fa2_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_12 = 0;
|
|
uint8_t u_csamul_rca16_fa3_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_12 = 0;
|
|
uint8_t u_csamul_rca16_fa4_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_12 = 0;
|
|
uint8_t u_csamul_rca16_fa5_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_12 = 0;
|
|
uint8_t u_csamul_rca16_fa6_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_12 = 0;
|
|
uint8_t u_csamul_rca16_fa7_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_12 = 0;
|
|
uint8_t u_csamul_rca16_fa8_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_12 = 0;
|
|
uint8_t u_csamul_rca16_fa9_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_12 = 0;
|
|
uint8_t u_csamul_rca16_fa10_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_12 = 0;
|
|
uint8_t u_csamul_rca16_fa11_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_12 = 0;
|
|
uint8_t u_csamul_rca16_fa12_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_12 = 0;
|
|
uint8_t u_csamul_rca16_fa13_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_12 = 0;
|
|
uint8_t u_csamul_rca16_fa14_12_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_12_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_12 = 0;
|
|
uint8_t u_csamul_rca16_and0_13 = 0;
|
|
uint8_t u_csamul_rca16_fa0_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_13 = 0;
|
|
uint8_t u_csamul_rca16_fa1_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_13 = 0;
|
|
uint8_t u_csamul_rca16_fa2_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_13 = 0;
|
|
uint8_t u_csamul_rca16_fa3_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_13 = 0;
|
|
uint8_t u_csamul_rca16_fa4_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_13 = 0;
|
|
uint8_t u_csamul_rca16_fa5_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_13 = 0;
|
|
uint8_t u_csamul_rca16_fa6_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_13 = 0;
|
|
uint8_t u_csamul_rca16_fa7_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_13 = 0;
|
|
uint8_t u_csamul_rca16_fa8_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_13 = 0;
|
|
uint8_t u_csamul_rca16_fa9_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_13 = 0;
|
|
uint8_t u_csamul_rca16_fa10_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_13 = 0;
|
|
uint8_t u_csamul_rca16_fa11_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_13 = 0;
|
|
uint8_t u_csamul_rca16_fa12_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_13 = 0;
|
|
uint8_t u_csamul_rca16_fa13_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_13 = 0;
|
|
uint8_t u_csamul_rca16_fa14_13_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_13_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_13 = 0;
|
|
uint8_t u_csamul_rca16_and0_14 = 0;
|
|
uint8_t u_csamul_rca16_fa0_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_14 = 0;
|
|
uint8_t u_csamul_rca16_fa1_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_14 = 0;
|
|
uint8_t u_csamul_rca16_fa2_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_14 = 0;
|
|
uint8_t u_csamul_rca16_fa3_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_14 = 0;
|
|
uint8_t u_csamul_rca16_fa4_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_14 = 0;
|
|
uint8_t u_csamul_rca16_fa5_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_14 = 0;
|
|
uint8_t u_csamul_rca16_fa6_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_14 = 0;
|
|
uint8_t u_csamul_rca16_fa7_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_14 = 0;
|
|
uint8_t u_csamul_rca16_fa8_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_14 = 0;
|
|
uint8_t u_csamul_rca16_fa9_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_14 = 0;
|
|
uint8_t u_csamul_rca16_fa10_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_14 = 0;
|
|
uint8_t u_csamul_rca16_fa11_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_14 = 0;
|
|
uint8_t u_csamul_rca16_fa12_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_14 = 0;
|
|
uint8_t u_csamul_rca16_fa13_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_14 = 0;
|
|
uint8_t u_csamul_rca16_fa14_14_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_14_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_14 = 0;
|
|
uint8_t u_csamul_rca16_and0_15 = 0;
|
|
uint8_t u_csamul_rca16_fa0_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa0_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and1_15 = 0;
|
|
uint8_t u_csamul_rca16_fa1_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa1_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and2_15 = 0;
|
|
uint8_t u_csamul_rca16_fa2_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa2_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and3_15 = 0;
|
|
uint8_t u_csamul_rca16_fa3_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa3_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and4_15 = 0;
|
|
uint8_t u_csamul_rca16_fa4_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa4_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and5_15 = 0;
|
|
uint8_t u_csamul_rca16_fa5_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa5_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and6_15 = 0;
|
|
uint8_t u_csamul_rca16_fa6_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa6_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and7_15 = 0;
|
|
uint8_t u_csamul_rca16_fa7_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa7_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and8_15 = 0;
|
|
uint8_t u_csamul_rca16_fa8_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa8_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and9_15 = 0;
|
|
uint8_t u_csamul_rca16_fa9_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa9_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and10_15 = 0;
|
|
uint8_t u_csamul_rca16_fa10_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa10_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and11_15 = 0;
|
|
uint8_t u_csamul_rca16_fa11_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa11_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and12_15 = 0;
|
|
uint8_t u_csamul_rca16_fa12_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa12_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and13_15 = 0;
|
|
uint8_t u_csamul_rca16_fa13_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa13_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and14_15 = 0;
|
|
uint8_t u_csamul_rca16_fa14_15_xor1 = 0;
|
|
uint8_t u_csamul_rca16_fa14_15_or0 = 0;
|
|
uint8_t u_csamul_rca16_and15_15 = 0;
|
|
uint64_t u_csamul_rca16_u_rca16_a = 0;
|
|
uint64_t u_csamul_rca16_u_rca16_b = 0;
|
|
uint64_t u_csamul_rca16_u_rca16_out = 0;
|
|
|
|
u_csamul_rca16_and0_0 = and_gate(((a >> 0) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and1_0 = and_gate(((a >> 1) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and2_0 = and_gate(((a >> 2) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and3_0 = and_gate(((a >> 3) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and4_0 = and_gate(((a >> 4) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and5_0 = and_gate(((a >> 5) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and6_0 = and_gate(((a >> 6) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and7_0 = and_gate(((a >> 7) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and8_0 = and_gate(((a >> 8) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and9_0 = and_gate(((a >> 9) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and10_0 = and_gate(((a >> 10) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and11_0 = and_gate(((a >> 11) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and12_0 = and_gate(((a >> 12) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and13_0 = and_gate(((a >> 13) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and14_0 = and_gate(((a >> 14) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and15_0 = and_gate(((a >> 15) & 0x01), ((b >> 0) & 0x01));
|
|
u_csamul_rca16_and0_1 = and_gate(((a >> 0) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha0_1_xor0 = (ha(((u_csamul_rca16_and0_1 >> 0) & 0x01), ((u_csamul_rca16_and1_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha0_1_and0 = (ha(((u_csamul_rca16_and0_1 >> 0) & 0x01), ((u_csamul_rca16_and1_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_1 = and_gate(((a >> 1) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha1_1_xor0 = (ha(((u_csamul_rca16_and1_1 >> 0) & 0x01), ((u_csamul_rca16_and2_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha1_1_and0 = (ha(((u_csamul_rca16_and1_1 >> 0) & 0x01), ((u_csamul_rca16_and2_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_1 = and_gate(((a >> 2) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha2_1_xor0 = (ha(((u_csamul_rca16_and2_1 >> 0) & 0x01), ((u_csamul_rca16_and3_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha2_1_and0 = (ha(((u_csamul_rca16_and2_1 >> 0) & 0x01), ((u_csamul_rca16_and3_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_1 = and_gate(((a >> 3) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha3_1_xor0 = (ha(((u_csamul_rca16_and3_1 >> 0) & 0x01), ((u_csamul_rca16_and4_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha3_1_and0 = (ha(((u_csamul_rca16_and3_1 >> 0) & 0x01), ((u_csamul_rca16_and4_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_1 = and_gate(((a >> 4) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha4_1_xor0 = (ha(((u_csamul_rca16_and4_1 >> 0) & 0x01), ((u_csamul_rca16_and5_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha4_1_and0 = (ha(((u_csamul_rca16_and4_1 >> 0) & 0x01), ((u_csamul_rca16_and5_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_1 = and_gate(((a >> 5) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha5_1_xor0 = (ha(((u_csamul_rca16_and5_1 >> 0) & 0x01), ((u_csamul_rca16_and6_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha5_1_and0 = (ha(((u_csamul_rca16_and5_1 >> 0) & 0x01), ((u_csamul_rca16_and6_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_1 = and_gate(((a >> 6) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha6_1_xor0 = (ha(((u_csamul_rca16_and6_1 >> 0) & 0x01), ((u_csamul_rca16_and7_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha6_1_and0 = (ha(((u_csamul_rca16_and6_1 >> 0) & 0x01), ((u_csamul_rca16_and7_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_1 = and_gate(((a >> 7) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha7_1_xor0 = (ha(((u_csamul_rca16_and7_1 >> 0) & 0x01), ((u_csamul_rca16_and8_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha7_1_and0 = (ha(((u_csamul_rca16_and7_1 >> 0) & 0x01), ((u_csamul_rca16_and8_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_1 = and_gate(((a >> 8) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha8_1_xor0 = (ha(((u_csamul_rca16_and8_1 >> 0) & 0x01), ((u_csamul_rca16_and9_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha8_1_and0 = (ha(((u_csamul_rca16_and8_1 >> 0) & 0x01), ((u_csamul_rca16_and9_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_1 = and_gate(((a >> 9) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha9_1_xor0 = (ha(((u_csamul_rca16_and9_1 >> 0) & 0x01), ((u_csamul_rca16_and10_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha9_1_and0 = (ha(((u_csamul_rca16_and9_1 >> 0) & 0x01), ((u_csamul_rca16_and10_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_1 = and_gate(((a >> 10) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha10_1_xor0 = (ha(((u_csamul_rca16_and10_1 >> 0) & 0x01), ((u_csamul_rca16_and11_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha10_1_and0 = (ha(((u_csamul_rca16_and10_1 >> 0) & 0x01), ((u_csamul_rca16_and11_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_1 = and_gate(((a >> 11) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha11_1_xor0 = (ha(((u_csamul_rca16_and11_1 >> 0) & 0x01), ((u_csamul_rca16_and12_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha11_1_and0 = (ha(((u_csamul_rca16_and11_1 >> 0) & 0x01), ((u_csamul_rca16_and12_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_1 = and_gate(((a >> 12) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha12_1_xor0 = (ha(((u_csamul_rca16_and12_1 >> 0) & 0x01), ((u_csamul_rca16_and13_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha12_1_and0 = (ha(((u_csamul_rca16_and12_1 >> 0) & 0x01), ((u_csamul_rca16_and13_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_1 = and_gate(((a >> 13) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha13_1_xor0 = (ha(((u_csamul_rca16_and13_1 >> 0) & 0x01), ((u_csamul_rca16_and14_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha13_1_and0 = (ha(((u_csamul_rca16_and13_1 >> 0) & 0x01), ((u_csamul_rca16_and14_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_1 = and_gate(((a >> 14) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_ha14_1_xor0 = (ha(((u_csamul_rca16_and14_1 >> 0) & 0x01), ((u_csamul_rca16_and15_0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_ha14_1_and0 = (ha(((u_csamul_rca16_and14_1 >> 0) & 0x01), ((u_csamul_rca16_and15_0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_1 = and_gate(((a >> 15) & 0x01), ((b >> 1) & 0x01));
|
|
u_csamul_rca16_and0_2 = and_gate(((a >> 0) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa0_2_xor1 = (fa(((u_csamul_rca16_and0_2 >> 0) & 0x01), ((u_csamul_rca16_ha1_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha0_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_2_or0 = (fa(((u_csamul_rca16_and0_2 >> 0) & 0x01), ((u_csamul_rca16_ha1_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha0_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_2 = and_gate(((a >> 1) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa1_2_xor1 = (fa(((u_csamul_rca16_and1_2 >> 0) & 0x01), ((u_csamul_rca16_ha2_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha1_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_2_or0 = (fa(((u_csamul_rca16_and1_2 >> 0) & 0x01), ((u_csamul_rca16_ha2_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha1_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_2 = and_gate(((a >> 2) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa2_2_xor1 = (fa(((u_csamul_rca16_and2_2 >> 0) & 0x01), ((u_csamul_rca16_ha3_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha2_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_2_or0 = (fa(((u_csamul_rca16_and2_2 >> 0) & 0x01), ((u_csamul_rca16_ha3_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha2_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_2 = and_gate(((a >> 3) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa3_2_xor1 = (fa(((u_csamul_rca16_and3_2 >> 0) & 0x01), ((u_csamul_rca16_ha4_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha3_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_2_or0 = (fa(((u_csamul_rca16_and3_2 >> 0) & 0x01), ((u_csamul_rca16_ha4_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha3_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_2 = and_gate(((a >> 4) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa4_2_xor1 = (fa(((u_csamul_rca16_and4_2 >> 0) & 0x01), ((u_csamul_rca16_ha5_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha4_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_2_or0 = (fa(((u_csamul_rca16_and4_2 >> 0) & 0x01), ((u_csamul_rca16_ha5_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha4_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_2 = and_gate(((a >> 5) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa5_2_xor1 = (fa(((u_csamul_rca16_and5_2 >> 0) & 0x01), ((u_csamul_rca16_ha6_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha5_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_2_or0 = (fa(((u_csamul_rca16_and5_2 >> 0) & 0x01), ((u_csamul_rca16_ha6_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha5_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_2 = and_gate(((a >> 6) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa6_2_xor1 = (fa(((u_csamul_rca16_and6_2 >> 0) & 0x01), ((u_csamul_rca16_ha7_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha6_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_2_or0 = (fa(((u_csamul_rca16_and6_2 >> 0) & 0x01), ((u_csamul_rca16_ha7_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha6_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_2 = and_gate(((a >> 7) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa7_2_xor1 = (fa(((u_csamul_rca16_and7_2 >> 0) & 0x01), ((u_csamul_rca16_ha8_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha7_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_2_or0 = (fa(((u_csamul_rca16_and7_2 >> 0) & 0x01), ((u_csamul_rca16_ha8_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha7_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_2 = and_gate(((a >> 8) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa8_2_xor1 = (fa(((u_csamul_rca16_and8_2 >> 0) & 0x01), ((u_csamul_rca16_ha9_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha8_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_2_or0 = (fa(((u_csamul_rca16_and8_2 >> 0) & 0x01), ((u_csamul_rca16_ha9_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha8_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_2 = and_gate(((a >> 9) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa9_2_xor1 = (fa(((u_csamul_rca16_and9_2 >> 0) & 0x01), ((u_csamul_rca16_ha10_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha9_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_2_or0 = (fa(((u_csamul_rca16_and9_2 >> 0) & 0x01), ((u_csamul_rca16_ha10_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha9_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_2 = and_gate(((a >> 10) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa10_2_xor1 = (fa(((u_csamul_rca16_and10_2 >> 0) & 0x01), ((u_csamul_rca16_ha11_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha10_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_2_or0 = (fa(((u_csamul_rca16_and10_2 >> 0) & 0x01), ((u_csamul_rca16_ha11_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha10_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_2 = and_gate(((a >> 11) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa11_2_xor1 = (fa(((u_csamul_rca16_and11_2 >> 0) & 0x01), ((u_csamul_rca16_ha12_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha11_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_2_or0 = (fa(((u_csamul_rca16_and11_2 >> 0) & 0x01), ((u_csamul_rca16_ha12_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha11_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_2 = and_gate(((a >> 12) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa12_2_xor1 = (fa(((u_csamul_rca16_and12_2 >> 0) & 0x01), ((u_csamul_rca16_ha13_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha12_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_2_or0 = (fa(((u_csamul_rca16_and12_2 >> 0) & 0x01), ((u_csamul_rca16_ha13_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha12_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_2 = and_gate(((a >> 13) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa13_2_xor1 = (fa(((u_csamul_rca16_and13_2 >> 0) & 0x01), ((u_csamul_rca16_ha14_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha13_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_2_or0 = (fa(((u_csamul_rca16_and13_2 >> 0) & 0x01), ((u_csamul_rca16_ha14_1_xor0 >> 0) & 0x01), ((u_csamul_rca16_ha13_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_2 = and_gate(((a >> 14) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_fa14_2_xor1 = (fa(((u_csamul_rca16_and14_2 >> 0) & 0x01), ((u_csamul_rca16_and15_1 >> 0) & 0x01), ((u_csamul_rca16_ha14_1_and0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_2_or0 = (fa(((u_csamul_rca16_and14_2 >> 0) & 0x01), ((u_csamul_rca16_and15_1 >> 0) & 0x01), ((u_csamul_rca16_ha14_1_and0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_2 = and_gate(((a >> 15) & 0x01), ((b >> 2) & 0x01));
|
|
u_csamul_rca16_and0_3 = and_gate(((a >> 0) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa0_3_xor1 = (fa(((u_csamul_rca16_and0_3 >> 0) & 0x01), ((u_csamul_rca16_fa1_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_3_or0 = (fa(((u_csamul_rca16_and0_3 >> 0) & 0x01), ((u_csamul_rca16_fa1_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_3 = and_gate(((a >> 1) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa1_3_xor1 = (fa(((u_csamul_rca16_and1_3 >> 0) & 0x01), ((u_csamul_rca16_fa2_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_3_or0 = (fa(((u_csamul_rca16_and1_3 >> 0) & 0x01), ((u_csamul_rca16_fa2_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_3 = and_gate(((a >> 2) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa2_3_xor1 = (fa(((u_csamul_rca16_and2_3 >> 0) & 0x01), ((u_csamul_rca16_fa3_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_3_or0 = (fa(((u_csamul_rca16_and2_3 >> 0) & 0x01), ((u_csamul_rca16_fa3_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_3 = and_gate(((a >> 3) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa3_3_xor1 = (fa(((u_csamul_rca16_and3_3 >> 0) & 0x01), ((u_csamul_rca16_fa4_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_3_or0 = (fa(((u_csamul_rca16_and3_3 >> 0) & 0x01), ((u_csamul_rca16_fa4_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_3 = and_gate(((a >> 4) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa4_3_xor1 = (fa(((u_csamul_rca16_and4_3 >> 0) & 0x01), ((u_csamul_rca16_fa5_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_3_or0 = (fa(((u_csamul_rca16_and4_3 >> 0) & 0x01), ((u_csamul_rca16_fa5_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_3 = and_gate(((a >> 5) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa5_3_xor1 = (fa(((u_csamul_rca16_and5_3 >> 0) & 0x01), ((u_csamul_rca16_fa6_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_3_or0 = (fa(((u_csamul_rca16_and5_3 >> 0) & 0x01), ((u_csamul_rca16_fa6_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_3 = and_gate(((a >> 6) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa6_3_xor1 = (fa(((u_csamul_rca16_and6_3 >> 0) & 0x01), ((u_csamul_rca16_fa7_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_3_or0 = (fa(((u_csamul_rca16_and6_3 >> 0) & 0x01), ((u_csamul_rca16_fa7_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_3 = and_gate(((a >> 7) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa7_3_xor1 = (fa(((u_csamul_rca16_and7_3 >> 0) & 0x01), ((u_csamul_rca16_fa8_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_3_or0 = (fa(((u_csamul_rca16_and7_3 >> 0) & 0x01), ((u_csamul_rca16_fa8_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_3 = and_gate(((a >> 8) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa8_3_xor1 = (fa(((u_csamul_rca16_and8_3 >> 0) & 0x01), ((u_csamul_rca16_fa9_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_3_or0 = (fa(((u_csamul_rca16_and8_3 >> 0) & 0x01), ((u_csamul_rca16_fa9_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_3 = and_gate(((a >> 9) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa9_3_xor1 = (fa(((u_csamul_rca16_and9_3 >> 0) & 0x01), ((u_csamul_rca16_fa10_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_3_or0 = (fa(((u_csamul_rca16_and9_3 >> 0) & 0x01), ((u_csamul_rca16_fa10_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_3 = and_gate(((a >> 10) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa10_3_xor1 = (fa(((u_csamul_rca16_and10_3 >> 0) & 0x01), ((u_csamul_rca16_fa11_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_3_or0 = (fa(((u_csamul_rca16_and10_3 >> 0) & 0x01), ((u_csamul_rca16_fa11_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_3 = and_gate(((a >> 11) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa11_3_xor1 = (fa(((u_csamul_rca16_and11_3 >> 0) & 0x01), ((u_csamul_rca16_fa12_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_3_or0 = (fa(((u_csamul_rca16_and11_3 >> 0) & 0x01), ((u_csamul_rca16_fa12_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_3 = and_gate(((a >> 12) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa12_3_xor1 = (fa(((u_csamul_rca16_and12_3 >> 0) & 0x01), ((u_csamul_rca16_fa13_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_3_or0 = (fa(((u_csamul_rca16_and12_3 >> 0) & 0x01), ((u_csamul_rca16_fa13_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_3 = and_gate(((a >> 13) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa13_3_xor1 = (fa(((u_csamul_rca16_and13_3 >> 0) & 0x01), ((u_csamul_rca16_fa14_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_3_or0 = (fa(((u_csamul_rca16_and13_3 >> 0) & 0x01), ((u_csamul_rca16_fa14_2_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_3 = and_gate(((a >> 14) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_fa14_3_xor1 = (fa(((u_csamul_rca16_and14_3 >> 0) & 0x01), ((u_csamul_rca16_and15_2 >> 0) & 0x01), ((u_csamul_rca16_fa14_2_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_3_or0 = (fa(((u_csamul_rca16_and14_3 >> 0) & 0x01), ((u_csamul_rca16_and15_2 >> 0) & 0x01), ((u_csamul_rca16_fa14_2_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_3 = and_gate(((a >> 15) & 0x01), ((b >> 3) & 0x01));
|
|
u_csamul_rca16_and0_4 = and_gate(((a >> 0) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa0_4_xor1 = (fa(((u_csamul_rca16_and0_4 >> 0) & 0x01), ((u_csamul_rca16_fa1_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_4_or0 = (fa(((u_csamul_rca16_and0_4 >> 0) & 0x01), ((u_csamul_rca16_fa1_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_4 = and_gate(((a >> 1) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa1_4_xor1 = (fa(((u_csamul_rca16_and1_4 >> 0) & 0x01), ((u_csamul_rca16_fa2_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_4_or0 = (fa(((u_csamul_rca16_and1_4 >> 0) & 0x01), ((u_csamul_rca16_fa2_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_4 = and_gate(((a >> 2) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa2_4_xor1 = (fa(((u_csamul_rca16_and2_4 >> 0) & 0x01), ((u_csamul_rca16_fa3_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_4_or0 = (fa(((u_csamul_rca16_and2_4 >> 0) & 0x01), ((u_csamul_rca16_fa3_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_4 = and_gate(((a >> 3) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa3_4_xor1 = (fa(((u_csamul_rca16_and3_4 >> 0) & 0x01), ((u_csamul_rca16_fa4_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_4_or0 = (fa(((u_csamul_rca16_and3_4 >> 0) & 0x01), ((u_csamul_rca16_fa4_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_4 = and_gate(((a >> 4) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa4_4_xor1 = (fa(((u_csamul_rca16_and4_4 >> 0) & 0x01), ((u_csamul_rca16_fa5_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_4_or0 = (fa(((u_csamul_rca16_and4_4 >> 0) & 0x01), ((u_csamul_rca16_fa5_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_4 = and_gate(((a >> 5) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa5_4_xor1 = (fa(((u_csamul_rca16_and5_4 >> 0) & 0x01), ((u_csamul_rca16_fa6_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_4_or0 = (fa(((u_csamul_rca16_and5_4 >> 0) & 0x01), ((u_csamul_rca16_fa6_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_4 = and_gate(((a >> 6) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa6_4_xor1 = (fa(((u_csamul_rca16_and6_4 >> 0) & 0x01), ((u_csamul_rca16_fa7_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_4_or0 = (fa(((u_csamul_rca16_and6_4 >> 0) & 0x01), ((u_csamul_rca16_fa7_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_4 = and_gate(((a >> 7) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa7_4_xor1 = (fa(((u_csamul_rca16_and7_4 >> 0) & 0x01), ((u_csamul_rca16_fa8_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_4_or0 = (fa(((u_csamul_rca16_and7_4 >> 0) & 0x01), ((u_csamul_rca16_fa8_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_4 = and_gate(((a >> 8) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa8_4_xor1 = (fa(((u_csamul_rca16_and8_4 >> 0) & 0x01), ((u_csamul_rca16_fa9_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_4_or0 = (fa(((u_csamul_rca16_and8_4 >> 0) & 0x01), ((u_csamul_rca16_fa9_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_4 = and_gate(((a >> 9) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa9_4_xor1 = (fa(((u_csamul_rca16_and9_4 >> 0) & 0x01), ((u_csamul_rca16_fa10_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_4_or0 = (fa(((u_csamul_rca16_and9_4 >> 0) & 0x01), ((u_csamul_rca16_fa10_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_4 = and_gate(((a >> 10) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa10_4_xor1 = (fa(((u_csamul_rca16_and10_4 >> 0) & 0x01), ((u_csamul_rca16_fa11_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_4_or0 = (fa(((u_csamul_rca16_and10_4 >> 0) & 0x01), ((u_csamul_rca16_fa11_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_4 = and_gate(((a >> 11) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa11_4_xor1 = (fa(((u_csamul_rca16_and11_4 >> 0) & 0x01), ((u_csamul_rca16_fa12_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_4_or0 = (fa(((u_csamul_rca16_and11_4 >> 0) & 0x01), ((u_csamul_rca16_fa12_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_4 = and_gate(((a >> 12) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa12_4_xor1 = (fa(((u_csamul_rca16_and12_4 >> 0) & 0x01), ((u_csamul_rca16_fa13_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_4_or0 = (fa(((u_csamul_rca16_and12_4 >> 0) & 0x01), ((u_csamul_rca16_fa13_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_4 = and_gate(((a >> 13) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa13_4_xor1 = (fa(((u_csamul_rca16_and13_4 >> 0) & 0x01), ((u_csamul_rca16_fa14_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_4_or0 = (fa(((u_csamul_rca16_and13_4 >> 0) & 0x01), ((u_csamul_rca16_fa14_3_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_4 = and_gate(((a >> 14) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_fa14_4_xor1 = (fa(((u_csamul_rca16_and14_4 >> 0) & 0x01), ((u_csamul_rca16_and15_3 >> 0) & 0x01), ((u_csamul_rca16_fa14_3_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_4_or0 = (fa(((u_csamul_rca16_and14_4 >> 0) & 0x01), ((u_csamul_rca16_and15_3 >> 0) & 0x01), ((u_csamul_rca16_fa14_3_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_4 = and_gate(((a >> 15) & 0x01), ((b >> 4) & 0x01));
|
|
u_csamul_rca16_and0_5 = and_gate(((a >> 0) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa0_5_xor1 = (fa(((u_csamul_rca16_and0_5 >> 0) & 0x01), ((u_csamul_rca16_fa1_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_5_or0 = (fa(((u_csamul_rca16_and0_5 >> 0) & 0x01), ((u_csamul_rca16_fa1_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_5 = and_gate(((a >> 1) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa1_5_xor1 = (fa(((u_csamul_rca16_and1_5 >> 0) & 0x01), ((u_csamul_rca16_fa2_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_5_or0 = (fa(((u_csamul_rca16_and1_5 >> 0) & 0x01), ((u_csamul_rca16_fa2_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_5 = and_gate(((a >> 2) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa2_5_xor1 = (fa(((u_csamul_rca16_and2_5 >> 0) & 0x01), ((u_csamul_rca16_fa3_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_5_or0 = (fa(((u_csamul_rca16_and2_5 >> 0) & 0x01), ((u_csamul_rca16_fa3_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_5 = and_gate(((a >> 3) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa3_5_xor1 = (fa(((u_csamul_rca16_and3_5 >> 0) & 0x01), ((u_csamul_rca16_fa4_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_5_or0 = (fa(((u_csamul_rca16_and3_5 >> 0) & 0x01), ((u_csamul_rca16_fa4_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_5 = and_gate(((a >> 4) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa4_5_xor1 = (fa(((u_csamul_rca16_and4_5 >> 0) & 0x01), ((u_csamul_rca16_fa5_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_5_or0 = (fa(((u_csamul_rca16_and4_5 >> 0) & 0x01), ((u_csamul_rca16_fa5_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_5 = and_gate(((a >> 5) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa5_5_xor1 = (fa(((u_csamul_rca16_and5_5 >> 0) & 0x01), ((u_csamul_rca16_fa6_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_5_or0 = (fa(((u_csamul_rca16_and5_5 >> 0) & 0x01), ((u_csamul_rca16_fa6_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_5 = and_gate(((a >> 6) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa6_5_xor1 = (fa(((u_csamul_rca16_and6_5 >> 0) & 0x01), ((u_csamul_rca16_fa7_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_5_or0 = (fa(((u_csamul_rca16_and6_5 >> 0) & 0x01), ((u_csamul_rca16_fa7_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_5 = and_gate(((a >> 7) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa7_5_xor1 = (fa(((u_csamul_rca16_and7_5 >> 0) & 0x01), ((u_csamul_rca16_fa8_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_5_or0 = (fa(((u_csamul_rca16_and7_5 >> 0) & 0x01), ((u_csamul_rca16_fa8_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_5 = and_gate(((a >> 8) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa8_5_xor1 = (fa(((u_csamul_rca16_and8_5 >> 0) & 0x01), ((u_csamul_rca16_fa9_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_5_or0 = (fa(((u_csamul_rca16_and8_5 >> 0) & 0x01), ((u_csamul_rca16_fa9_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_5 = and_gate(((a >> 9) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa9_5_xor1 = (fa(((u_csamul_rca16_and9_5 >> 0) & 0x01), ((u_csamul_rca16_fa10_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_5_or0 = (fa(((u_csamul_rca16_and9_5 >> 0) & 0x01), ((u_csamul_rca16_fa10_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_5 = and_gate(((a >> 10) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa10_5_xor1 = (fa(((u_csamul_rca16_and10_5 >> 0) & 0x01), ((u_csamul_rca16_fa11_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_5_or0 = (fa(((u_csamul_rca16_and10_5 >> 0) & 0x01), ((u_csamul_rca16_fa11_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_5 = and_gate(((a >> 11) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa11_5_xor1 = (fa(((u_csamul_rca16_and11_5 >> 0) & 0x01), ((u_csamul_rca16_fa12_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_5_or0 = (fa(((u_csamul_rca16_and11_5 >> 0) & 0x01), ((u_csamul_rca16_fa12_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_5 = and_gate(((a >> 12) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa12_5_xor1 = (fa(((u_csamul_rca16_and12_5 >> 0) & 0x01), ((u_csamul_rca16_fa13_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_5_or0 = (fa(((u_csamul_rca16_and12_5 >> 0) & 0x01), ((u_csamul_rca16_fa13_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_5 = and_gate(((a >> 13) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa13_5_xor1 = (fa(((u_csamul_rca16_and13_5 >> 0) & 0x01), ((u_csamul_rca16_fa14_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_5_or0 = (fa(((u_csamul_rca16_and13_5 >> 0) & 0x01), ((u_csamul_rca16_fa14_4_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_5 = and_gate(((a >> 14) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_fa14_5_xor1 = (fa(((u_csamul_rca16_and14_5 >> 0) & 0x01), ((u_csamul_rca16_and15_4 >> 0) & 0x01), ((u_csamul_rca16_fa14_4_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_5_or0 = (fa(((u_csamul_rca16_and14_5 >> 0) & 0x01), ((u_csamul_rca16_and15_4 >> 0) & 0x01), ((u_csamul_rca16_fa14_4_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_5 = and_gate(((a >> 15) & 0x01), ((b >> 5) & 0x01));
|
|
u_csamul_rca16_and0_6 = and_gate(((a >> 0) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa0_6_xor1 = (fa(((u_csamul_rca16_and0_6 >> 0) & 0x01), ((u_csamul_rca16_fa1_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_6_or0 = (fa(((u_csamul_rca16_and0_6 >> 0) & 0x01), ((u_csamul_rca16_fa1_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_6 = and_gate(((a >> 1) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa1_6_xor1 = (fa(((u_csamul_rca16_and1_6 >> 0) & 0x01), ((u_csamul_rca16_fa2_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_6_or0 = (fa(((u_csamul_rca16_and1_6 >> 0) & 0x01), ((u_csamul_rca16_fa2_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_6 = and_gate(((a >> 2) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa2_6_xor1 = (fa(((u_csamul_rca16_and2_6 >> 0) & 0x01), ((u_csamul_rca16_fa3_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_6_or0 = (fa(((u_csamul_rca16_and2_6 >> 0) & 0x01), ((u_csamul_rca16_fa3_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_6 = and_gate(((a >> 3) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa3_6_xor1 = (fa(((u_csamul_rca16_and3_6 >> 0) & 0x01), ((u_csamul_rca16_fa4_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_6_or0 = (fa(((u_csamul_rca16_and3_6 >> 0) & 0x01), ((u_csamul_rca16_fa4_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_6 = and_gate(((a >> 4) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa4_6_xor1 = (fa(((u_csamul_rca16_and4_6 >> 0) & 0x01), ((u_csamul_rca16_fa5_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_6_or0 = (fa(((u_csamul_rca16_and4_6 >> 0) & 0x01), ((u_csamul_rca16_fa5_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_6 = and_gate(((a >> 5) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa5_6_xor1 = (fa(((u_csamul_rca16_and5_6 >> 0) & 0x01), ((u_csamul_rca16_fa6_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_6_or0 = (fa(((u_csamul_rca16_and5_6 >> 0) & 0x01), ((u_csamul_rca16_fa6_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_6 = and_gate(((a >> 6) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa6_6_xor1 = (fa(((u_csamul_rca16_and6_6 >> 0) & 0x01), ((u_csamul_rca16_fa7_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_6_or0 = (fa(((u_csamul_rca16_and6_6 >> 0) & 0x01), ((u_csamul_rca16_fa7_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_6 = and_gate(((a >> 7) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa7_6_xor1 = (fa(((u_csamul_rca16_and7_6 >> 0) & 0x01), ((u_csamul_rca16_fa8_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_6_or0 = (fa(((u_csamul_rca16_and7_6 >> 0) & 0x01), ((u_csamul_rca16_fa8_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_6 = and_gate(((a >> 8) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa8_6_xor1 = (fa(((u_csamul_rca16_and8_6 >> 0) & 0x01), ((u_csamul_rca16_fa9_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_6_or0 = (fa(((u_csamul_rca16_and8_6 >> 0) & 0x01), ((u_csamul_rca16_fa9_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_6 = and_gate(((a >> 9) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa9_6_xor1 = (fa(((u_csamul_rca16_and9_6 >> 0) & 0x01), ((u_csamul_rca16_fa10_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_6_or0 = (fa(((u_csamul_rca16_and9_6 >> 0) & 0x01), ((u_csamul_rca16_fa10_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_6 = and_gate(((a >> 10) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa10_6_xor1 = (fa(((u_csamul_rca16_and10_6 >> 0) & 0x01), ((u_csamul_rca16_fa11_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_6_or0 = (fa(((u_csamul_rca16_and10_6 >> 0) & 0x01), ((u_csamul_rca16_fa11_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_6 = and_gate(((a >> 11) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa11_6_xor1 = (fa(((u_csamul_rca16_and11_6 >> 0) & 0x01), ((u_csamul_rca16_fa12_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_6_or0 = (fa(((u_csamul_rca16_and11_6 >> 0) & 0x01), ((u_csamul_rca16_fa12_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_6 = and_gate(((a >> 12) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa12_6_xor1 = (fa(((u_csamul_rca16_and12_6 >> 0) & 0x01), ((u_csamul_rca16_fa13_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_6_or0 = (fa(((u_csamul_rca16_and12_6 >> 0) & 0x01), ((u_csamul_rca16_fa13_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_6 = and_gate(((a >> 13) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa13_6_xor1 = (fa(((u_csamul_rca16_and13_6 >> 0) & 0x01), ((u_csamul_rca16_fa14_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_6_or0 = (fa(((u_csamul_rca16_and13_6 >> 0) & 0x01), ((u_csamul_rca16_fa14_5_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_6 = and_gate(((a >> 14) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_fa14_6_xor1 = (fa(((u_csamul_rca16_and14_6 >> 0) & 0x01), ((u_csamul_rca16_and15_5 >> 0) & 0x01), ((u_csamul_rca16_fa14_5_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_6_or0 = (fa(((u_csamul_rca16_and14_6 >> 0) & 0x01), ((u_csamul_rca16_and15_5 >> 0) & 0x01), ((u_csamul_rca16_fa14_5_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_6 = and_gate(((a >> 15) & 0x01), ((b >> 6) & 0x01));
|
|
u_csamul_rca16_and0_7 = and_gate(((a >> 0) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa0_7_xor1 = (fa(((u_csamul_rca16_and0_7 >> 0) & 0x01), ((u_csamul_rca16_fa1_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_7_or0 = (fa(((u_csamul_rca16_and0_7 >> 0) & 0x01), ((u_csamul_rca16_fa1_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_7 = and_gate(((a >> 1) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa1_7_xor1 = (fa(((u_csamul_rca16_and1_7 >> 0) & 0x01), ((u_csamul_rca16_fa2_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_7_or0 = (fa(((u_csamul_rca16_and1_7 >> 0) & 0x01), ((u_csamul_rca16_fa2_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_7 = and_gate(((a >> 2) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa2_7_xor1 = (fa(((u_csamul_rca16_and2_7 >> 0) & 0x01), ((u_csamul_rca16_fa3_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_7_or0 = (fa(((u_csamul_rca16_and2_7 >> 0) & 0x01), ((u_csamul_rca16_fa3_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_7 = and_gate(((a >> 3) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa3_7_xor1 = (fa(((u_csamul_rca16_and3_7 >> 0) & 0x01), ((u_csamul_rca16_fa4_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_7_or0 = (fa(((u_csamul_rca16_and3_7 >> 0) & 0x01), ((u_csamul_rca16_fa4_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_7 = and_gate(((a >> 4) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa4_7_xor1 = (fa(((u_csamul_rca16_and4_7 >> 0) & 0x01), ((u_csamul_rca16_fa5_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_7_or0 = (fa(((u_csamul_rca16_and4_7 >> 0) & 0x01), ((u_csamul_rca16_fa5_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_7 = and_gate(((a >> 5) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa5_7_xor1 = (fa(((u_csamul_rca16_and5_7 >> 0) & 0x01), ((u_csamul_rca16_fa6_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_7_or0 = (fa(((u_csamul_rca16_and5_7 >> 0) & 0x01), ((u_csamul_rca16_fa6_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_7 = and_gate(((a >> 6) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa6_7_xor1 = (fa(((u_csamul_rca16_and6_7 >> 0) & 0x01), ((u_csamul_rca16_fa7_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_7_or0 = (fa(((u_csamul_rca16_and6_7 >> 0) & 0x01), ((u_csamul_rca16_fa7_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_7 = and_gate(((a >> 7) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa7_7_xor1 = (fa(((u_csamul_rca16_and7_7 >> 0) & 0x01), ((u_csamul_rca16_fa8_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_7_or0 = (fa(((u_csamul_rca16_and7_7 >> 0) & 0x01), ((u_csamul_rca16_fa8_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_7 = and_gate(((a >> 8) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa8_7_xor1 = (fa(((u_csamul_rca16_and8_7 >> 0) & 0x01), ((u_csamul_rca16_fa9_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_7_or0 = (fa(((u_csamul_rca16_and8_7 >> 0) & 0x01), ((u_csamul_rca16_fa9_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_7 = and_gate(((a >> 9) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa9_7_xor1 = (fa(((u_csamul_rca16_and9_7 >> 0) & 0x01), ((u_csamul_rca16_fa10_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_7_or0 = (fa(((u_csamul_rca16_and9_7 >> 0) & 0x01), ((u_csamul_rca16_fa10_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_7 = and_gate(((a >> 10) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa10_7_xor1 = (fa(((u_csamul_rca16_and10_7 >> 0) & 0x01), ((u_csamul_rca16_fa11_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_7_or0 = (fa(((u_csamul_rca16_and10_7 >> 0) & 0x01), ((u_csamul_rca16_fa11_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_7 = and_gate(((a >> 11) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa11_7_xor1 = (fa(((u_csamul_rca16_and11_7 >> 0) & 0x01), ((u_csamul_rca16_fa12_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_7_or0 = (fa(((u_csamul_rca16_and11_7 >> 0) & 0x01), ((u_csamul_rca16_fa12_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_7 = and_gate(((a >> 12) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa12_7_xor1 = (fa(((u_csamul_rca16_and12_7 >> 0) & 0x01), ((u_csamul_rca16_fa13_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_7_or0 = (fa(((u_csamul_rca16_and12_7 >> 0) & 0x01), ((u_csamul_rca16_fa13_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_7 = and_gate(((a >> 13) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa13_7_xor1 = (fa(((u_csamul_rca16_and13_7 >> 0) & 0x01), ((u_csamul_rca16_fa14_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_7_or0 = (fa(((u_csamul_rca16_and13_7 >> 0) & 0x01), ((u_csamul_rca16_fa14_6_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_7 = and_gate(((a >> 14) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_fa14_7_xor1 = (fa(((u_csamul_rca16_and14_7 >> 0) & 0x01), ((u_csamul_rca16_and15_6 >> 0) & 0x01), ((u_csamul_rca16_fa14_6_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_7_or0 = (fa(((u_csamul_rca16_and14_7 >> 0) & 0x01), ((u_csamul_rca16_and15_6 >> 0) & 0x01), ((u_csamul_rca16_fa14_6_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_7 = and_gate(((a >> 15) & 0x01), ((b >> 7) & 0x01));
|
|
u_csamul_rca16_and0_8 = and_gate(((a >> 0) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa0_8_xor1 = (fa(((u_csamul_rca16_and0_8 >> 0) & 0x01), ((u_csamul_rca16_fa1_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_8_or0 = (fa(((u_csamul_rca16_and0_8 >> 0) & 0x01), ((u_csamul_rca16_fa1_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_8 = and_gate(((a >> 1) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa1_8_xor1 = (fa(((u_csamul_rca16_and1_8 >> 0) & 0x01), ((u_csamul_rca16_fa2_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_8_or0 = (fa(((u_csamul_rca16_and1_8 >> 0) & 0x01), ((u_csamul_rca16_fa2_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_8 = and_gate(((a >> 2) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa2_8_xor1 = (fa(((u_csamul_rca16_and2_8 >> 0) & 0x01), ((u_csamul_rca16_fa3_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_8_or0 = (fa(((u_csamul_rca16_and2_8 >> 0) & 0x01), ((u_csamul_rca16_fa3_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_8 = and_gate(((a >> 3) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa3_8_xor1 = (fa(((u_csamul_rca16_and3_8 >> 0) & 0x01), ((u_csamul_rca16_fa4_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_8_or0 = (fa(((u_csamul_rca16_and3_8 >> 0) & 0x01), ((u_csamul_rca16_fa4_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_8 = and_gate(((a >> 4) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa4_8_xor1 = (fa(((u_csamul_rca16_and4_8 >> 0) & 0x01), ((u_csamul_rca16_fa5_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_8_or0 = (fa(((u_csamul_rca16_and4_8 >> 0) & 0x01), ((u_csamul_rca16_fa5_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_8 = and_gate(((a >> 5) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa5_8_xor1 = (fa(((u_csamul_rca16_and5_8 >> 0) & 0x01), ((u_csamul_rca16_fa6_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_8_or0 = (fa(((u_csamul_rca16_and5_8 >> 0) & 0x01), ((u_csamul_rca16_fa6_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_8 = and_gate(((a >> 6) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa6_8_xor1 = (fa(((u_csamul_rca16_and6_8 >> 0) & 0x01), ((u_csamul_rca16_fa7_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_8_or0 = (fa(((u_csamul_rca16_and6_8 >> 0) & 0x01), ((u_csamul_rca16_fa7_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_8 = and_gate(((a >> 7) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa7_8_xor1 = (fa(((u_csamul_rca16_and7_8 >> 0) & 0x01), ((u_csamul_rca16_fa8_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_8_or0 = (fa(((u_csamul_rca16_and7_8 >> 0) & 0x01), ((u_csamul_rca16_fa8_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_8 = and_gate(((a >> 8) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa8_8_xor1 = (fa(((u_csamul_rca16_and8_8 >> 0) & 0x01), ((u_csamul_rca16_fa9_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_8_or0 = (fa(((u_csamul_rca16_and8_8 >> 0) & 0x01), ((u_csamul_rca16_fa9_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_8 = and_gate(((a >> 9) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa9_8_xor1 = (fa(((u_csamul_rca16_and9_8 >> 0) & 0x01), ((u_csamul_rca16_fa10_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_8_or0 = (fa(((u_csamul_rca16_and9_8 >> 0) & 0x01), ((u_csamul_rca16_fa10_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_8 = and_gate(((a >> 10) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa10_8_xor1 = (fa(((u_csamul_rca16_and10_8 >> 0) & 0x01), ((u_csamul_rca16_fa11_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_8_or0 = (fa(((u_csamul_rca16_and10_8 >> 0) & 0x01), ((u_csamul_rca16_fa11_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_8 = and_gate(((a >> 11) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa11_8_xor1 = (fa(((u_csamul_rca16_and11_8 >> 0) & 0x01), ((u_csamul_rca16_fa12_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_8_or0 = (fa(((u_csamul_rca16_and11_8 >> 0) & 0x01), ((u_csamul_rca16_fa12_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_8 = and_gate(((a >> 12) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa12_8_xor1 = (fa(((u_csamul_rca16_and12_8 >> 0) & 0x01), ((u_csamul_rca16_fa13_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_8_or0 = (fa(((u_csamul_rca16_and12_8 >> 0) & 0x01), ((u_csamul_rca16_fa13_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_8 = and_gate(((a >> 13) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa13_8_xor1 = (fa(((u_csamul_rca16_and13_8 >> 0) & 0x01), ((u_csamul_rca16_fa14_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_8_or0 = (fa(((u_csamul_rca16_and13_8 >> 0) & 0x01), ((u_csamul_rca16_fa14_7_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_8 = and_gate(((a >> 14) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_fa14_8_xor1 = (fa(((u_csamul_rca16_and14_8 >> 0) & 0x01), ((u_csamul_rca16_and15_7 >> 0) & 0x01), ((u_csamul_rca16_fa14_7_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_8_or0 = (fa(((u_csamul_rca16_and14_8 >> 0) & 0x01), ((u_csamul_rca16_and15_7 >> 0) & 0x01), ((u_csamul_rca16_fa14_7_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_8 = and_gate(((a >> 15) & 0x01), ((b >> 8) & 0x01));
|
|
u_csamul_rca16_and0_9 = and_gate(((a >> 0) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa0_9_xor1 = (fa(((u_csamul_rca16_and0_9 >> 0) & 0x01), ((u_csamul_rca16_fa1_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_9_or0 = (fa(((u_csamul_rca16_and0_9 >> 0) & 0x01), ((u_csamul_rca16_fa1_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_9 = and_gate(((a >> 1) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa1_9_xor1 = (fa(((u_csamul_rca16_and1_9 >> 0) & 0x01), ((u_csamul_rca16_fa2_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_9_or0 = (fa(((u_csamul_rca16_and1_9 >> 0) & 0x01), ((u_csamul_rca16_fa2_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_9 = and_gate(((a >> 2) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa2_9_xor1 = (fa(((u_csamul_rca16_and2_9 >> 0) & 0x01), ((u_csamul_rca16_fa3_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_9_or0 = (fa(((u_csamul_rca16_and2_9 >> 0) & 0x01), ((u_csamul_rca16_fa3_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_9 = and_gate(((a >> 3) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa3_9_xor1 = (fa(((u_csamul_rca16_and3_9 >> 0) & 0x01), ((u_csamul_rca16_fa4_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_9_or0 = (fa(((u_csamul_rca16_and3_9 >> 0) & 0x01), ((u_csamul_rca16_fa4_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_9 = and_gate(((a >> 4) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa4_9_xor1 = (fa(((u_csamul_rca16_and4_9 >> 0) & 0x01), ((u_csamul_rca16_fa5_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_9_or0 = (fa(((u_csamul_rca16_and4_9 >> 0) & 0x01), ((u_csamul_rca16_fa5_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_9 = and_gate(((a >> 5) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa5_9_xor1 = (fa(((u_csamul_rca16_and5_9 >> 0) & 0x01), ((u_csamul_rca16_fa6_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_9_or0 = (fa(((u_csamul_rca16_and5_9 >> 0) & 0x01), ((u_csamul_rca16_fa6_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_9 = and_gate(((a >> 6) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa6_9_xor1 = (fa(((u_csamul_rca16_and6_9 >> 0) & 0x01), ((u_csamul_rca16_fa7_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_9_or0 = (fa(((u_csamul_rca16_and6_9 >> 0) & 0x01), ((u_csamul_rca16_fa7_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_9 = and_gate(((a >> 7) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa7_9_xor1 = (fa(((u_csamul_rca16_and7_9 >> 0) & 0x01), ((u_csamul_rca16_fa8_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_9_or0 = (fa(((u_csamul_rca16_and7_9 >> 0) & 0x01), ((u_csamul_rca16_fa8_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_9 = and_gate(((a >> 8) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa8_9_xor1 = (fa(((u_csamul_rca16_and8_9 >> 0) & 0x01), ((u_csamul_rca16_fa9_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_9_or0 = (fa(((u_csamul_rca16_and8_9 >> 0) & 0x01), ((u_csamul_rca16_fa9_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_9 = and_gate(((a >> 9) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa9_9_xor1 = (fa(((u_csamul_rca16_and9_9 >> 0) & 0x01), ((u_csamul_rca16_fa10_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_9_or0 = (fa(((u_csamul_rca16_and9_9 >> 0) & 0x01), ((u_csamul_rca16_fa10_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_9 = and_gate(((a >> 10) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa10_9_xor1 = (fa(((u_csamul_rca16_and10_9 >> 0) & 0x01), ((u_csamul_rca16_fa11_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_9_or0 = (fa(((u_csamul_rca16_and10_9 >> 0) & 0x01), ((u_csamul_rca16_fa11_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_9 = and_gate(((a >> 11) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa11_9_xor1 = (fa(((u_csamul_rca16_and11_9 >> 0) & 0x01), ((u_csamul_rca16_fa12_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_9_or0 = (fa(((u_csamul_rca16_and11_9 >> 0) & 0x01), ((u_csamul_rca16_fa12_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_9 = and_gate(((a >> 12) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa12_9_xor1 = (fa(((u_csamul_rca16_and12_9 >> 0) & 0x01), ((u_csamul_rca16_fa13_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_9_or0 = (fa(((u_csamul_rca16_and12_9 >> 0) & 0x01), ((u_csamul_rca16_fa13_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_9 = and_gate(((a >> 13) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa13_9_xor1 = (fa(((u_csamul_rca16_and13_9 >> 0) & 0x01), ((u_csamul_rca16_fa14_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_9_or0 = (fa(((u_csamul_rca16_and13_9 >> 0) & 0x01), ((u_csamul_rca16_fa14_8_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_9 = and_gate(((a >> 14) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_fa14_9_xor1 = (fa(((u_csamul_rca16_and14_9 >> 0) & 0x01), ((u_csamul_rca16_and15_8 >> 0) & 0x01), ((u_csamul_rca16_fa14_8_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_9_or0 = (fa(((u_csamul_rca16_and14_9 >> 0) & 0x01), ((u_csamul_rca16_and15_8 >> 0) & 0x01), ((u_csamul_rca16_fa14_8_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_9 = and_gate(((a >> 15) & 0x01), ((b >> 9) & 0x01));
|
|
u_csamul_rca16_and0_10 = and_gate(((a >> 0) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa0_10_xor1 = (fa(((u_csamul_rca16_and0_10 >> 0) & 0x01), ((u_csamul_rca16_fa1_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_10_or0 = (fa(((u_csamul_rca16_and0_10 >> 0) & 0x01), ((u_csamul_rca16_fa1_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_10 = and_gate(((a >> 1) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa1_10_xor1 = (fa(((u_csamul_rca16_and1_10 >> 0) & 0x01), ((u_csamul_rca16_fa2_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_10_or0 = (fa(((u_csamul_rca16_and1_10 >> 0) & 0x01), ((u_csamul_rca16_fa2_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_10 = and_gate(((a >> 2) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa2_10_xor1 = (fa(((u_csamul_rca16_and2_10 >> 0) & 0x01), ((u_csamul_rca16_fa3_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_10_or0 = (fa(((u_csamul_rca16_and2_10 >> 0) & 0x01), ((u_csamul_rca16_fa3_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_10 = and_gate(((a >> 3) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa3_10_xor1 = (fa(((u_csamul_rca16_and3_10 >> 0) & 0x01), ((u_csamul_rca16_fa4_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_10_or0 = (fa(((u_csamul_rca16_and3_10 >> 0) & 0x01), ((u_csamul_rca16_fa4_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_10 = and_gate(((a >> 4) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa4_10_xor1 = (fa(((u_csamul_rca16_and4_10 >> 0) & 0x01), ((u_csamul_rca16_fa5_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_10_or0 = (fa(((u_csamul_rca16_and4_10 >> 0) & 0x01), ((u_csamul_rca16_fa5_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_10 = and_gate(((a >> 5) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa5_10_xor1 = (fa(((u_csamul_rca16_and5_10 >> 0) & 0x01), ((u_csamul_rca16_fa6_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_10_or0 = (fa(((u_csamul_rca16_and5_10 >> 0) & 0x01), ((u_csamul_rca16_fa6_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_10 = and_gate(((a >> 6) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa6_10_xor1 = (fa(((u_csamul_rca16_and6_10 >> 0) & 0x01), ((u_csamul_rca16_fa7_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_10_or0 = (fa(((u_csamul_rca16_and6_10 >> 0) & 0x01), ((u_csamul_rca16_fa7_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_10 = and_gate(((a >> 7) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa7_10_xor1 = (fa(((u_csamul_rca16_and7_10 >> 0) & 0x01), ((u_csamul_rca16_fa8_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_10_or0 = (fa(((u_csamul_rca16_and7_10 >> 0) & 0x01), ((u_csamul_rca16_fa8_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_10 = and_gate(((a >> 8) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa8_10_xor1 = (fa(((u_csamul_rca16_and8_10 >> 0) & 0x01), ((u_csamul_rca16_fa9_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_10_or0 = (fa(((u_csamul_rca16_and8_10 >> 0) & 0x01), ((u_csamul_rca16_fa9_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_10 = and_gate(((a >> 9) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa9_10_xor1 = (fa(((u_csamul_rca16_and9_10 >> 0) & 0x01), ((u_csamul_rca16_fa10_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_10_or0 = (fa(((u_csamul_rca16_and9_10 >> 0) & 0x01), ((u_csamul_rca16_fa10_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_10 = and_gate(((a >> 10) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa10_10_xor1 = (fa(((u_csamul_rca16_and10_10 >> 0) & 0x01), ((u_csamul_rca16_fa11_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_10_or0 = (fa(((u_csamul_rca16_and10_10 >> 0) & 0x01), ((u_csamul_rca16_fa11_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_10 = and_gate(((a >> 11) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa11_10_xor1 = (fa(((u_csamul_rca16_and11_10 >> 0) & 0x01), ((u_csamul_rca16_fa12_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_10_or0 = (fa(((u_csamul_rca16_and11_10 >> 0) & 0x01), ((u_csamul_rca16_fa12_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_10 = and_gate(((a >> 12) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa12_10_xor1 = (fa(((u_csamul_rca16_and12_10 >> 0) & 0x01), ((u_csamul_rca16_fa13_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_10_or0 = (fa(((u_csamul_rca16_and12_10 >> 0) & 0x01), ((u_csamul_rca16_fa13_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_10 = and_gate(((a >> 13) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa13_10_xor1 = (fa(((u_csamul_rca16_and13_10 >> 0) & 0x01), ((u_csamul_rca16_fa14_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_10_or0 = (fa(((u_csamul_rca16_and13_10 >> 0) & 0x01), ((u_csamul_rca16_fa14_9_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_10 = and_gate(((a >> 14) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_fa14_10_xor1 = (fa(((u_csamul_rca16_and14_10 >> 0) & 0x01), ((u_csamul_rca16_and15_9 >> 0) & 0x01), ((u_csamul_rca16_fa14_9_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_10_or0 = (fa(((u_csamul_rca16_and14_10 >> 0) & 0x01), ((u_csamul_rca16_and15_9 >> 0) & 0x01), ((u_csamul_rca16_fa14_9_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_10 = and_gate(((a >> 15) & 0x01), ((b >> 10) & 0x01));
|
|
u_csamul_rca16_and0_11 = and_gate(((a >> 0) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa0_11_xor1 = (fa(((u_csamul_rca16_and0_11 >> 0) & 0x01), ((u_csamul_rca16_fa1_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_11_or0 = (fa(((u_csamul_rca16_and0_11 >> 0) & 0x01), ((u_csamul_rca16_fa1_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_11 = and_gate(((a >> 1) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa1_11_xor1 = (fa(((u_csamul_rca16_and1_11 >> 0) & 0x01), ((u_csamul_rca16_fa2_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_11_or0 = (fa(((u_csamul_rca16_and1_11 >> 0) & 0x01), ((u_csamul_rca16_fa2_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_11 = and_gate(((a >> 2) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa2_11_xor1 = (fa(((u_csamul_rca16_and2_11 >> 0) & 0x01), ((u_csamul_rca16_fa3_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_11_or0 = (fa(((u_csamul_rca16_and2_11 >> 0) & 0x01), ((u_csamul_rca16_fa3_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_11 = and_gate(((a >> 3) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa3_11_xor1 = (fa(((u_csamul_rca16_and3_11 >> 0) & 0x01), ((u_csamul_rca16_fa4_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_11_or0 = (fa(((u_csamul_rca16_and3_11 >> 0) & 0x01), ((u_csamul_rca16_fa4_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_11 = and_gate(((a >> 4) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa4_11_xor1 = (fa(((u_csamul_rca16_and4_11 >> 0) & 0x01), ((u_csamul_rca16_fa5_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_11_or0 = (fa(((u_csamul_rca16_and4_11 >> 0) & 0x01), ((u_csamul_rca16_fa5_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_11 = and_gate(((a >> 5) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa5_11_xor1 = (fa(((u_csamul_rca16_and5_11 >> 0) & 0x01), ((u_csamul_rca16_fa6_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_11_or0 = (fa(((u_csamul_rca16_and5_11 >> 0) & 0x01), ((u_csamul_rca16_fa6_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_11 = and_gate(((a >> 6) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa6_11_xor1 = (fa(((u_csamul_rca16_and6_11 >> 0) & 0x01), ((u_csamul_rca16_fa7_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_11_or0 = (fa(((u_csamul_rca16_and6_11 >> 0) & 0x01), ((u_csamul_rca16_fa7_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_11 = and_gate(((a >> 7) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa7_11_xor1 = (fa(((u_csamul_rca16_and7_11 >> 0) & 0x01), ((u_csamul_rca16_fa8_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_11_or0 = (fa(((u_csamul_rca16_and7_11 >> 0) & 0x01), ((u_csamul_rca16_fa8_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_11 = and_gate(((a >> 8) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa8_11_xor1 = (fa(((u_csamul_rca16_and8_11 >> 0) & 0x01), ((u_csamul_rca16_fa9_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_11_or0 = (fa(((u_csamul_rca16_and8_11 >> 0) & 0x01), ((u_csamul_rca16_fa9_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_11 = and_gate(((a >> 9) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa9_11_xor1 = (fa(((u_csamul_rca16_and9_11 >> 0) & 0x01), ((u_csamul_rca16_fa10_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_11_or0 = (fa(((u_csamul_rca16_and9_11 >> 0) & 0x01), ((u_csamul_rca16_fa10_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_11 = and_gate(((a >> 10) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa10_11_xor1 = (fa(((u_csamul_rca16_and10_11 >> 0) & 0x01), ((u_csamul_rca16_fa11_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_11_or0 = (fa(((u_csamul_rca16_and10_11 >> 0) & 0x01), ((u_csamul_rca16_fa11_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_11 = and_gate(((a >> 11) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa11_11_xor1 = (fa(((u_csamul_rca16_and11_11 >> 0) & 0x01), ((u_csamul_rca16_fa12_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_11_or0 = (fa(((u_csamul_rca16_and11_11 >> 0) & 0x01), ((u_csamul_rca16_fa12_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_11 = and_gate(((a >> 12) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa12_11_xor1 = (fa(((u_csamul_rca16_and12_11 >> 0) & 0x01), ((u_csamul_rca16_fa13_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_11_or0 = (fa(((u_csamul_rca16_and12_11 >> 0) & 0x01), ((u_csamul_rca16_fa13_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_11 = and_gate(((a >> 13) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa13_11_xor1 = (fa(((u_csamul_rca16_and13_11 >> 0) & 0x01), ((u_csamul_rca16_fa14_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_11_or0 = (fa(((u_csamul_rca16_and13_11 >> 0) & 0x01), ((u_csamul_rca16_fa14_10_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_11 = and_gate(((a >> 14) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_fa14_11_xor1 = (fa(((u_csamul_rca16_and14_11 >> 0) & 0x01), ((u_csamul_rca16_and15_10 >> 0) & 0x01), ((u_csamul_rca16_fa14_10_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_11_or0 = (fa(((u_csamul_rca16_and14_11 >> 0) & 0x01), ((u_csamul_rca16_and15_10 >> 0) & 0x01), ((u_csamul_rca16_fa14_10_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_11 = and_gate(((a >> 15) & 0x01), ((b >> 11) & 0x01));
|
|
u_csamul_rca16_and0_12 = and_gate(((a >> 0) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa0_12_xor1 = (fa(((u_csamul_rca16_and0_12 >> 0) & 0x01), ((u_csamul_rca16_fa1_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_12_or0 = (fa(((u_csamul_rca16_and0_12 >> 0) & 0x01), ((u_csamul_rca16_fa1_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_12 = and_gate(((a >> 1) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa1_12_xor1 = (fa(((u_csamul_rca16_and1_12 >> 0) & 0x01), ((u_csamul_rca16_fa2_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_12_or0 = (fa(((u_csamul_rca16_and1_12 >> 0) & 0x01), ((u_csamul_rca16_fa2_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_12 = and_gate(((a >> 2) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa2_12_xor1 = (fa(((u_csamul_rca16_and2_12 >> 0) & 0x01), ((u_csamul_rca16_fa3_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_12_or0 = (fa(((u_csamul_rca16_and2_12 >> 0) & 0x01), ((u_csamul_rca16_fa3_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_12 = and_gate(((a >> 3) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa3_12_xor1 = (fa(((u_csamul_rca16_and3_12 >> 0) & 0x01), ((u_csamul_rca16_fa4_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_12_or0 = (fa(((u_csamul_rca16_and3_12 >> 0) & 0x01), ((u_csamul_rca16_fa4_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_12 = and_gate(((a >> 4) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa4_12_xor1 = (fa(((u_csamul_rca16_and4_12 >> 0) & 0x01), ((u_csamul_rca16_fa5_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_12_or0 = (fa(((u_csamul_rca16_and4_12 >> 0) & 0x01), ((u_csamul_rca16_fa5_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_12 = and_gate(((a >> 5) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa5_12_xor1 = (fa(((u_csamul_rca16_and5_12 >> 0) & 0x01), ((u_csamul_rca16_fa6_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_12_or0 = (fa(((u_csamul_rca16_and5_12 >> 0) & 0x01), ((u_csamul_rca16_fa6_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_12 = and_gate(((a >> 6) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa6_12_xor1 = (fa(((u_csamul_rca16_and6_12 >> 0) & 0x01), ((u_csamul_rca16_fa7_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_12_or0 = (fa(((u_csamul_rca16_and6_12 >> 0) & 0x01), ((u_csamul_rca16_fa7_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_12 = and_gate(((a >> 7) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa7_12_xor1 = (fa(((u_csamul_rca16_and7_12 >> 0) & 0x01), ((u_csamul_rca16_fa8_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_12_or0 = (fa(((u_csamul_rca16_and7_12 >> 0) & 0x01), ((u_csamul_rca16_fa8_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_12 = and_gate(((a >> 8) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa8_12_xor1 = (fa(((u_csamul_rca16_and8_12 >> 0) & 0x01), ((u_csamul_rca16_fa9_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_12_or0 = (fa(((u_csamul_rca16_and8_12 >> 0) & 0x01), ((u_csamul_rca16_fa9_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_12 = and_gate(((a >> 9) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa9_12_xor1 = (fa(((u_csamul_rca16_and9_12 >> 0) & 0x01), ((u_csamul_rca16_fa10_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_12_or0 = (fa(((u_csamul_rca16_and9_12 >> 0) & 0x01), ((u_csamul_rca16_fa10_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_12 = and_gate(((a >> 10) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa10_12_xor1 = (fa(((u_csamul_rca16_and10_12 >> 0) & 0x01), ((u_csamul_rca16_fa11_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_12_or0 = (fa(((u_csamul_rca16_and10_12 >> 0) & 0x01), ((u_csamul_rca16_fa11_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_12 = and_gate(((a >> 11) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa11_12_xor1 = (fa(((u_csamul_rca16_and11_12 >> 0) & 0x01), ((u_csamul_rca16_fa12_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_12_or0 = (fa(((u_csamul_rca16_and11_12 >> 0) & 0x01), ((u_csamul_rca16_fa12_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_12 = and_gate(((a >> 12) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa12_12_xor1 = (fa(((u_csamul_rca16_and12_12 >> 0) & 0x01), ((u_csamul_rca16_fa13_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_12_or0 = (fa(((u_csamul_rca16_and12_12 >> 0) & 0x01), ((u_csamul_rca16_fa13_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_12 = and_gate(((a >> 13) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa13_12_xor1 = (fa(((u_csamul_rca16_and13_12 >> 0) & 0x01), ((u_csamul_rca16_fa14_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_12_or0 = (fa(((u_csamul_rca16_and13_12 >> 0) & 0x01), ((u_csamul_rca16_fa14_11_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_12 = and_gate(((a >> 14) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_fa14_12_xor1 = (fa(((u_csamul_rca16_and14_12 >> 0) & 0x01), ((u_csamul_rca16_and15_11 >> 0) & 0x01), ((u_csamul_rca16_fa14_11_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_12_or0 = (fa(((u_csamul_rca16_and14_12 >> 0) & 0x01), ((u_csamul_rca16_and15_11 >> 0) & 0x01), ((u_csamul_rca16_fa14_11_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_12 = and_gate(((a >> 15) & 0x01), ((b >> 12) & 0x01));
|
|
u_csamul_rca16_and0_13 = and_gate(((a >> 0) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa0_13_xor1 = (fa(((u_csamul_rca16_and0_13 >> 0) & 0x01), ((u_csamul_rca16_fa1_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_13_or0 = (fa(((u_csamul_rca16_and0_13 >> 0) & 0x01), ((u_csamul_rca16_fa1_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_13 = and_gate(((a >> 1) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa1_13_xor1 = (fa(((u_csamul_rca16_and1_13 >> 0) & 0x01), ((u_csamul_rca16_fa2_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_13_or0 = (fa(((u_csamul_rca16_and1_13 >> 0) & 0x01), ((u_csamul_rca16_fa2_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_13 = and_gate(((a >> 2) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa2_13_xor1 = (fa(((u_csamul_rca16_and2_13 >> 0) & 0x01), ((u_csamul_rca16_fa3_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_13_or0 = (fa(((u_csamul_rca16_and2_13 >> 0) & 0x01), ((u_csamul_rca16_fa3_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_13 = and_gate(((a >> 3) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa3_13_xor1 = (fa(((u_csamul_rca16_and3_13 >> 0) & 0x01), ((u_csamul_rca16_fa4_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_13_or0 = (fa(((u_csamul_rca16_and3_13 >> 0) & 0x01), ((u_csamul_rca16_fa4_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_13 = and_gate(((a >> 4) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa4_13_xor1 = (fa(((u_csamul_rca16_and4_13 >> 0) & 0x01), ((u_csamul_rca16_fa5_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_13_or0 = (fa(((u_csamul_rca16_and4_13 >> 0) & 0x01), ((u_csamul_rca16_fa5_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_13 = and_gate(((a >> 5) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa5_13_xor1 = (fa(((u_csamul_rca16_and5_13 >> 0) & 0x01), ((u_csamul_rca16_fa6_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_13_or0 = (fa(((u_csamul_rca16_and5_13 >> 0) & 0x01), ((u_csamul_rca16_fa6_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_13 = and_gate(((a >> 6) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa6_13_xor1 = (fa(((u_csamul_rca16_and6_13 >> 0) & 0x01), ((u_csamul_rca16_fa7_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_13_or0 = (fa(((u_csamul_rca16_and6_13 >> 0) & 0x01), ((u_csamul_rca16_fa7_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_13 = and_gate(((a >> 7) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa7_13_xor1 = (fa(((u_csamul_rca16_and7_13 >> 0) & 0x01), ((u_csamul_rca16_fa8_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_13_or0 = (fa(((u_csamul_rca16_and7_13 >> 0) & 0x01), ((u_csamul_rca16_fa8_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_13 = and_gate(((a >> 8) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa8_13_xor1 = (fa(((u_csamul_rca16_and8_13 >> 0) & 0x01), ((u_csamul_rca16_fa9_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_13_or0 = (fa(((u_csamul_rca16_and8_13 >> 0) & 0x01), ((u_csamul_rca16_fa9_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_13 = and_gate(((a >> 9) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa9_13_xor1 = (fa(((u_csamul_rca16_and9_13 >> 0) & 0x01), ((u_csamul_rca16_fa10_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_13_or0 = (fa(((u_csamul_rca16_and9_13 >> 0) & 0x01), ((u_csamul_rca16_fa10_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_13 = and_gate(((a >> 10) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa10_13_xor1 = (fa(((u_csamul_rca16_and10_13 >> 0) & 0x01), ((u_csamul_rca16_fa11_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_13_or0 = (fa(((u_csamul_rca16_and10_13 >> 0) & 0x01), ((u_csamul_rca16_fa11_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_13 = and_gate(((a >> 11) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa11_13_xor1 = (fa(((u_csamul_rca16_and11_13 >> 0) & 0x01), ((u_csamul_rca16_fa12_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_13_or0 = (fa(((u_csamul_rca16_and11_13 >> 0) & 0x01), ((u_csamul_rca16_fa12_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_13 = and_gate(((a >> 12) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa12_13_xor1 = (fa(((u_csamul_rca16_and12_13 >> 0) & 0x01), ((u_csamul_rca16_fa13_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_13_or0 = (fa(((u_csamul_rca16_and12_13 >> 0) & 0x01), ((u_csamul_rca16_fa13_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_13 = and_gate(((a >> 13) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa13_13_xor1 = (fa(((u_csamul_rca16_and13_13 >> 0) & 0x01), ((u_csamul_rca16_fa14_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_13_or0 = (fa(((u_csamul_rca16_and13_13 >> 0) & 0x01), ((u_csamul_rca16_fa14_12_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_13 = and_gate(((a >> 14) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_fa14_13_xor1 = (fa(((u_csamul_rca16_and14_13 >> 0) & 0x01), ((u_csamul_rca16_and15_12 >> 0) & 0x01), ((u_csamul_rca16_fa14_12_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_13_or0 = (fa(((u_csamul_rca16_and14_13 >> 0) & 0x01), ((u_csamul_rca16_and15_12 >> 0) & 0x01), ((u_csamul_rca16_fa14_12_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_13 = and_gate(((a >> 15) & 0x01), ((b >> 13) & 0x01));
|
|
u_csamul_rca16_and0_14 = and_gate(((a >> 0) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa0_14_xor1 = (fa(((u_csamul_rca16_and0_14 >> 0) & 0x01), ((u_csamul_rca16_fa1_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_14_or0 = (fa(((u_csamul_rca16_and0_14 >> 0) & 0x01), ((u_csamul_rca16_fa1_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_14 = and_gate(((a >> 1) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa1_14_xor1 = (fa(((u_csamul_rca16_and1_14 >> 0) & 0x01), ((u_csamul_rca16_fa2_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_14_or0 = (fa(((u_csamul_rca16_and1_14 >> 0) & 0x01), ((u_csamul_rca16_fa2_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_14 = and_gate(((a >> 2) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa2_14_xor1 = (fa(((u_csamul_rca16_and2_14 >> 0) & 0x01), ((u_csamul_rca16_fa3_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_14_or0 = (fa(((u_csamul_rca16_and2_14 >> 0) & 0x01), ((u_csamul_rca16_fa3_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_14 = and_gate(((a >> 3) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa3_14_xor1 = (fa(((u_csamul_rca16_and3_14 >> 0) & 0x01), ((u_csamul_rca16_fa4_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_14_or0 = (fa(((u_csamul_rca16_and3_14 >> 0) & 0x01), ((u_csamul_rca16_fa4_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_14 = and_gate(((a >> 4) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa4_14_xor1 = (fa(((u_csamul_rca16_and4_14 >> 0) & 0x01), ((u_csamul_rca16_fa5_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_14_or0 = (fa(((u_csamul_rca16_and4_14 >> 0) & 0x01), ((u_csamul_rca16_fa5_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_14 = and_gate(((a >> 5) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa5_14_xor1 = (fa(((u_csamul_rca16_and5_14 >> 0) & 0x01), ((u_csamul_rca16_fa6_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_14_or0 = (fa(((u_csamul_rca16_and5_14 >> 0) & 0x01), ((u_csamul_rca16_fa6_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_14 = and_gate(((a >> 6) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa6_14_xor1 = (fa(((u_csamul_rca16_and6_14 >> 0) & 0x01), ((u_csamul_rca16_fa7_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_14_or0 = (fa(((u_csamul_rca16_and6_14 >> 0) & 0x01), ((u_csamul_rca16_fa7_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_14 = and_gate(((a >> 7) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa7_14_xor1 = (fa(((u_csamul_rca16_and7_14 >> 0) & 0x01), ((u_csamul_rca16_fa8_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_14_or0 = (fa(((u_csamul_rca16_and7_14 >> 0) & 0x01), ((u_csamul_rca16_fa8_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_14 = and_gate(((a >> 8) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa8_14_xor1 = (fa(((u_csamul_rca16_and8_14 >> 0) & 0x01), ((u_csamul_rca16_fa9_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_14_or0 = (fa(((u_csamul_rca16_and8_14 >> 0) & 0x01), ((u_csamul_rca16_fa9_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_14 = and_gate(((a >> 9) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa9_14_xor1 = (fa(((u_csamul_rca16_and9_14 >> 0) & 0x01), ((u_csamul_rca16_fa10_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_14_or0 = (fa(((u_csamul_rca16_and9_14 >> 0) & 0x01), ((u_csamul_rca16_fa10_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_14 = and_gate(((a >> 10) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa10_14_xor1 = (fa(((u_csamul_rca16_and10_14 >> 0) & 0x01), ((u_csamul_rca16_fa11_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_14_or0 = (fa(((u_csamul_rca16_and10_14 >> 0) & 0x01), ((u_csamul_rca16_fa11_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_14 = and_gate(((a >> 11) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa11_14_xor1 = (fa(((u_csamul_rca16_and11_14 >> 0) & 0x01), ((u_csamul_rca16_fa12_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_14_or0 = (fa(((u_csamul_rca16_and11_14 >> 0) & 0x01), ((u_csamul_rca16_fa12_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_14 = and_gate(((a >> 12) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa12_14_xor1 = (fa(((u_csamul_rca16_and12_14 >> 0) & 0x01), ((u_csamul_rca16_fa13_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_14_or0 = (fa(((u_csamul_rca16_and12_14 >> 0) & 0x01), ((u_csamul_rca16_fa13_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_14 = and_gate(((a >> 13) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa13_14_xor1 = (fa(((u_csamul_rca16_and13_14 >> 0) & 0x01), ((u_csamul_rca16_fa14_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_14_or0 = (fa(((u_csamul_rca16_and13_14 >> 0) & 0x01), ((u_csamul_rca16_fa14_13_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_14 = and_gate(((a >> 14) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_fa14_14_xor1 = (fa(((u_csamul_rca16_and14_14 >> 0) & 0x01), ((u_csamul_rca16_and15_13 >> 0) & 0x01), ((u_csamul_rca16_fa14_13_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_14_or0 = (fa(((u_csamul_rca16_and14_14 >> 0) & 0x01), ((u_csamul_rca16_and15_13 >> 0) & 0x01), ((u_csamul_rca16_fa14_13_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_14 = and_gate(((a >> 15) & 0x01), ((b >> 14) & 0x01));
|
|
u_csamul_rca16_and0_15 = and_gate(((a >> 0) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa0_15_xor1 = (fa(((u_csamul_rca16_and0_15 >> 0) & 0x01), ((u_csamul_rca16_fa1_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa0_15_or0 = (fa(((u_csamul_rca16_and0_15 >> 0) & 0x01), ((u_csamul_rca16_fa1_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa0_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and1_15 = and_gate(((a >> 1) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa1_15_xor1 = (fa(((u_csamul_rca16_and1_15 >> 0) & 0x01), ((u_csamul_rca16_fa2_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa1_15_or0 = (fa(((u_csamul_rca16_and1_15 >> 0) & 0x01), ((u_csamul_rca16_fa2_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa1_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and2_15 = and_gate(((a >> 2) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa2_15_xor1 = (fa(((u_csamul_rca16_and2_15 >> 0) & 0x01), ((u_csamul_rca16_fa3_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa2_15_or0 = (fa(((u_csamul_rca16_and2_15 >> 0) & 0x01), ((u_csamul_rca16_fa3_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa2_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and3_15 = and_gate(((a >> 3) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa3_15_xor1 = (fa(((u_csamul_rca16_and3_15 >> 0) & 0x01), ((u_csamul_rca16_fa4_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa3_15_or0 = (fa(((u_csamul_rca16_and3_15 >> 0) & 0x01), ((u_csamul_rca16_fa4_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa3_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and4_15 = and_gate(((a >> 4) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa4_15_xor1 = (fa(((u_csamul_rca16_and4_15 >> 0) & 0x01), ((u_csamul_rca16_fa5_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa4_15_or0 = (fa(((u_csamul_rca16_and4_15 >> 0) & 0x01), ((u_csamul_rca16_fa5_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa4_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and5_15 = and_gate(((a >> 5) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa5_15_xor1 = (fa(((u_csamul_rca16_and5_15 >> 0) & 0x01), ((u_csamul_rca16_fa6_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa5_15_or0 = (fa(((u_csamul_rca16_and5_15 >> 0) & 0x01), ((u_csamul_rca16_fa6_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa5_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and6_15 = and_gate(((a >> 6) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa6_15_xor1 = (fa(((u_csamul_rca16_and6_15 >> 0) & 0x01), ((u_csamul_rca16_fa7_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa6_15_or0 = (fa(((u_csamul_rca16_and6_15 >> 0) & 0x01), ((u_csamul_rca16_fa7_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa6_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and7_15 = and_gate(((a >> 7) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa7_15_xor1 = (fa(((u_csamul_rca16_and7_15 >> 0) & 0x01), ((u_csamul_rca16_fa8_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa7_15_or0 = (fa(((u_csamul_rca16_and7_15 >> 0) & 0x01), ((u_csamul_rca16_fa8_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa7_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and8_15 = and_gate(((a >> 8) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa8_15_xor1 = (fa(((u_csamul_rca16_and8_15 >> 0) & 0x01), ((u_csamul_rca16_fa9_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa8_15_or0 = (fa(((u_csamul_rca16_and8_15 >> 0) & 0x01), ((u_csamul_rca16_fa9_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa8_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and9_15 = and_gate(((a >> 9) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa9_15_xor1 = (fa(((u_csamul_rca16_and9_15 >> 0) & 0x01), ((u_csamul_rca16_fa10_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa9_15_or0 = (fa(((u_csamul_rca16_and9_15 >> 0) & 0x01), ((u_csamul_rca16_fa10_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa9_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and10_15 = and_gate(((a >> 10) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa10_15_xor1 = (fa(((u_csamul_rca16_and10_15 >> 0) & 0x01), ((u_csamul_rca16_fa11_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa10_15_or0 = (fa(((u_csamul_rca16_and10_15 >> 0) & 0x01), ((u_csamul_rca16_fa11_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa10_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and11_15 = and_gate(((a >> 11) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa11_15_xor1 = (fa(((u_csamul_rca16_and11_15 >> 0) & 0x01), ((u_csamul_rca16_fa12_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa11_15_or0 = (fa(((u_csamul_rca16_and11_15 >> 0) & 0x01), ((u_csamul_rca16_fa12_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa11_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and12_15 = and_gate(((a >> 12) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa12_15_xor1 = (fa(((u_csamul_rca16_and12_15 >> 0) & 0x01), ((u_csamul_rca16_fa13_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa12_15_or0 = (fa(((u_csamul_rca16_and12_15 >> 0) & 0x01), ((u_csamul_rca16_fa13_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa12_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and13_15 = and_gate(((a >> 13) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa13_15_xor1 = (fa(((u_csamul_rca16_and13_15 >> 0) & 0x01), ((u_csamul_rca16_fa14_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa13_15_or0 = (fa(((u_csamul_rca16_and13_15 >> 0) & 0x01), ((u_csamul_rca16_fa14_14_xor1 >> 0) & 0x01), ((u_csamul_rca16_fa13_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and14_15 = and_gate(((a >> 14) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_fa14_15_xor1 = (fa(((u_csamul_rca16_and14_15 >> 0) & 0x01), ((u_csamul_rca16_and15_14 >> 0) & 0x01), ((u_csamul_rca16_fa14_14_or0 >> 0) & 0x01)) >> 0) & 0x01;
|
|
u_csamul_rca16_fa14_15_or0 = (fa(((u_csamul_rca16_and14_15 >> 0) & 0x01), ((u_csamul_rca16_and15_14 >> 0) & 0x01), ((u_csamul_rca16_fa14_14_or0 >> 0) & 0x01)) >> 1) & 0x01;
|
|
u_csamul_rca16_and15_15 = and_gate(((a >> 15) & 0x01), ((b >> 15) & 0x01));
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa1_15_xor1 >> 0) & 0x01ull) << 0;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa2_15_xor1 >> 0) & 0x01ull) << 1;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa3_15_xor1 >> 0) & 0x01ull) << 2;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa4_15_xor1 >> 0) & 0x01ull) << 3;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa5_15_xor1 >> 0) & 0x01ull) << 4;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa6_15_xor1 >> 0) & 0x01ull) << 5;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa7_15_xor1 >> 0) & 0x01ull) << 6;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa8_15_xor1 >> 0) & 0x01ull) << 7;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa9_15_xor1 >> 0) & 0x01ull) << 8;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa10_15_xor1 >> 0) & 0x01ull) << 9;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa11_15_xor1 >> 0) & 0x01ull) << 10;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa12_15_xor1 >> 0) & 0x01ull) << 11;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa13_15_xor1 >> 0) & 0x01ull) << 12;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_fa14_15_xor1 >> 0) & 0x01ull) << 13;
|
|
u_csamul_rca16_u_rca16_a |= ((u_csamul_rca16_and15_15 >> 0) & 0x01ull) << 14;
|
|
u_csamul_rca16_u_rca16_a |= (0x00) << 15;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa0_15_or0 >> 0) & 0x01ull) << 0;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa1_15_or0 >> 0) & 0x01ull) << 1;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa2_15_or0 >> 0) & 0x01ull) << 2;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa3_15_or0 >> 0) & 0x01ull) << 3;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa4_15_or0 >> 0) & 0x01ull) << 4;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa5_15_or0 >> 0) & 0x01ull) << 5;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa6_15_or0 >> 0) & 0x01ull) << 6;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa7_15_or0 >> 0) & 0x01ull) << 7;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa8_15_or0 >> 0) & 0x01ull) << 8;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa9_15_or0 >> 0) & 0x01ull) << 9;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa10_15_or0 >> 0) & 0x01ull) << 10;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa11_15_or0 >> 0) & 0x01ull) << 11;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa12_15_or0 >> 0) & 0x01ull) << 12;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa13_15_or0 >> 0) & 0x01ull) << 13;
|
|
u_csamul_rca16_u_rca16_b |= ((u_csamul_rca16_fa14_15_or0 >> 0) & 0x01ull) << 14;
|
|
u_csamul_rca16_u_rca16_b |= (0x00) << 15;
|
|
u_csamul_rca16_u_rca16_out = u_rca16(u_csamul_rca16_u_rca16_a, u_csamul_rca16_u_rca16_b);
|
|
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_and0_0 >> 0) & 0x01ull) << 0;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_ha0_1_xor0 >> 0) & 0x01ull) << 1;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_2_xor1 >> 0) & 0x01ull) << 2;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_3_xor1 >> 0) & 0x01ull) << 3;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_4_xor1 >> 0) & 0x01ull) << 4;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_5_xor1 >> 0) & 0x01ull) << 5;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_6_xor1 >> 0) & 0x01ull) << 6;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_7_xor1 >> 0) & 0x01ull) << 7;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_8_xor1 >> 0) & 0x01ull) << 8;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_9_xor1 >> 0) & 0x01ull) << 9;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_10_xor1 >> 0) & 0x01ull) << 10;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_11_xor1 >> 0) & 0x01ull) << 11;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_12_xor1 >> 0) & 0x01ull) << 12;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_13_xor1 >> 0) & 0x01ull) << 13;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_14_xor1 >> 0) & 0x01ull) << 14;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_fa0_15_xor1 >> 0) & 0x01ull) << 15;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 0) & 0x01ull) << 16;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 1) & 0x01ull) << 17;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 2) & 0x01ull) << 18;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 3) & 0x01ull) << 19;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 4) & 0x01ull) << 20;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 5) & 0x01ull) << 21;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 6) & 0x01ull) << 22;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 7) & 0x01ull) << 23;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 8) & 0x01ull) << 24;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 9) & 0x01ull) << 25;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 10) & 0x01ull) << 26;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 11) & 0x01ull) << 27;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 12) & 0x01ull) << 28;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 13) & 0x01ull) << 29;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 14) & 0x01ull) << 30;
|
|
u_csamul_rca16_out |= ((u_csamul_rca16_u_rca16_out >> 15) & 0x01ull) << 31;
|
|
return u_csamul_rca16_out;
|
|
} |