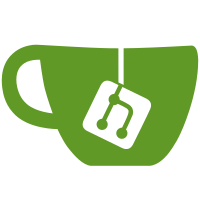
* #10 CGP Circuits as inputs (#11) * CGP Circuits as inputs * #10 support of signed output in general circuit * input as output works * output connected to input (c) * automated verilog testing * output rename * Implemented CSA and Wallace tree multiplier composing of CSAs. Also did some code cleanup. * Typos fix and code cleanup. * Added new (approximate) multiplier architectures and did some minor changes regarding sign extension for c output formats. * Updated automated testing scripts. * Small bugfix in python code generation (I initially thought this line is useless). * Updated generated circuits folder. Co-authored-by: Vojta Mrazek <mrazek@fit.vutbr.cz>
1569 lines
115 KiB
C
1569 lines
115 KiB
C
#include <stdio.h>
|
|
#include <stdint.h>
|
|
|
|
uint64_t u_wallace_rca12(uint64_t a, uint64_t b){
|
|
uint64_t u_wallace_rca12_out = 0;
|
|
uint8_t u_wallace_rca12_and_2_0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_1 = 0;
|
|
uint8_t u_wallace_rca12_ha0_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha0_and0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_0 = 0;
|
|
uint8_t u_wallace_rca12_and_2_1 = 0;
|
|
uint8_t u_wallace_rca12_fa0_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa0_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa0_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa0_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa0_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_4_0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_1 = 0;
|
|
uint8_t u_wallace_rca12_fa1_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa1_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa1_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa1_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa1_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_0 = 0;
|
|
uint8_t u_wallace_rca12_and_4_1 = 0;
|
|
uint8_t u_wallace_rca12_fa2_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa2_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa2_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa2_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa2_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_6_0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_1 = 0;
|
|
uint8_t u_wallace_rca12_fa3_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa3_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa3_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa3_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa3_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_0 = 0;
|
|
uint8_t u_wallace_rca12_and_6_1 = 0;
|
|
uint8_t u_wallace_rca12_fa4_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa4_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa4_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa4_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa4_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_8_0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_1 = 0;
|
|
uint8_t u_wallace_rca12_fa5_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa5_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa5_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa5_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa5_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_0 = 0;
|
|
uint8_t u_wallace_rca12_and_8_1 = 0;
|
|
uint8_t u_wallace_rca12_fa6_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa6_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa6_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa6_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa6_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_10_0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_1 = 0;
|
|
uint8_t u_wallace_rca12_fa7_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa7_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa7_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa7_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa7_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_0 = 0;
|
|
uint8_t u_wallace_rca12_and_10_1 = 0;
|
|
uint8_t u_wallace_rca12_fa8_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa8_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa8_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa8_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa8_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_1 = 0;
|
|
uint8_t u_wallace_rca12_and_10_2 = 0;
|
|
uint8_t u_wallace_rca12_fa9_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa9_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa9_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa9_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa9_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_2 = 0;
|
|
uint8_t u_wallace_rca12_and_10_3 = 0;
|
|
uint8_t u_wallace_rca12_fa10_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa10_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa10_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa10_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa10_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_3 = 0;
|
|
uint8_t u_wallace_rca12_and_10_4 = 0;
|
|
uint8_t u_wallace_rca12_fa11_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa11_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa11_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa11_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa11_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_4 = 0;
|
|
uint8_t u_wallace_rca12_and_10_5 = 0;
|
|
uint8_t u_wallace_rca12_fa12_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa12_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa12_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa12_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa12_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_5 = 0;
|
|
uint8_t u_wallace_rca12_and_10_6 = 0;
|
|
uint8_t u_wallace_rca12_fa13_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa13_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa13_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa13_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa13_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_6 = 0;
|
|
uint8_t u_wallace_rca12_and_10_7 = 0;
|
|
uint8_t u_wallace_rca12_fa14_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa14_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa14_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa14_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa14_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_7 = 0;
|
|
uint8_t u_wallace_rca12_and_10_8 = 0;
|
|
uint8_t u_wallace_rca12_fa15_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa15_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa15_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa15_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa15_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_8 = 0;
|
|
uint8_t u_wallace_rca12_and_10_9 = 0;
|
|
uint8_t u_wallace_rca12_fa16_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa16_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa16_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa16_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa16_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_9 = 0;
|
|
uint8_t u_wallace_rca12_and_10_10 = 0;
|
|
uint8_t u_wallace_rca12_fa17_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa17_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa17_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa17_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa17_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_2 = 0;
|
|
uint8_t u_wallace_rca12_and_0_3 = 0;
|
|
uint8_t u_wallace_rca12_ha1_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha1_and0 = 0;
|
|
uint8_t u_wallace_rca12_and_2_2 = 0;
|
|
uint8_t u_wallace_rca12_and_1_3 = 0;
|
|
uint8_t u_wallace_rca12_fa18_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa18_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa18_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa18_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa18_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_2 = 0;
|
|
uint8_t u_wallace_rca12_and_2_3 = 0;
|
|
uint8_t u_wallace_rca12_fa19_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa19_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa19_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa19_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa19_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_4_2 = 0;
|
|
uint8_t u_wallace_rca12_and_3_3 = 0;
|
|
uint8_t u_wallace_rca12_fa20_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa20_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa20_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa20_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa20_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_2 = 0;
|
|
uint8_t u_wallace_rca12_and_4_3 = 0;
|
|
uint8_t u_wallace_rca12_fa21_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa21_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa21_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa21_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa21_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_6_2 = 0;
|
|
uint8_t u_wallace_rca12_and_5_3 = 0;
|
|
uint8_t u_wallace_rca12_fa22_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa22_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa22_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa22_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa22_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_2 = 0;
|
|
uint8_t u_wallace_rca12_and_6_3 = 0;
|
|
uint8_t u_wallace_rca12_fa23_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa23_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa23_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa23_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa23_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_8_2 = 0;
|
|
uint8_t u_wallace_rca12_and_7_3 = 0;
|
|
uint8_t u_wallace_rca12_fa24_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa24_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa24_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa24_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa24_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_2 = 0;
|
|
uint8_t u_wallace_rca12_and_8_3 = 0;
|
|
uint8_t u_wallace_rca12_fa25_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa25_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa25_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa25_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa25_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_3 = 0;
|
|
uint8_t u_wallace_rca12_and_8_4 = 0;
|
|
uint8_t u_wallace_rca12_fa26_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa26_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa26_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa26_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa26_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_4 = 0;
|
|
uint8_t u_wallace_rca12_and_8_5 = 0;
|
|
uint8_t u_wallace_rca12_fa27_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa27_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa27_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa27_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa27_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_5 = 0;
|
|
uint8_t u_wallace_rca12_and_8_6 = 0;
|
|
uint8_t u_wallace_rca12_fa28_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa28_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa28_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa28_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa28_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_6 = 0;
|
|
uint8_t u_wallace_rca12_and_8_7 = 0;
|
|
uint8_t u_wallace_rca12_fa29_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa29_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa29_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa29_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa29_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_7 = 0;
|
|
uint8_t u_wallace_rca12_and_8_8 = 0;
|
|
uint8_t u_wallace_rca12_fa30_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa30_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa30_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa30_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa30_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_8 = 0;
|
|
uint8_t u_wallace_rca12_and_8_9 = 0;
|
|
uint8_t u_wallace_rca12_fa31_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa31_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa31_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa31_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa31_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_9 = 0;
|
|
uint8_t u_wallace_rca12_and_8_10 = 0;
|
|
uint8_t u_wallace_rca12_fa32_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa32_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa32_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa32_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa32_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_10 = 0;
|
|
uint8_t u_wallace_rca12_and_8_11 = 0;
|
|
uint8_t u_wallace_rca12_fa33_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa33_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa33_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa33_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa33_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_0_4 = 0;
|
|
uint8_t u_wallace_rca12_ha2_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha2_and0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_4 = 0;
|
|
uint8_t u_wallace_rca12_and_0_5 = 0;
|
|
uint8_t u_wallace_rca12_fa34_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa34_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa34_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa34_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa34_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_2_4 = 0;
|
|
uint8_t u_wallace_rca12_and_1_5 = 0;
|
|
uint8_t u_wallace_rca12_fa35_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa35_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa35_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa35_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa35_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_4 = 0;
|
|
uint8_t u_wallace_rca12_and_2_5 = 0;
|
|
uint8_t u_wallace_rca12_fa36_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa36_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa36_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa36_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa36_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_4_4 = 0;
|
|
uint8_t u_wallace_rca12_and_3_5 = 0;
|
|
uint8_t u_wallace_rca12_fa37_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa37_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa37_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa37_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa37_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_4 = 0;
|
|
uint8_t u_wallace_rca12_and_4_5 = 0;
|
|
uint8_t u_wallace_rca12_fa38_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa38_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa38_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa38_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa38_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_6_4 = 0;
|
|
uint8_t u_wallace_rca12_and_5_5 = 0;
|
|
uint8_t u_wallace_rca12_fa39_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa39_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa39_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa39_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa39_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_4 = 0;
|
|
uint8_t u_wallace_rca12_and_6_5 = 0;
|
|
uint8_t u_wallace_rca12_fa40_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa40_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa40_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa40_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa40_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_5 = 0;
|
|
uint8_t u_wallace_rca12_and_6_6 = 0;
|
|
uint8_t u_wallace_rca12_fa41_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa41_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa41_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa41_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa41_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_6 = 0;
|
|
uint8_t u_wallace_rca12_and_6_7 = 0;
|
|
uint8_t u_wallace_rca12_fa42_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa42_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa42_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa42_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa42_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_7 = 0;
|
|
uint8_t u_wallace_rca12_and_6_8 = 0;
|
|
uint8_t u_wallace_rca12_fa43_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa43_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa43_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa43_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa43_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_8 = 0;
|
|
uint8_t u_wallace_rca12_and_6_9 = 0;
|
|
uint8_t u_wallace_rca12_fa44_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa44_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa44_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa44_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa44_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_9 = 0;
|
|
uint8_t u_wallace_rca12_and_6_10 = 0;
|
|
uint8_t u_wallace_rca12_fa45_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa45_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa45_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa45_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa45_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_10 = 0;
|
|
uint8_t u_wallace_rca12_and_6_11 = 0;
|
|
uint8_t u_wallace_rca12_fa46_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa46_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa46_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa46_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa46_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_7_11 = 0;
|
|
uint8_t u_wallace_rca12_fa47_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa47_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa47_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa47_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa47_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha3_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha3_and0 = 0;
|
|
uint8_t u_wallace_rca12_and_0_6 = 0;
|
|
uint8_t u_wallace_rca12_fa48_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa48_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa48_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa48_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa48_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_6 = 0;
|
|
uint8_t u_wallace_rca12_and_0_7 = 0;
|
|
uint8_t u_wallace_rca12_fa49_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa49_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa49_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa49_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa49_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_2_6 = 0;
|
|
uint8_t u_wallace_rca12_and_1_7 = 0;
|
|
uint8_t u_wallace_rca12_fa50_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa50_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa50_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa50_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa50_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_6 = 0;
|
|
uint8_t u_wallace_rca12_and_2_7 = 0;
|
|
uint8_t u_wallace_rca12_fa51_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa51_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa51_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa51_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa51_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_4_6 = 0;
|
|
uint8_t u_wallace_rca12_and_3_7 = 0;
|
|
uint8_t u_wallace_rca12_fa52_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa52_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa52_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa52_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa52_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_6 = 0;
|
|
uint8_t u_wallace_rca12_and_4_7 = 0;
|
|
uint8_t u_wallace_rca12_fa53_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa53_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa53_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa53_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa53_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_7 = 0;
|
|
uint8_t u_wallace_rca12_and_4_8 = 0;
|
|
uint8_t u_wallace_rca12_fa54_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa54_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa54_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa54_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa54_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_8 = 0;
|
|
uint8_t u_wallace_rca12_and_4_9 = 0;
|
|
uint8_t u_wallace_rca12_fa55_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa55_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa55_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa55_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa55_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_9 = 0;
|
|
uint8_t u_wallace_rca12_and_4_10 = 0;
|
|
uint8_t u_wallace_rca12_fa56_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa56_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa56_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa56_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa56_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_10 = 0;
|
|
uint8_t u_wallace_rca12_and_4_11 = 0;
|
|
uint8_t u_wallace_rca12_fa57_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa57_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa57_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa57_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa57_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_5_11 = 0;
|
|
uint8_t u_wallace_rca12_fa58_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa58_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa58_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa58_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa58_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa59_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa59_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa59_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa59_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa59_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha4_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha4_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa60_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa60_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa60_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa60_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa60_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_0_8 = 0;
|
|
uint8_t u_wallace_rca12_fa61_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa61_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa61_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa61_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa61_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_8 = 0;
|
|
uint8_t u_wallace_rca12_and_0_9 = 0;
|
|
uint8_t u_wallace_rca12_fa62_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa62_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa62_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa62_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa62_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_2_8 = 0;
|
|
uint8_t u_wallace_rca12_and_1_9 = 0;
|
|
uint8_t u_wallace_rca12_fa63_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa63_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa63_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa63_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa63_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_8 = 0;
|
|
uint8_t u_wallace_rca12_and_2_9 = 0;
|
|
uint8_t u_wallace_rca12_fa64_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa64_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa64_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa64_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa64_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_9 = 0;
|
|
uint8_t u_wallace_rca12_and_2_10 = 0;
|
|
uint8_t u_wallace_rca12_fa65_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa65_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa65_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa65_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa65_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_10 = 0;
|
|
uint8_t u_wallace_rca12_and_2_11 = 0;
|
|
uint8_t u_wallace_rca12_fa66_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa66_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa66_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa66_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa66_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_3_11 = 0;
|
|
uint8_t u_wallace_rca12_fa67_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa67_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa67_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa67_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa67_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa68_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa68_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa68_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa68_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa68_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa69_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa69_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa69_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa69_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa69_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha5_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha5_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa70_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa70_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa70_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa70_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa70_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa71_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa71_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa71_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa71_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa71_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_0_10 = 0;
|
|
uint8_t u_wallace_rca12_fa72_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa72_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa72_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa72_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa72_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_10 = 0;
|
|
uint8_t u_wallace_rca12_and_0_11 = 0;
|
|
uint8_t u_wallace_rca12_fa73_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa73_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa73_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa73_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa73_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_11 = 0;
|
|
uint8_t u_wallace_rca12_fa74_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa74_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa74_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa74_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa74_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa75_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa75_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa75_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa75_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa75_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa76_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa76_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa76_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa76_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa76_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa77_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa77_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa77_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa77_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa77_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha6_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha6_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa78_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa78_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa78_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa78_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa78_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa79_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa79_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa79_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa79_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa79_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa80_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa80_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa80_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa80_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa80_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa81_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa81_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa81_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa81_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa81_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa82_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa82_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa82_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa82_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa82_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa83_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa83_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa83_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa83_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa83_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha7_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha7_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa84_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa84_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa84_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa84_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa84_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa85_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa85_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa85_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa85_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa85_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa86_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa86_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa86_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa86_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa86_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa87_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa87_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa87_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa87_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa87_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha8_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha8_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa88_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa88_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa88_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa88_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa88_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa89_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa89_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa89_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa89_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa89_or0 = 0;
|
|
uint8_t u_wallace_rca12_ha9_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha9_and0 = 0;
|
|
uint8_t u_wallace_rca12_ha10_xor0 = 0;
|
|
uint8_t u_wallace_rca12_ha10_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa90_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa90_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa90_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa90_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa90_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa91_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa91_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa91_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa91_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa91_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa92_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa92_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa92_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa92_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa92_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa93_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa93_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa93_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa93_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa93_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa94_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa94_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa94_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa94_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa94_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa95_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa95_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa95_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa95_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa95_or0 = 0;
|
|
uint8_t u_wallace_rca12_fa96_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa96_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa96_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa96_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa96_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_9_11 = 0;
|
|
uint8_t u_wallace_rca12_fa97_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa97_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa97_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa97_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa97_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_11_10 = 0;
|
|
uint8_t u_wallace_rca12_fa98_xor0 = 0;
|
|
uint8_t u_wallace_rca12_fa98_and0 = 0;
|
|
uint8_t u_wallace_rca12_fa98_xor1 = 0;
|
|
uint8_t u_wallace_rca12_fa98_and1 = 0;
|
|
uint8_t u_wallace_rca12_fa98_or0 = 0;
|
|
uint8_t u_wallace_rca12_and_0_0 = 0;
|
|
uint8_t u_wallace_rca12_and_1_0 = 0;
|
|
uint8_t u_wallace_rca12_and_0_2 = 0;
|
|
uint8_t u_wallace_rca12_and_10_11 = 0;
|
|
uint8_t u_wallace_rca12_and_0_1 = 0;
|
|
uint8_t u_wallace_rca12_and_11_11 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_ha_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_ha_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa1_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa1_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa1_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa1_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa1_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa2_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa2_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa2_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa2_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa2_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa3_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa3_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa3_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa3_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa3_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa4_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa4_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa4_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa4_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa4_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa5_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa5_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa5_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa5_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa5_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa6_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa6_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa6_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa6_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa6_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa7_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa7_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa7_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa7_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa7_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa8_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa8_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa8_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa8_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa8_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa9_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa9_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa9_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa9_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa9_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa10_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa10_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa10_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa10_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa10_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa11_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa11_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa11_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa11_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa11_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa12_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa12_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa12_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa12_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa12_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa13_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa13_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa13_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa13_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa13_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa14_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa14_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa14_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa14_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa14_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa15_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa15_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa15_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa15_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa15_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa16_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa16_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa16_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa16_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa16_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa17_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa17_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa17_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa17_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa17_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa18_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa18_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa18_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa18_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa18_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa19_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa19_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa19_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa19_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa19_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa20_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa20_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa20_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa20_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa20_or0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa21_xor0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa21_and0 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa21_xor1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa21_and1 = 0;
|
|
uint8_t u_wallace_rca12_u_rca22_fa21_or0 = 0;
|
|
|
|
u_wallace_rca12_and_2_0 = ((a >> 2) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_1 = ((a >> 1) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_ha0_xor0 = ((u_wallace_rca12_and_2_0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha0_and0 = ((u_wallace_rca12_and_2_0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_0 = ((a >> 3) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_2_1 = ((a >> 2) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa0_xor0 = ((u_wallace_rca12_ha0_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa0_and0 = ((u_wallace_rca12_ha0_and0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa0_xor1 = ((u_wallace_rca12_fa0_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa0_and1 = ((u_wallace_rca12_fa0_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa0_or0 = ((u_wallace_rca12_fa0_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa0_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_4_0 = ((a >> 4) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_1 = ((a >> 3) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa1_xor0 = ((u_wallace_rca12_fa0_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa1_and0 = ((u_wallace_rca12_fa0_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa1_xor1 = ((u_wallace_rca12_fa1_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa1_and1 = ((u_wallace_rca12_fa1_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa1_or0 = ((u_wallace_rca12_fa1_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa1_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_0 = ((a >> 5) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_4_1 = ((a >> 4) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa2_xor0 = ((u_wallace_rca12_fa1_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa2_and0 = ((u_wallace_rca12_fa1_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa2_xor1 = ((u_wallace_rca12_fa2_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa2_and1 = ((u_wallace_rca12_fa2_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa2_or0 = ((u_wallace_rca12_fa2_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa2_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_6_0 = ((a >> 6) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_1 = ((a >> 5) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa3_xor0 = ((u_wallace_rca12_fa2_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa3_and0 = ((u_wallace_rca12_fa2_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa3_xor1 = ((u_wallace_rca12_fa3_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa3_and1 = ((u_wallace_rca12_fa3_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa3_or0 = ((u_wallace_rca12_fa3_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa3_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_0 = ((a >> 7) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_6_1 = ((a >> 6) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa4_xor0 = ((u_wallace_rca12_fa3_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa4_and0 = ((u_wallace_rca12_fa3_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa4_xor1 = ((u_wallace_rca12_fa4_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa4_and1 = ((u_wallace_rca12_fa4_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa4_or0 = ((u_wallace_rca12_fa4_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa4_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_8_0 = ((a >> 8) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_1 = ((a >> 7) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa5_xor0 = ((u_wallace_rca12_fa4_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa5_and0 = ((u_wallace_rca12_fa4_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa5_xor1 = ((u_wallace_rca12_fa5_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa5_and1 = ((u_wallace_rca12_fa5_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa5_or0 = ((u_wallace_rca12_fa5_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa5_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_0 = ((a >> 9) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_8_1 = ((a >> 8) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa6_xor0 = ((u_wallace_rca12_fa5_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa6_and0 = ((u_wallace_rca12_fa5_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa6_xor1 = ((u_wallace_rca12_fa6_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa6_and1 = ((u_wallace_rca12_fa6_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa6_or0 = ((u_wallace_rca12_fa6_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa6_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_10_0 = ((a >> 10) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_1 = ((a >> 9) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa7_xor0 = ((u_wallace_rca12_fa6_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa7_and0 = ((u_wallace_rca12_fa6_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa7_xor1 = ((u_wallace_rca12_fa7_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa7_and1 = ((u_wallace_rca12_fa7_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa7_or0 = ((u_wallace_rca12_fa7_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa7_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_0 = ((a >> 11) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_10_1 = ((a >> 10) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_fa8_xor0 = ((u_wallace_rca12_fa7_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa8_and0 = ((u_wallace_rca12_fa7_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa8_xor1 = ((u_wallace_rca12_fa8_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa8_and1 = ((u_wallace_rca12_fa8_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa8_or0 = ((u_wallace_rca12_fa8_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa8_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_1 = ((a >> 11) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_and_10_2 = ((a >> 10) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_fa9_xor0 = ((u_wallace_rca12_fa8_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa9_and0 = ((u_wallace_rca12_fa8_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa9_xor1 = ((u_wallace_rca12_fa9_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa9_and1 = ((u_wallace_rca12_fa9_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa9_or0 = ((u_wallace_rca12_fa9_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa9_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_2 = ((a >> 11) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_10_3 = ((a >> 10) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa10_xor0 = ((u_wallace_rca12_fa9_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa10_and0 = ((u_wallace_rca12_fa9_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa10_xor1 = ((u_wallace_rca12_fa10_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa10_and1 = ((u_wallace_rca12_fa10_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa10_or0 = ((u_wallace_rca12_fa10_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa10_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_3 = ((a >> 11) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_and_10_4 = ((a >> 10) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_fa11_xor0 = ((u_wallace_rca12_fa10_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa11_and0 = ((u_wallace_rca12_fa10_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa11_xor1 = ((u_wallace_rca12_fa11_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa11_and1 = ((u_wallace_rca12_fa11_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa11_or0 = ((u_wallace_rca12_fa11_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa11_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_4 = ((a >> 11) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_10_5 = ((a >> 10) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa12_xor0 = ((u_wallace_rca12_fa11_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa12_and0 = ((u_wallace_rca12_fa11_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa12_xor1 = ((u_wallace_rca12_fa12_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa12_and1 = ((u_wallace_rca12_fa12_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa12_or0 = ((u_wallace_rca12_fa12_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa12_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_5 = ((a >> 11) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_and_10_6 = ((a >> 10) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_fa13_xor0 = ((u_wallace_rca12_fa12_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa13_and0 = ((u_wallace_rca12_fa12_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa13_xor1 = ((u_wallace_rca12_fa13_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa13_and1 = ((u_wallace_rca12_fa13_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa13_or0 = ((u_wallace_rca12_fa13_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa13_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_6 = ((a >> 11) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_10_7 = ((a >> 10) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa14_xor0 = ((u_wallace_rca12_fa13_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa14_and0 = ((u_wallace_rca12_fa13_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa14_xor1 = ((u_wallace_rca12_fa14_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa14_and1 = ((u_wallace_rca12_fa14_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa14_or0 = ((u_wallace_rca12_fa14_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa14_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_7 = ((a >> 11) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_and_10_8 = ((a >> 10) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_fa15_xor0 = ((u_wallace_rca12_fa14_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa15_and0 = ((u_wallace_rca12_fa14_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa15_xor1 = ((u_wallace_rca12_fa15_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa15_and1 = ((u_wallace_rca12_fa15_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa15_or0 = ((u_wallace_rca12_fa15_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa15_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_8 = ((a >> 11) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_10_9 = ((a >> 10) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa16_xor0 = ((u_wallace_rca12_fa15_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa16_and0 = ((u_wallace_rca12_fa15_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa16_xor1 = ((u_wallace_rca12_fa16_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa16_and1 = ((u_wallace_rca12_fa16_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa16_or0 = ((u_wallace_rca12_fa16_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa16_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_9 = ((a >> 11) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_and_10_10 = ((a >> 10) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa17_xor0 = ((u_wallace_rca12_fa16_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa17_and0 = ((u_wallace_rca12_fa16_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa17_xor1 = ((u_wallace_rca12_fa17_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_10_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa17_and1 = ((u_wallace_rca12_fa17_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_10_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa17_or0 = ((u_wallace_rca12_fa17_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa17_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_2 = ((a >> 1) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_0_3 = ((a >> 0) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_ha1_xor0 = ((u_wallace_rca12_and_1_2 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_3 >> 0) & 0x01);
|
|
u_wallace_rca12_ha1_and0 = ((u_wallace_rca12_and_1_2 >> 0) & 0x01) & ((u_wallace_rca12_and_0_3 >> 0) & 0x01);
|
|
u_wallace_rca12_and_2_2 = ((a >> 2) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_1_3 = ((a >> 1) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa18_xor0 = ((u_wallace_rca12_ha1_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa18_and0 = ((u_wallace_rca12_ha1_and0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa18_xor1 = ((u_wallace_rca12_fa18_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa18_and1 = ((u_wallace_rca12_fa18_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa18_or0 = ((u_wallace_rca12_fa18_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa18_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_2 = ((a >> 3) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_2_3 = ((a >> 2) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa19_xor0 = ((u_wallace_rca12_fa18_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa19_and0 = ((u_wallace_rca12_fa18_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa19_xor1 = ((u_wallace_rca12_fa19_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa19_and1 = ((u_wallace_rca12_fa19_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa19_or0 = ((u_wallace_rca12_fa19_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa19_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_4_2 = ((a >> 4) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_3_3 = ((a >> 3) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa20_xor0 = ((u_wallace_rca12_fa19_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa20_and0 = ((u_wallace_rca12_fa19_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa20_xor1 = ((u_wallace_rca12_fa20_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa20_and1 = ((u_wallace_rca12_fa20_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa20_or0 = ((u_wallace_rca12_fa20_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa20_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_2 = ((a >> 5) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_4_3 = ((a >> 4) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa21_xor0 = ((u_wallace_rca12_fa20_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa21_and0 = ((u_wallace_rca12_fa20_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa21_xor1 = ((u_wallace_rca12_fa21_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa21_and1 = ((u_wallace_rca12_fa21_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa21_or0 = ((u_wallace_rca12_fa21_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa21_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_6_2 = ((a >> 6) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_5_3 = ((a >> 5) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa22_xor0 = ((u_wallace_rca12_fa21_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa22_and0 = ((u_wallace_rca12_fa21_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa22_xor1 = ((u_wallace_rca12_fa22_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa22_and1 = ((u_wallace_rca12_fa22_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa22_or0 = ((u_wallace_rca12_fa22_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa22_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_2 = ((a >> 7) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_6_3 = ((a >> 6) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa23_xor0 = ((u_wallace_rca12_fa22_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa23_and0 = ((u_wallace_rca12_fa22_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa23_xor1 = ((u_wallace_rca12_fa23_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa23_and1 = ((u_wallace_rca12_fa23_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa23_or0 = ((u_wallace_rca12_fa23_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa23_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_8_2 = ((a >> 8) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_7_3 = ((a >> 7) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa24_xor0 = ((u_wallace_rca12_fa23_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa24_and0 = ((u_wallace_rca12_fa23_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa24_xor1 = ((u_wallace_rca12_fa24_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa24_and1 = ((u_wallace_rca12_fa24_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa24_or0 = ((u_wallace_rca12_fa24_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa24_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_2 = ((a >> 9) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_8_3 = ((a >> 8) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_fa25_xor0 = ((u_wallace_rca12_fa24_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa25_and0 = ((u_wallace_rca12_fa24_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_2 >> 0) & 0x01);
|
|
u_wallace_rca12_fa25_xor1 = ((u_wallace_rca12_fa25_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa25_and1 = ((u_wallace_rca12_fa25_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa25_or0 = ((u_wallace_rca12_fa25_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa25_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_3 = ((a >> 9) & 0x01) & ((b >> 3) & 0x01);
|
|
u_wallace_rca12_and_8_4 = ((a >> 8) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_fa26_xor0 = ((u_wallace_rca12_fa25_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa26_and0 = ((u_wallace_rca12_fa25_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_3 >> 0) & 0x01);
|
|
u_wallace_rca12_fa26_xor1 = ((u_wallace_rca12_fa26_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa26_and1 = ((u_wallace_rca12_fa26_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa26_or0 = ((u_wallace_rca12_fa26_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa26_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_4 = ((a >> 9) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_8_5 = ((a >> 8) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa27_xor0 = ((u_wallace_rca12_fa26_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa27_and0 = ((u_wallace_rca12_fa26_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa27_xor1 = ((u_wallace_rca12_fa27_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa27_and1 = ((u_wallace_rca12_fa27_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa27_or0 = ((u_wallace_rca12_fa27_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa27_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_5 = ((a >> 9) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_and_8_6 = ((a >> 8) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_fa28_xor0 = ((u_wallace_rca12_fa27_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa28_and0 = ((u_wallace_rca12_fa27_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa28_xor1 = ((u_wallace_rca12_fa28_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa28_and1 = ((u_wallace_rca12_fa28_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa28_or0 = ((u_wallace_rca12_fa28_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa28_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_6 = ((a >> 9) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_8_7 = ((a >> 8) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa29_xor0 = ((u_wallace_rca12_fa28_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa29_and0 = ((u_wallace_rca12_fa28_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa29_xor1 = ((u_wallace_rca12_fa29_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa29_and1 = ((u_wallace_rca12_fa29_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa29_or0 = ((u_wallace_rca12_fa29_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa29_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_7 = ((a >> 9) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_and_8_8 = ((a >> 8) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_fa30_xor0 = ((u_wallace_rca12_fa29_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa30_and0 = ((u_wallace_rca12_fa29_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa30_xor1 = ((u_wallace_rca12_fa30_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa30_and1 = ((u_wallace_rca12_fa30_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa30_or0 = ((u_wallace_rca12_fa30_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa30_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_8 = ((a >> 9) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_8_9 = ((a >> 8) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa31_xor0 = ((u_wallace_rca12_fa30_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa31_and0 = ((u_wallace_rca12_fa30_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa31_xor1 = ((u_wallace_rca12_fa31_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa31_and1 = ((u_wallace_rca12_fa31_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa31_or0 = ((u_wallace_rca12_fa31_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa31_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_9 = ((a >> 9) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_and_8_10 = ((a >> 8) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa32_xor0 = ((u_wallace_rca12_fa31_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa32_and0 = ((u_wallace_rca12_fa31_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa32_xor1 = ((u_wallace_rca12_fa32_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa32_and1 = ((u_wallace_rca12_fa32_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa32_or0 = ((u_wallace_rca12_fa32_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa32_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_10 = ((a >> 9) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_and_8_11 = ((a >> 8) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa33_xor0 = ((u_wallace_rca12_fa32_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa33_and0 = ((u_wallace_rca12_fa32_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa33_xor1 = ((u_wallace_rca12_fa33_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_8_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa33_and1 = ((u_wallace_rca12_fa33_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_8_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa33_or0 = ((u_wallace_rca12_fa33_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa33_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_0_4 = ((a >> 0) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_ha2_xor0 = ((u_wallace_rca12_and_0_4 >> 0) & 0x01) ^ ((u_wallace_rca12_fa1_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha2_and0 = ((u_wallace_rca12_and_0_4 >> 0) & 0x01) & ((u_wallace_rca12_fa1_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_4 = ((a >> 1) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_0_5 = ((a >> 0) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa34_xor0 = ((u_wallace_rca12_ha2_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa34_and0 = ((u_wallace_rca12_ha2_and0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa34_xor1 = ((u_wallace_rca12_fa34_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa34_and1 = ((u_wallace_rca12_fa34_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa34_or0 = ((u_wallace_rca12_fa34_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa34_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_2_4 = ((a >> 2) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_1_5 = ((a >> 1) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa35_xor0 = ((u_wallace_rca12_fa34_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa35_and0 = ((u_wallace_rca12_fa34_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa35_xor1 = ((u_wallace_rca12_fa35_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa35_and1 = ((u_wallace_rca12_fa35_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa35_or0 = ((u_wallace_rca12_fa35_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa35_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_4 = ((a >> 3) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_2_5 = ((a >> 2) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa36_xor0 = ((u_wallace_rca12_fa35_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa36_and0 = ((u_wallace_rca12_fa35_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa36_xor1 = ((u_wallace_rca12_fa36_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa36_and1 = ((u_wallace_rca12_fa36_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa36_or0 = ((u_wallace_rca12_fa36_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa36_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_4_4 = ((a >> 4) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_3_5 = ((a >> 3) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa37_xor0 = ((u_wallace_rca12_fa36_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa37_and0 = ((u_wallace_rca12_fa36_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa37_xor1 = ((u_wallace_rca12_fa37_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa37_and1 = ((u_wallace_rca12_fa37_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa37_or0 = ((u_wallace_rca12_fa37_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa37_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_4 = ((a >> 5) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_4_5 = ((a >> 4) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa38_xor0 = ((u_wallace_rca12_fa37_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa38_and0 = ((u_wallace_rca12_fa37_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa38_xor1 = ((u_wallace_rca12_fa38_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa38_and1 = ((u_wallace_rca12_fa38_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa38_or0 = ((u_wallace_rca12_fa38_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa38_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_6_4 = ((a >> 6) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_5_5 = ((a >> 5) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa39_xor0 = ((u_wallace_rca12_fa38_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa39_and0 = ((u_wallace_rca12_fa38_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa39_xor1 = ((u_wallace_rca12_fa39_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa39_and1 = ((u_wallace_rca12_fa39_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa39_or0 = ((u_wallace_rca12_fa39_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa39_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_4 = ((a >> 7) & 0x01) & ((b >> 4) & 0x01);
|
|
u_wallace_rca12_and_6_5 = ((a >> 6) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_fa40_xor0 = ((u_wallace_rca12_fa39_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa40_and0 = ((u_wallace_rca12_fa39_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_4 >> 0) & 0x01);
|
|
u_wallace_rca12_fa40_xor1 = ((u_wallace_rca12_fa40_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa40_and1 = ((u_wallace_rca12_fa40_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa40_or0 = ((u_wallace_rca12_fa40_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa40_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_5 = ((a >> 7) & 0x01) & ((b >> 5) & 0x01);
|
|
u_wallace_rca12_and_6_6 = ((a >> 6) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_fa41_xor0 = ((u_wallace_rca12_fa40_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa41_and0 = ((u_wallace_rca12_fa40_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_5 >> 0) & 0x01);
|
|
u_wallace_rca12_fa41_xor1 = ((u_wallace_rca12_fa41_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa41_and1 = ((u_wallace_rca12_fa41_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa41_or0 = ((u_wallace_rca12_fa41_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa41_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_6 = ((a >> 7) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_6_7 = ((a >> 6) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa42_xor0 = ((u_wallace_rca12_fa41_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa42_and0 = ((u_wallace_rca12_fa41_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa42_xor1 = ((u_wallace_rca12_fa42_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa42_and1 = ((u_wallace_rca12_fa42_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa42_or0 = ((u_wallace_rca12_fa42_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa42_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_7 = ((a >> 7) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_and_6_8 = ((a >> 6) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_fa43_xor0 = ((u_wallace_rca12_fa42_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa43_and0 = ((u_wallace_rca12_fa42_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa43_xor1 = ((u_wallace_rca12_fa43_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa43_and1 = ((u_wallace_rca12_fa43_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa43_or0 = ((u_wallace_rca12_fa43_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa43_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_8 = ((a >> 7) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_6_9 = ((a >> 6) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa44_xor0 = ((u_wallace_rca12_fa43_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa44_and0 = ((u_wallace_rca12_fa43_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa44_xor1 = ((u_wallace_rca12_fa44_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa44_and1 = ((u_wallace_rca12_fa44_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa44_or0 = ((u_wallace_rca12_fa44_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa44_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_9 = ((a >> 7) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_and_6_10 = ((a >> 6) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa45_xor0 = ((u_wallace_rca12_fa44_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa45_and0 = ((u_wallace_rca12_fa44_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa45_xor1 = ((u_wallace_rca12_fa45_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa45_and1 = ((u_wallace_rca12_fa45_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa45_or0 = ((u_wallace_rca12_fa45_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa45_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_10 = ((a >> 7) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_and_6_11 = ((a >> 6) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa46_xor0 = ((u_wallace_rca12_fa45_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa46_and0 = ((u_wallace_rca12_fa45_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa46_xor1 = ((u_wallace_rca12_fa46_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_6_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa46_and1 = ((u_wallace_rca12_fa46_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_6_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa46_or0 = ((u_wallace_rca12_fa46_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa46_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_7_11 = ((a >> 7) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa47_xor0 = ((u_wallace_rca12_fa46_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_7_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa47_and0 = ((u_wallace_rca12_fa46_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_7_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa47_xor1 = ((u_wallace_rca12_fa47_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa15_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa47_and1 = ((u_wallace_rca12_fa47_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa15_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa47_or0 = ((u_wallace_rca12_fa47_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa47_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha3_xor0 = ((u_wallace_rca12_fa2_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa19_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha3_and0 = ((u_wallace_rca12_fa2_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa19_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_0_6 = ((a >> 0) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_fa48_xor0 = ((u_wallace_rca12_ha3_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa48_and0 = ((u_wallace_rca12_ha3_and0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa48_xor1 = ((u_wallace_rca12_fa48_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa3_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa48_and1 = ((u_wallace_rca12_fa48_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa3_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa48_or0 = ((u_wallace_rca12_fa48_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa48_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_6 = ((a >> 1) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_0_7 = ((a >> 0) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa49_xor0 = ((u_wallace_rca12_fa48_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa49_and0 = ((u_wallace_rca12_fa48_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa49_xor1 = ((u_wallace_rca12_fa49_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa49_and1 = ((u_wallace_rca12_fa49_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa49_or0 = ((u_wallace_rca12_fa49_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa49_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_2_6 = ((a >> 2) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_1_7 = ((a >> 1) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa50_xor0 = ((u_wallace_rca12_fa49_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa50_and0 = ((u_wallace_rca12_fa49_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa50_xor1 = ((u_wallace_rca12_fa50_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa50_and1 = ((u_wallace_rca12_fa50_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa50_or0 = ((u_wallace_rca12_fa50_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa50_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_6 = ((a >> 3) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_2_7 = ((a >> 2) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa51_xor0 = ((u_wallace_rca12_fa50_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa51_and0 = ((u_wallace_rca12_fa50_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa51_xor1 = ((u_wallace_rca12_fa51_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa51_and1 = ((u_wallace_rca12_fa51_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa51_or0 = ((u_wallace_rca12_fa51_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa51_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_4_6 = ((a >> 4) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_3_7 = ((a >> 3) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa52_xor0 = ((u_wallace_rca12_fa51_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa52_and0 = ((u_wallace_rca12_fa51_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa52_xor1 = ((u_wallace_rca12_fa52_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa52_and1 = ((u_wallace_rca12_fa52_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa52_or0 = ((u_wallace_rca12_fa52_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa52_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_6 = ((a >> 5) & 0x01) & ((b >> 6) & 0x01);
|
|
u_wallace_rca12_and_4_7 = ((a >> 4) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_fa53_xor0 = ((u_wallace_rca12_fa52_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa53_and0 = ((u_wallace_rca12_fa52_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_6 >> 0) & 0x01);
|
|
u_wallace_rca12_fa53_xor1 = ((u_wallace_rca12_fa53_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa53_and1 = ((u_wallace_rca12_fa53_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa53_or0 = ((u_wallace_rca12_fa53_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa53_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_7 = ((a >> 5) & 0x01) & ((b >> 7) & 0x01);
|
|
u_wallace_rca12_and_4_8 = ((a >> 4) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_fa54_xor0 = ((u_wallace_rca12_fa53_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa54_and0 = ((u_wallace_rca12_fa53_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_7 >> 0) & 0x01);
|
|
u_wallace_rca12_fa54_xor1 = ((u_wallace_rca12_fa54_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa54_and1 = ((u_wallace_rca12_fa54_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa54_or0 = ((u_wallace_rca12_fa54_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa54_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_8 = ((a >> 5) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_4_9 = ((a >> 4) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa55_xor0 = ((u_wallace_rca12_fa54_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa55_and0 = ((u_wallace_rca12_fa54_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa55_xor1 = ((u_wallace_rca12_fa55_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa55_and1 = ((u_wallace_rca12_fa55_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa55_or0 = ((u_wallace_rca12_fa55_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa55_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_9 = ((a >> 5) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_and_4_10 = ((a >> 4) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa56_xor0 = ((u_wallace_rca12_fa55_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa56_and0 = ((u_wallace_rca12_fa55_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa56_xor1 = ((u_wallace_rca12_fa56_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa56_and1 = ((u_wallace_rca12_fa56_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa56_or0 = ((u_wallace_rca12_fa56_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa56_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_10 = ((a >> 5) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_and_4_11 = ((a >> 4) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa57_xor0 = ((u_wallace_rca12_fa56_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa57_and0 = ((u_wallace_rca12_fa56_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa57_xor1 = ((u_wallace_rca12_fa57_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_4_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa57_and1 = ((u_wallace_rca12_fa57_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_4_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa57_or0 = ((u_wallace_rca12_fa57_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa57_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_5_11 = ((a >> 5) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa58_xor0 = ((u_wallace_rca12_fa57_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_5_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa58_and0 = ((u_wallace_rca12_fa57_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_5_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa58_xor1 = ((u_wallace_rca12_fa58_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa13_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa58_and1 = ((u_wallace_rca12_fa58_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa13_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa58_or0 = ((u_wallace_rca12_fa58_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa58_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa59_xor0 = ((u_wallace_rca12_fa58_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa14_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa59_and0 = ((u_wallace_rca12_fa58_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa14_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa59_xor1 = ((u_wallace_rca12_fa59_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa31_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa59_and1 = ((u_wallace_rca12_fa59_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa31_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa59_or0 = ((u_wallace_rca12_fa59_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa59_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha4_xor0 = ((u_wallace_rca12_fa20_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa35_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha4_and0 = ((u_wallace_rca12_fa20_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa35_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa60_xor0 = ((u_wallace_rca12_ha4_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa4_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa60_and0 = ((u_wallace_rca12_ha4_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa4_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa60_xor1 = ((u_wallace_rca12_fa60_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa21_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa60_and1 = ((u_wallace_rca12_fa60_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa21_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa60_or0 = ((u_wallace_rca12_fa60_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa60_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_0_8 = ((a >> 0) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_fa61_xor0 = ((u_wallace_rca12_fa60_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa61_and0 = ((u_wallace_rca12_fa60_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa61_xor1 = ((u_wallace_rca12_fa61_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa5_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa61_and1 = ((u_wallace_rca12_fa61_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa5_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa61_or0 = ((u_wallace_rca12_fa61_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa61_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_8 = ((a >> 1) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_0_9 = ((a >> 0) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa62_xor0 = ((u_wallace_rca12_fa61_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa62_and0 = ((u_wallace_rca12_fa61_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa62_xor1 = ((u_wallace_rca12_fa62_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa62_and1 = ((u_wallace_rca12_fa62_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa62_or0 = ((u_wallace_rca12_fa62_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa62_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_2_8 = ((a >> 2) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_1_9 = ((a >> 1) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa63_xor0 = ((u_wallace_rca12_fa62_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa63_and0 = ((u_wallace_rca12_fa62_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa63_xor1 = ((u_wallace_rca12_fa63_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa63_and1 = ((u_wallace_rca12_fa63_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa63_or0 = ((u_wallace_rca12_fa63_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa63_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_8 = ((a >> 3) & 0x01) & ((b >> 8) & 0x01);
|
|
u_wallace_rca12_and_2_9 = ((a >> 2) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_fa64_xor0 = ((u_wallace_rca12_fa63_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa64_and0 = ((u_wallace_rca12_fa63_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_8 >> 0) & 0x01);
|
|
u_wallace_rca12_fa64_xor1 = ((u_wallace_rca12_fa64_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa64_and1 = ((u_wallace_rca12_fa64_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa64_or0 = ((u_wallace_rca12_fa64_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa64_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_9 = ((a >> 3) & 0x01) & ((b >> 9) & 0x01);
|
|
u_wallace_rca12_and_2_10 = ((a >> 2) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa65_xor0 = ((u_wallace_rca12_fa64_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa65_and0 = ((u_wallace_rca12_fa64_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_9 >> 0) & 0x01);
|
|
u_wallace_rca12_fa65_xor1 = ((u_wallace_rca12_fa65_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa65_and1 = ((u_wallace_rca12_fa65_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa65_or0 = ((u_wallace_rca12_fa65_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa65_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_10 = ((a >> 3) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_and_2_11 = ((a >> 2) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa66_xor0 = ((u_wallace_rca12_fa65_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa66_and0 = ((u_wallace_rca12_fa65_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa66_xor1 = ((u_wallace_rca12_fa66_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_2_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa66_and1 = ((u_wallace_rca12_fa66_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_2_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa66_or0 = ((u_wallace_rca12_fa66_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa66_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_3_11 = ((a >> 3) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa67_xor0 = ((u_wallace_rca12_fa66_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_3_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa67_and0 = ((u_wallace_rca12_fa66_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_3_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa67_xor1 = ((u_wallace_rca12_fa67_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa11_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa67_and1 = ((u_wallace_rca12_fa67_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa11_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa67_or0 = ((u_wallace_rca12_fa67_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa67_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa68_xor0 = ((u_wallace_rca12_fa67_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa12_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa68_and0 = ((u_wallace_rca12_fa67_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa12_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa68_xor1 = ((u_wallace_rca12_fa68_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa29_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa68_and1 = ((u_wallace_rca12_fa68_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa29_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa68_or0 = ((u_wallace_rca12_fa68_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa68_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa69_xor0 = ((u_wallace_rca12_fa68_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa30_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa69_and0 = ((u_wallace_rca12_fa68_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa30_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa69_xor1 = ((u_wallace_rca12_fa69_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa45_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa69_and1 = ((u_wallace_rca12_fa69_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa45_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa69_or0 = ((u_wallace_rca12_fa69_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa69_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha5_xor0 = ((u_wallace_rca12_fa36_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa49_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha5_and0 = ((u_wallace_rca12_fa36_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa49_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa70_xor0 = ((u_wallace_rca12_ha5_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa22_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa70_and0 = ((u_wallace_rca12_ha5_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa22_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa70_xor1 = ((u_wallace_rca12_fa70_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa37_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa70_and1 = ((u_wallace_rca12_fa70_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa37_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa70_or0 = ((u_wallace_rca12_fa70_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa70_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa71_xor0 = ((u_wallace_rca12_fa70_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa6_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa71_and0 = ((u_wallace_rca12_fa70_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa6_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa71_xor1 = ((u_wallace_rca12_fa71_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa23_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa71_and1 = ((u_wallace_rca12_fa71_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa23_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa71_or0 = ((u_wallace_rca12_fa71_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa71_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_0_10 = ((a >> 0) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa72_xor0 = ((u_wallace_rca12_fa71_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa72_and0 = ((u_wallace_rca12_fa71_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa72_xor1 = ((u_wallace_rca12_fa72_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa7_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa72_and1 = ((u_wallace_rca12_fa72_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa7_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa72_or0 = ((u_wallace_rca12_fa72_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa72_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_10 = ((a >> 1) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_and_0_11 = ((a >> 0) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa73_xor0 = ((u_wallace_rca12_fa72_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa73_and0 = ((u_wallace_rca12_fa72_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa73_xor1 = ((u_wallace_rca12_fa73_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa73_and1 = ((u_wallace_rca12_fa73_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa73_or0 = ((u_wallace_rca12_fa73_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa73_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_11 = ((a >> 1) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa74_xor0 = ((u_wallace_rca12_fa73_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_1_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa74_and0 = ((u_wallace_rca12_fa73_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_1_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa74_xor1 = ((u_wallace_rca12_fa74_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa9_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa74_and1 = ((u_wallace_rca12_fa74_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa9_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa74_or0 = ((u_wallace_rca12_fa74_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa74_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa75_xor0 = ((u_wallace_rca12_fa74_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa10_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa75_and0 = ((u_wallace_rca12_fa74_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa10_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa75_xor1 = ((u_wallace_rca12_fa75_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa27_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa75_and1 = ((u_wallace_rca12_fa75_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa27_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa75_or0 = ((u_wallace_rca12_fa75_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa75_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa76_xor0 = ((u_wallace_rca12_fa75_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa28_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa76_and0 = ((u_wallace_rca12_fa75_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa28_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa76_xor1 = ((u_wallace_rca12_fa76_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa43_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa76_and1 = ((u_wallace_rca12_fa76_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa43_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa76_or0 = ((u_wallace_rca12_fa76_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa76_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa77_xor0 = ((u_wallace_rca12_fa76_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa44_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa77_and0 = ((u_wallace_rca12_fa76_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa44_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa77_xor1 = ((u_wallace_rca12_fa77_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa57_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa77_and1 = ((u_wallace_rca12_fa77_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa57_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa77_or0 = ((u_wallace_rca12_fa77_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa77_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha6_xor0 = ((u_wallace_rca12_fa50_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa61_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha6_and0 = ((u_wallace_rca12_fa50_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa61_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa78_xor0 = ((u_wallace_rca12_ha6_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa38_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa78_and0 = ((u_wallace_rca12_ha6_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa38_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa78_xor1 = ((u_wallace_rca12_fa78_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa51_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa78_and1 = ((u_wallace_rca12_fa78_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa51_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa78_or0 = ((u_wallace_rca12_fa78_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa78_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa79_xor0 = ((u_wallace_rca12_fa78_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa24_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa79_and0 = ((u_wallace_rca12_fa78_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa24_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa79_xor1 = ((u_wallace_rca12_fa79_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa39_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa79_and1 = ((u_wallace_rca12_fa79_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa39_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa79_or0 = ((u_wallace_rca12_fa79_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa79_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa80_xor0 = ((u_wallace_rca12_fa79_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa8_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa80_and0 = ((u_wallace_rca12_fa79_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa8_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa80_xor1 = ((u_wallace_rca12_fa80_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa25_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa80_and1 = ((u_wallace_rca12_fa80_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa25_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa80_or0 = ((u_wallace_rca12_fa80_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa80_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa81_xor0 = ((u_wallace_rca12_fa80_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa26_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa81_and0 = ((u_wallace_rca12_fa80_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa26_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa81_xor1 = ((u_wallace_rca12_fa81_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa41_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa81_and1 = ((u_wallace_rca12_fa81_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa41_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa81_or0 = ((u_wallace_rca12_fa81_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa81_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa82_xor0 = ((u_wallace_rca12_fa81_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa42_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa82_and0 = ((u_wallace_rca12_fa81_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa42_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa82_xor1 = ((u_wallace_rca12_fa82_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa55_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa82_and1 = ((u_wallace_rca12_fa82_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa55_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa82_or0 = ((u_wallace_rca12_fa82_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa82_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa83_xor0 = ((u_wallace_rca12_fa82_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa56_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa83_and0 = ((u_wallace_rca12_fa82_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa56_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa83_xor1 = ((u_wallace_rca12_fa83_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa67_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa83_and1 = ((u_wallace_rca12_fa83_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa67_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa83_or0 = ((u_wallace_rca12_fa83_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa83_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha7_xor0 = ((u_wallace_rca12_fa62_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa71_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha7_and0 = ((u_wallace_rca12_fa62_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa71_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa84_xor0 = ((u_wallace_rca12_ha7_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa52_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa84_and0 = ((u_wallace_rca12_ha7_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa52_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa84_xor1 = ((u_wallace_rca12_fa84_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa63_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa84_and1 = ((u_wallace_rca12_fa84_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa63_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa84_or0 = ((u_wallace_rca12_fa84_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa84_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa85_xor0 = ((u_wallace_rca12_fa84_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa40_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa85_and0 = ((u_wallace_rca12_fa84_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa40_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa85_xor1 = ((u_wallace_rca12_fa85_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa53_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa85_and1 = ((u_wallace_rca12_fa85_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa53_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa85_or0 = ((u_wallace_rca12_fa85_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa85_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa86_xor0 = ((u_wallace_rca12_fa85_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa54_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa86_and0 = ((u_wallace_rca12_fa85_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa54_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa86_xor1 = ((u_wallace_rca12_fa86_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa65_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa86_and1 = ((u_wallace_rca12_fa86_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa65_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa86_or0 = ((u_wallace_rca12_fa86_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa86_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa87_xor0 = ((u_wallace_rca12_fa86_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa66_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa87_and0 = ((u_wallace_rca12_fa86_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa66_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa87_xor1 = ((u_wallace_rca12_fa87_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa75_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa87_and1 = ((u_wallace_rca12_fa87_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa75_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa87_or0 = ((u_wallace_rca12_fa87_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa87_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha8_xor0 = ((u_wallace_rca12_fa72_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa79_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha8_and0 = ((u_wallace_rca12_fa72_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa79_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa88_xor0 = ((u_wallace_rca12_ha8_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa64_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa88_and0 = ((u_wallace_rca12_ha8_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa64_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa88_xor1 = ((u_wallace_rca12_fa88_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa73_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa88_and1 = ((u_wallace_rca12_fa88_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa73_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa88_or0 = ((u_wallace_rca12_fa88_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa88_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa89_xor0 = ((u_wallace_rca12_fa88_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa74_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa89_and0 = ((u_wallace_rca12_fa88_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa74_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa89_xor1 = ((u_wallace_rca12_fa89_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa81_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa89_and1 = ((u_wallace_rca12_fa89_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa81_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa89_or0 = ((u_wallace_rca12_fa89_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa89_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha9_xor0 = ((u_wallace_rca12_fa80_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa85_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha9_and0 = ((u_wallace_rca12_fa80_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa85_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha10_xor0 = ((u_wallace_rca12_ha9_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa86_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_ha10_and0 = ((u_wallace_rca12_ha9_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa86_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa90_xor0 = ((u_wallace_rca12_ha10_and0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa89_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa90_and0 = ((u_wallace_rca12_ha10_and0 >> 0) & 0x01) & ((u_wallace_rca12_fa89_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa90_xor1 = ((u_wallace_rca12_fa90_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa82_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa90_and1 = ((u_wallace_rca12_fa90_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa82_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa90_or0 = ((u_wallace_rca12_fa90_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa90_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa91_xor0 = ((u_wallace_rca12_fa90_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa87_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa91_and0 = ((u_wallace_rca12_fa90_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa87_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa91_xor1 = ((u_wallace_rca12_fa91_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa76_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa91_and1 = ((u_wallace_rca12_fa91_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa76_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa91_or0 = ((u_wallace_rca12_fa91_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa91_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa92_xor0 = ((u_wallace_rca12_fa91_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa83_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa92_and0 = ((u_wallace_rca12_fa91_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa83_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa92_xor1 = ((u_wallace_rca12_fa92_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa68_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa92_and1 = ((u_wallace_rca12_fa92_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa68_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa92_or0 = ((u_wallace_rca12_fa92_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa92_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa93_xor0 = ((u_wallace_rca12_fa92_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa77_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa93_and0 = ((u_wallace_rca12_fa92_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa77_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa93_xor1 = ((u_wallace_rca12_fa93_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa58_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa93_and1 = ((u_wallace_rca12_fa93_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa58_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa93_or0 = ((u_wallace_rca12_fa93_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa93_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa94_xor0 = ((u_wallace_rca12_fa93_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa69_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa94_and0 = ((u_wallace_rca12_fa93_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa69_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa94_xor1 = ((u_wallace_rca12_fa94_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa46_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa94_and1 = ((u_wallace_rca12_fa94_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa46_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa94_or0 = ((u_wallace_rca12_fa94_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa94_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa95_xor0 = ((u_wallace_rca12_fa94_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa59_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa95_and0 = ((u_wallace_rca12_fa94_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa59_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa95_xor1 = ((u_wallace_rca12_fa95_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa32_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa95_and1 = ((u_wallace_rca12_fa95_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa32_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa95_or0 = ((u_wallace_rca12_fa95_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa95_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa96_xor0 = ((u_wallace_rca12_fa95_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa47_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa96_and0 = ((u_wallace_rca12_fa95_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa47_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa96_xor1 = ((u_wallace_rca12_fa96_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa16_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa96_and1 = ((u_wallace_rca12_fa96_xor0 >> 0) & 0x01) & ((u_wallace_rca12_fa16_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_fa96_or0 = ((u_wallace_rca12_fa96_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa96_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_9_11 = ((a >> 9) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_fa97_xor0 = ((u_wallace_rca12_fa96_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa33_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa97_and0 = ((u_wallace_rca12_fa96_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa33_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa97_xor1 = ((u_wallace_rca12_fa97_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_9_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa97_and1 = ((u_wallace_rca12_fa97_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_9_11 >> 0) & 0x01);
|
|
u_wallace_rca12_fa97_or0 = ((u_wallace_rca12_fa97_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa97_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_11_10 = ((a >> 11) & 0x01) & ((b >> 10) & 0x01);
|
|
u_wallace_rca12_fa98_xor0 = ((u_wallace_rca12_fa97_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_fa17_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa98_and0 = ((u_wallace_rca12_fa97_or0 >> 0) & 0x01) & ((u_wallace_rca12_fa17_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_fa98_xor1 = ((u_wallace_rca12_fa98_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa98_and1 = ((u_wallace_rca12_fa98_xor0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_10 >> 0) & 0x01);
|
|
u_wallace_rca12_fa98_or0 = ((u_wallace_rca12_fa98_and0 >> 0) & 0x01) | ((u_wallace_rca12_fa98_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_and_0_0 = ((a >> 0) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_1_0 = ((a >> 1) & 0x01) & ((b >> 0) & 0x01);
|
|
u_wallace_rca12_and_0_2 = ((a >> 0) & 0x01) & ((b >> 2) & 0x01);
|
|
u_wallace_rca12_and_10_11 = ((a >> 10) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_and_0_1 = ((a >> 0) & 0x01) & ((b >> 1) & 0x01);
|
|
u_wallace_rca12_and_11_11 = ((a >> 11) & 0x01) & ((b >> 11) & 0x01);
|
|
u_wallace_rca12_u_rca22_ha_xor0 = ((u_wallace_rca12_and_1_0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_0_1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_ha_and0 = ((u_wallace_rca12_and_1_0 >> 0) & 0x01) & ((u_wallace_rca12_and_0_1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa1_xor0 = ((u_wallace_rca12_and_0_2 >> 0) & 0x01) ^ ((u_wallace_rca12_ha0_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa1_and0 = ((u_wallace_rca12_and_0_2 >> 0) & 0x01) & ((u_wallace_rca12_ha0_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa1_xor1 = ((u_wallace_rca12_u_rca22_fa1_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_ha_and0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa1_and1 = ((u_wallace_rca12_u_rca22_fa1_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_ha_and0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa1_or0 = ((u_wallace_rca12_u_rca22_fa1_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa1_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa2_xor0 = ((u_wallace_rca12_fa0_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha1_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa2_and0 = ((u_wallace_rca12_fa0_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha1_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa2_xor1 = ((u_wallace_rca12_u_rca22_fa2_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa1_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa2_and1 = ((u_wallace_rca12_u_rca22_fa2_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa1_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa2_or0 = ((u_wallace_rca12_u_rca22_fa2_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa2_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa3_xor0 = ((u_wallace_rca12_fa18_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha2_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa3_and0 = ((u_wallace_rca12_fa18_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha2_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa3_xor1 = ((u_wallace_rca12_u_rca22_fa3_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa2_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa3_and1 = ((u_wallace_rca12_u_rca22_fa3_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa2_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa3_or0 = ((u_wallace_rca12_u_rca22_fa3_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa3_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa4_xor0 = ((u_wallace_rca12_fa34_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha3_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa4_and0 = ((u_wallace_rca12_fa34_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha3_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa4_xor1 = ((u_wallace_rca12_u_rca22_fa4_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa3_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa4_and1 = ((u_wallace_rca12_u_rca22_fa4_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa3_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa4_or0 = ((u_wallace_rca12_u_rca22_fa4_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa4_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa5_xor0 = ((u_wallace_rca12_fa48_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha4_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa5_and0 = ((u_wallace_rca12_fa48_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha4_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa5_xor1 = ((u_wallace_rca12_u_rca22_fa5_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa4_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa5_and1 = ((u_wallace_rca12_u_rca22_fa5_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa4_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa5_or0 = ((u_wallace_rca12_u_rca22_fa5_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa5_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa6_xor0 = ((u_wallace_rca12_fa60_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha5_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa6_and0 = ((u_wallace_rca12_fa60_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha5_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa6_xor1 = ((u_wallace_rca12_u_rca22_fa6_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa5_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa6_and1 = ((u_wallace_rca12_u_rca22_fa6_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa5_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa6_or0 = ((u_wallace_rca12_u_rca22_fa6_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa6_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa7_xor0 = ((u_wallace_rca12_fa70_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha6_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa7_and0 = ((u_wallace_rca12_fa70_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha6_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa7_xor1 = ((u_wallace_rca12_u_rca22_fa7_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa6_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa7_and1 = ((u_wallace_rca12_u_rca22_fa7_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa6_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa7_or0 = ((u_wallace_rca12_u_rca22_fa7_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa7_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa8_xor0 = ((u_wallace_rca12_fa78_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha7_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa8_and0 = ((u_wallace_rca12_fa78_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha7_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa8_xor1 = ((u_wallace_rca12_u_rca22_fa8_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa7_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa8_and1 = ((u_wallace_rca12_u_rca22_fa8_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa7_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa8_or0 = ((u_wallace_rca12_u_rca22_fa8_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa8_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa9_xor0 = ((u_wallace_rca12_fa84_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha8_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa9_and0 = ((u_wallace_rca12_fa84_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha8_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa9_xor1 = ((u_wallace_rca12_u_rca22_fa9_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa8_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa9_and1 = ((u_wallace_rca12_u_rca22_fa9_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa8_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa9_or0 = ((u_wallace_rca12_u_rca22_fa9_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa9_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa10_xor0 = ((u_wallace_rca12_fa88_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha9_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa10_and0 = ((u_wallace_rca12_fa88_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha9_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa10_xor1 = ((u_wallace_rca12_u_rca22_fa10_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa9_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa10_and1 = ((u_wallace_rca12_u_rca22_fa10_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa9_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa10_or0 = ((u_wallace_rca12_u_rca22_fa10_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa10_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa11_xor0 = ((u_wallace_rca12_fa89_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_ha10_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa11_and0 = ((u_wallace_rca12_fa89_xor1 >> 0) & 0x01) & ((u_wallace_rca12_ha10_xor0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa11_xor1 = ((u_wallace_rca12_u_rca22_fa11_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa10_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa11_and1 = ((u_wallace_rca12_u_rca22_fa11_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa10_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa11_or0 = ((u_wallace_rca12_u_rca22_fa11_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa11_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa12_xor0 = ((u_wallace_rca12_fa87_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa90_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa12_and0 = ((u_wallace_rca12_fa87_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa90_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa12_xor1 = ((u_wallace_rca12_u_rca22_fa12_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa11_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa12_and1 = ((u_wallace_rca12_u_rca22_fa12_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa11_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa12_or0 = ((u_wallace_rca12_u_rca22_fa12_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa12_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa13_xor0 = ((u_wallace_rca12_fa83_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa91_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa13_and0 = ((u_wallace_rca12_fa83_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa91_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa13_xor1 = ((u_wallace_rca12_u_rca22_fa13_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa12_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa13_and1 = ((u_wallace_rca12_u_rca22_fa13_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa12_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa13_or0 = ((u_wallace_rca12_u_rca22_fa13_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa13_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa14_xor0 = ((u_wallace_rca12_fa77_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa92_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa14_and0 = ((u_wallace_rca12_fa77_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa92_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa14_xor1 = ((u_wallace_rca12_u_rca22_fa14_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa13_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa14_and1 = ((u_wallace_rca12_u_rca22_fa14_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa13_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa14_or0 = ((u_wallace_rca12_u_rca22_fa14_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa14_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa15_xor0 = ((u_wallace_rca12_fa69_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa93_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa15_and0 = ((u_wallace_rca12_fa69_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa93_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa15_xor1 = ((u_wallace_rca12_u_rca22_fa15_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa14_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa15_and1 = ((u_wallace_rca12_u_rca22_fa15_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa14_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa15_or0 = ((u_wallace_rca12_u_rca22_fa15_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa15_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa16_xor0 = ((u_wallace_rca12_fa59_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa94_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa16_and0 = ((u_wallace_rca12_fa59_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa94_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa16_xor1 = ((u_wallace_rca12_u_rca22_fa16_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa15_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa16_and1 = ((u_wallace_rca12_u_rca22_fa16_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa15_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa16_or0 = ((u_wallace_rca12_u_rca22_fa16_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa16_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa17_xor0 = ((u_wallace_rca12_fa47_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa95_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa17_and0 = ((u_wallace_rca12_fa47_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa95_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa17_xor1 = ((u_wallace_rca12_u_rca22_fa17_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa16_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa17_and1 = ((u_wallace_rca12_u_rca22_fa17_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa16_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa17_or0 = ((u_wallace_rca12_u_rca22_fa17_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa17_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa18_xor0 = ((u_wallace_rca12_fa33_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa96_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa18_and0 = ((u_wallace_rca12_fa33_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa96_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa18_xor1 = ((u_wallace_rca12_u_rca22_fa18_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa17_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa18_and1 = ((u_wallace_rca12_u_rca22_fa18_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa17_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa18_or0 = ((u_wallace_rca12_u_rca22_fa18_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa18_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa19_xor0 = ((u_wallace_rca12_fa17_xor1 >> 0) & 0x01) ^ ((u_wallace_rca12_fa97_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa19_and0 = ((u_wallace_rca12_fa17_xor1 >> 0) & 0x01) & ((u_wallace_rca12_fa97_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa19_xor1 = ((u_wallace_rca12_u_rca22_fa19_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa18_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa19_and1 = ((u_wallace_rca12_u_rca22_fa19_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa18_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa19_or0 = ((u_wallace_rca12_u_rca22_fa19_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa19_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa20_xor0 = ((u_wallace_rca12_and_10_11 >> 0) & 0x01) ^ ((u_wallace_rca12_fa98_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa20_and0 = ((u_wallace_rca12_and_10_11 >> 0) & 0x01) & ((u_wallace_rca12_fa98_xor1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa20_xor1 = ((u_wallace_rca12_u_rca22_fa20_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa19_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa20_and1 = ((u_wallace_rca12_u_rca22_fa20_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa19_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa20_or0 = ((u_wallace_rca12_u_rca22_fa20_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa20_and1 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa21_xor0 = ((u_wallace_rca12_fa98_or0 >> 0) & 0x01) ^ ((u_wallace_rca12_and_11_11 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa21_and0 = ((u_wallace_rca12_fa98_or0 >> 0) & 0x01) & ((u_wallace_rca12_and_11_11 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa21_xor1 = ((u_wallace_rca12_u_rca22_fa21_xor0 >> 0) & 0x01) ^ ((u_wallace_rca12_u_rca22_fa20_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa21_and1 = ((u_wallace_rca12_u_rca22_fa21_xor0 >> 0) & 0x01) & ((u_wallace_rca12_u_rca22_fa20_or0 >> 0) & 0x01);
|
|
u_wallace_rca12_u_rca22_fa21_or0 = ((u_wallace_rca12_u_rca22_fa21_and0 >> 0) & 0x01) | ((u_wallace_rca12_u_rca22_fa21_and1 >> 0) & 0x01);
|
|
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_and_0_0 >> 0) & 0x01ull) << 0;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_ha_xor0 >> 0) & 0x01ull) << 1;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa1_xor1 >> 0) & 0x01ull) << 2;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa2_xor1 >> 0) & 0x01ull) << 3;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa3_xor1 >> 0) & 0x01ull) << 4;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa4_xor1 >> 0) & 0x01ull) << 5;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa5_xor1 >> 0) & 0x01ull) << 6;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa6_xor1 >> 0) & 0x01ull) << 7;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa7_xor1 >> 0) & 0x01ull) << 8;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa8_xor1 >> 0) & 0x01ull) << 9;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa9_xor1 >> 0) & 0x01ull) << 10;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa10_xor1 >> 0) & 0x01ull) << 11;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa11_xor1 >> 0) & 0x01ull) << 12;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa12_xor1 >> 0) & 0x01ull) << 13;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa13_xor1 >> 0) & 0x01ull) << 14;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa14_xor1 >> 0) & 0x01ull) << 15;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa15_xor1 >> 0) & 0x01ull) << 16;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa16_xor1 >> 0) & 0x01ull) << 17;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa17_xor1 >> 0) & 0x01ull) << 18;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa18_xor1 >> 0) & 0x01ull) << 19;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa19_xor1 >> 0) & 0x01ull) << 20;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa20_xor1 >> 0) & 0x01ull) << 21;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa21_xor1 >> 0) & 0x01ull) << 22;
|
|
u_wallace_rca12_out |= ((u_wallace_rca12_u_rca22_fa21_or0 >> 0) & 0x01ull) << 23;
|
|
return u_wallace_rca12_out;
|
|
} |